Exploring Concurrency in Elixir: A Deep Dive into Processes, Actors, Tasks, and Agents
In a world where software is rapidly evolving, concurrent programming has become an essential skill for every developer. This is why hiring Elixir developers, who are adept in handling such requirements, can be a game-changer for your software development process. Concurrency allows programs to perform multiple computations simultaneously, which can dramatically improve the efficiency of your software. Elixir, a dynamic and functional language designed for building scalable and maintainable applications, excels in this realm, making Elixir developers a sought-after resource for businesses looking to build high-performance applications.
Elixir leverages the Erlang Virtual Machine (VM), known for running low-latency, distributed, and fault-tolerant systems. This VM allows Elixir to offer excellent support for concurrency through its ‘processes’ and ‘actors’ model.
In this blog post, we’ll take a deep dive into Elixir’s concurrency model, dissecting the concepts of ‘processes’ and ‘actors’, and understanding how these can be used to build robust, concurrent applications.
1. Elixir’s Processes
An important aspect to remember about Elixir is that the term ‘process’ does not refer to the traditional Operating System (OS) process. Instead, these are lightweight processes managed entirely within the Erlang VM. They are isolated from each other, run concurrently, communicate via message passing, and are not bound to any operating system threads.
Let’s understand this through an example:
```elixir defmodule MyModule do def my_process do IO.puts "Hello from PID: #{inspect(self())}" end end pid = spawn(MyModule, :my_process, []) ```
In this code snippet, we’ve defined a function `my_process` inside `MyModule`. We’ve then used the `spawn` function to create a new process that executes the `my_process` function. The `spawn` function returns a process ID (PID), which is unique to each process. If you run this code, you should see an output similar to “Hello from PID: #PID<0.159.0>”, where the exact PID will vary.
2. Actor Model
Elixir, via the Erlang VM, follows the Actor Model for concurrent computation. This model treats “actors” as the universal primitives of concurrent computation. In the context of Elixir, each process acts as an “actor”.
Actors can:
– Maintain a private state.
– Process messages from a queue one at a time.
– Create more actors.
– Send messages to other actors.
Actors communicate with each other through asynchronous message passing. They do not share state with other actors, leading to a model where data is immutable. This helps prevent common concurrency problems like race conditions.
Let’s build on our previous example and incorporate some aspects of the actor model:
```elixir defmodule MyActor do def loop do receive do {from, msg} -> IO.puts "Received: #{msg}" send(from, {:ok, "Message received: #{msg}"}) loop() end end end defmodule Main do def run do pid = spawn(MyActor, :loop, []) send(pid, {self(), "Hello, actor!"}) receive do {:ok, msg} -> IO.puts msg end end end Main.run ```
In this example, we have `MyActor` set up to receive messages in a loop. Each message is a tuple, where the first element is the PID of the sender, and the second element is the actual message. Once `MyActor` receives a message, it prints it and sends a confirmation back to the sender.
The `Main.run` function spawns `MyActor` and sends it a message. It then waits to receive the confirmation message from `MyActor`. If you run this code, you’ll see the sent and received messages printed out.
3. Understanding Concurrency in Elixir
Understanding concurrency in Elixir boils down to understanding how processes and actors work. This is one reason why companies look to hire Elixir developers, as they bring with them this essential knowledge. At its core, the idea is simple: Elixir processes, which act as actors, run independently and communicate with each other via message passing. Elixir developers understand this model inherently and are able to harness its power effectively. Moreover, Elixir also provides higher-level abstractions built on these processes, like Tasks and Agents, which make working with concurrency even easier. By opting to hire Elixir developers, you are ensuring that these advanced features are leveraged to the full extent in your software development process.
3.1 Tasks
Tasks in Elixir provide a straightforward way to spawn processes and handle their results. They are often used when you want to perform a computation and care about the result.
Let’s look at an example where we calculate the Fibonacci of a number concurrently:
```elixir defmodule Fibonacci do def fib(n) when n < 2, do: n def fib(n), do: fib(n-1) + fib(n-2) end Enum.each(1..20, fn n -> Task.async(fn -> {n, Fibonacci.fib(n)} end) end) |> Enum.map(&Task.await/1) ```
In this example, we’re starting a new task for each Fibonacci calculation with `Task.async`. The tasks are run concurrently, and then we wait for each of them to finish with `Task.await`. This allows us to efficiently calculate multiple Fibonacci numbers at once.
3.2 Agents
Agents are a way to maintain state in Elixir. They provide a simple abstraction around state that can be accessed from different processes.
Here’s an example of an agent:
```elixir defmodule Counter do def start_link(initial_value) do Agent.start_link(fn -> initial_value end, name: __MODULE__) end def value, do: Agent.get(__MODULE__, & &1) def increment, do: Agent.update(__MODULE__, &(&1 + 1)) end Counter.start_link(0) IO.puts Counter.value() # Outputs: 0 Counter.increment() IO.puts Counter.value() # Outputs: 1 ```
In this example, we’ve created a counter where the state (the current value of the counter) is stored in an agent. We can retrieve the state with `Agent.get` and update it with `Agent.update`.
Conclusion
Elixir’s concurrency model, with its lightweight processes and actor model, paves the way for creating highly concurrent, scalable, and fault-tolerant applications. These unique traits make it advantageous to hire Elixir developers for your project. While mastering these core concepts is crucial, the beauty of Elixir lies in its abstractions like Tasks and Agents. These features allow Elixir developers to write concurrent code that is not only efficient but also highly readable and maintainable. Hence, by choosing to hire Elixir developers, you equip your team with the necessary tools to adeptly tackle the concurrent challenges of today’s software landscape.
Table of Contents
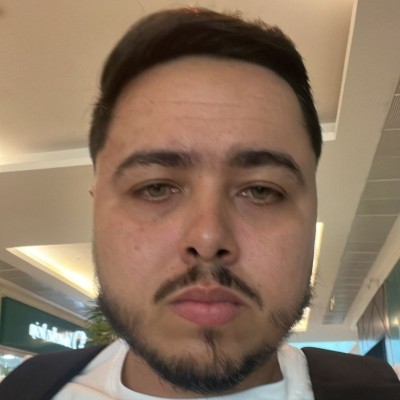
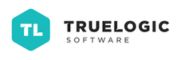