Building Elixir CLI Applications with ExCLI
Elixir is renowned for its scalability and fault-tolerance, but it’s also well-suited for building powerful command-line interface (CLI) applications. The ExCLI library simplifies the process of creating CLI tools with Elixir, providing a clean and intuitive API for command parsing and execution. This blog explores how to build effective CLI applications using Elixir and ExCLI, offering practical examples and insights into leveraging this powerful library.
Understanding ExCLI
ExCLI is a library designed to facilitate the creation of CLI applications in Elixir. It provides functionality for defining commands, parsing arguments, and handling user input in a way that’s straightforward and maintainable.
Using Elixir and ExCLI for CLI Development
Elixir’s expressive syntax and concurrency model, combined with ExCLI’s features, make it an excellent choice for developing CLI tools. Below are some key aspects and code examples demonstrating how to build CLI applications with Elixir and ExCLI.
1. Setting Up Your Project
To get started with ExCLI, you first need to add it to your Elixir project. Add `ex_cli` to your list of dependencies in `mix.exs`:
```elixir defp deps do [ {:ex_cli, "~> 0.1.0"} ] end ```
Then, run `mix deps.get` to install the dependency.
2. Defining Commands and Options
ExCLI allows you to define commands and their associated options easily. Below is an example of how to create a simple CLI application that supports a command with options.
Example: Creating a Command with Options
```elixir defmodule MyCLI do use ExCLI def run(args) do args |> parse_options() |> execute_command() end defp parse_options(args) do ExCLI.parse_options(args, [ option("--greet", type: :string, default: "World", description: "Name to greet") ]) end defp execute_command(%{greet: name}) do IO.puts("Hello, {name}!") end end ```
To run this CLI tool, use:
```sh mix run -e "MyCLI.run(System.argv())" ```
You can pass the `–greet` option to customize the greeting.
3. Handling Command-Line Arguments
Processing and validating command-line arguments is crucial for building effective CLI tools. ExCLI provides mechanisms to handle various types of arguments and validate user input.
Example: Parsing and Validating Arguments
```elixir defmodule MyCLI do use ExCLI def run(args) do case parse_arguments(args) do {:ok, options} -> process_options(options) {:error, message} -> IO.puts("Error: {message}") end end defp parse_arguments(args) do case ExCLI.parse_options(args, [ option("--file", type: :string, required: true, description: "Path to the file") ]) do {:ok, options} -> {:ok, options} {:error, _} -> {:error, "Missing required option --file"} end end defp process_options(%{file: file_path}) do IO.puts("Processing file: {file_path}") Add file processing logic here end end ```
In this example, the `–file` option is required, and the CLI will output an error if it is missing.
4. Providing Help and Documentation
A well-designed CLI tool should offer users help and documentation. ExCLI makes it easy to provide usage information and descriptions for your commands.
Example: Adding Help and Descriptions
```elixir defmodule MyCLI do use ExCLI def run(args) do args |> parse_options() |> handle_command() end defp parse_options(args) do ExCLI.parse_options(args, [ option("--version", type: :boolean, description: "Show version"), option("--help", type: :boolean, description: "Show help") ]) end defp handle_command(%{version: true}) do IO.puts("MyCLI version 1.0.0") end defp handle_command(%{help: true}) do IO.puts("Usage: my_cli [options]") IO.puts("--version Show version") IO.puts("--help Show help") end defp handle_command(_) do IO.puts("Run with --help for usage information.") end end ```
In this example, the CLI tool can display version information or help text based on the user’s input.
Conclusion
Elixir, combined with the ExCLI library, offers a powerful platform for building command-line interface applications. From setting up projects and defining commands to handling arguments and providing help, ExCLI simplifies the process of creating robust CLI tools. By leveraging these capabilities, developers can build effective and user-friendly CLI applications that enhance productivity and streamline tasks.
Further Reading:
Table of Contents
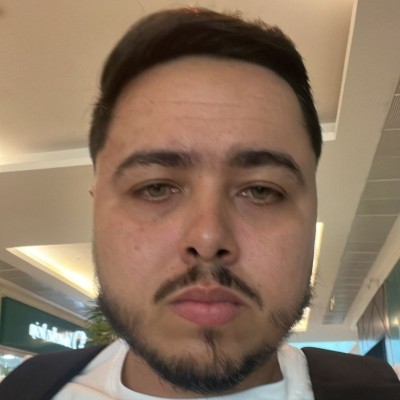
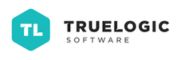