Real-Time Interactivity: Crafting a Chat Application with Elixir, Phoenix, and WebSockets
Real-time applications are a vital component of the modern web. From multiplayer games to real-time notifications, live chat rooms, and collaborative online tools like Google Docs, real-time technology is everywhere. It’s for this reason that many companies choose to hire Elixir developers; their expertise can make all the difference in this ever-evolving landscape.
Various technologies make these applications possible, but in this article, we’ll focus on the combination of Elixir and WebSockets. Elixir, a functional, concurrent, general-purpose programming language that runs on the Erlang virtual machine (BEAM), is an excellent choice when hiring developers for building scalable and maintainable applications. Especially, those that require real-time features shine particularly well when Elixir is at play.
WebSockets, a communication protocol providing full-duplex communication channels over a single TCP connection, is an ideal companion to Elixir. Together, they can offer a robust and reliable foundation for real-time applications.
With the right team of Elixir developers, leveraging the power of these technologies can yield impressive results. Let’s dive in and see how these two can be used together to build powerful real-time applications.
1. An Introduction to Elixir and Phoenix
Elixir is designed for creating scalable and maintainable applications. It achieves this through Erlang’s features for building fault-tolerant systems, combined with a syntax that allows for easy readability and writing.
On top of Elixir, we have Phoenix, a web framework built for the Elixir programming language. One of the most powerful features of Phoenix is Channels that provide real-time communication. Channels in Phoenix use a WebSocket connection for client-server communication.
2. An Introduction to WebSockets
WebSockets provide a way for real-time bidirectional communication between the server and the client. In contrast to HTTP’s request/response model, WebSockets allow the server to push updates to the client as they become available. This is especially important for real-time applications where the state of the system can change rapidly and unpredictably.
3. Building a Chat Application with Elixir, Phoenix and WebSockets
Let’s build a simple real-time chat application using Elixir, Phoenix, and WebSockets. This will provide a concrete example of how to use these technologies together.
3.1. Create a new Phoenix application:
```bash mix phx.new chat_app ```
3.2. Create a new Channel:
Channels are the way Phoenix handles real-time features. Navigate to the `lib/chat_app_web/channels` directory and create a new file called `room_channel.ex`.
```elixir defmodule ChatAppWeb.RoomChannel do use Phoenix.Channel def join("room:lobby", _message, socket) do {:ok, socket} end end ```
This sets up a basic channel. When a client attempts to join the “room:lobby” topic, the `join` function will be called, and the client will be connected.
3.3. Update the Socket module:
Next, you need to update the `user_socket.ex` file found in the `lib/chat_app_web/channels` directory to include the new channel.
```elixir defmodule ChatAppWeb.UserSocket do use Phoenix.Socket channel("room:*", ChatAppWeb.RoomChannel) def connect(_params, socket, _connect_info) do {:ok, socket} end def id(_socket), do: nil end ```
This tells Phoenix to use the `RoomChannel` module when a WebSocket connection is made with the topic “room:*”.
3.4. Handle Incoming Messages:
Now, we’ll add a `handle_in/3` function to our `RoomChannel` to broadcast any new message that comes in.
```elixir defmodule ChatAppWeb.RoomChannel do use Phoenix.Channel def join("room:lobby", _message, socket) do {:ok, socket} end def handle_in("new_msg", %{ "body" => body }, socket) do broadcast(socket, "new_msg", %{ body: body }) {:noreply, socket} end end ```
Whenever a message with the event “new_msg” is received, the message is broadcasted to all subscribers.
3.5. Connect the Front-end:
On the front-end, you’ll use JavaScript to open a WebSocket connection to your server and join the room topic. You’ll also listen for the “new_msg” event and add new messages to the chat as they come in.
```javascript let socket = new Phoenix.Socket("/socket", {}) socket.connect() let channel = socket.channel("room:lobby", {}) channel.join() .receive("ok", resp => { console.log("Joined successfully", resp) }) .receive("error", resp => { console.log("Unable to join", resp) }) channel.on("new_msg", payload => { console.log(payload.body) }) ```
This is a basic real-time chat app and serves as a great starting point to understand the fundamentals of Elixir, Phoenix, and WebSockets. Of course, in a real-world scenario, things get more complex. You’d want to authenticate users, handle different rooms, persist messages to a database, and more. These are areas where the expertise of those who hire Elixir developers shines.
However, even with the simplified version we’ve created, this example should give you a sense of how you can use Elixir, Phoenix, and WebSockets to build a real-time application. And if the task seems daunting, remember that you can always hire Elixir developers who specialize in this area to ensure a robust and effective solution.
Conclusion
Building real-time applications with Elixir, Phoenix and WebSockets is a streamlined process, which is why many businesses opt to hire Elixir developers. Thanks to the functionality that Elixir and Phoenix provide, specifically through Channels, it enables easy real-time communication between the client and the server.
With Elixir’s strong concurrency model, applications can handle a large number of users and messages at the same time. This makes Elixir an excellent choice for real-time applications and a strong reason to hire Elixir developers when building such applications.
Remember, the application we built in this article is basic and there’s a lot more to explore and learn. Perhaps you might even consider hiring Elixir developers to delve deeper into features like user authentication, different chat rooms, message persistence, and more! With the right team of Elixir professionals, you can take your real-time application to new heights.
This is just the beginning of what you can do with Elixir, Phoenix, and WebSockets. The possibilities are endless!
Table of Contents
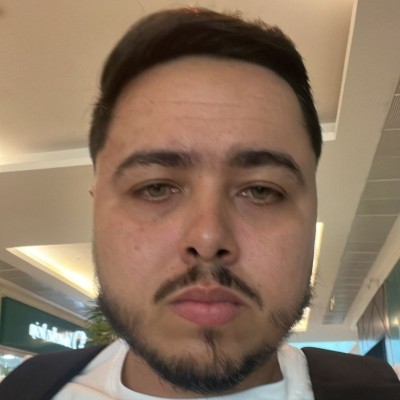
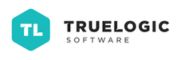