Exploring Elixir’s Concurrency Primitives: GenStage and Flow
Elixir is renowned for its concurrency and fault-tolerance features, making it an ideal choice for scalable and efficient data processing. Two of its core concurrency primitives, GenStage and Flow, offer advanced capabilities for managing and processing data in concurrent systems. This blog explores how to leverage GenStage and Flow in Elixir to build robust and performant applications.
Understanding Elixir’s Concurrency Primitives
Elixir’s concurrency primitives, GenStage and Flow, facilitate efficient data handling and processing. GenStage provides a mechanism for building concurrent stages in a pipeline, while Flow simplifies working with collections of data in a concurrent and distributed manner.
Using GenStage for Data Processing
GenStage is a library that allows you to create stages of data processing that can communicate with each other in a pipeline. It helps manage the flow of data and handle backpressure in concurrent systems.
1. Defining GenStage Producers and Consumers
In GenStage, you define producers and consumers to handle data flow. Here’s a simple example of a producer and a consumer using GenStage:
Example: Creating a Producer and Consumer
```elixir defmodule MyProducer do use GenStage def start_link(init_arg) do GenStage.start_link(__MODULE__, init_arg, name: :my_producer) end def init(_init_arg) do {:producer, 0} end def handle_demand(demand, state) do events = Enum.to_list(state..(state + demand - 1)) {:noreply, events, state + demand} end end defmodule MyConsumer do use GenStage def start_link(init_arg) do GenStage.start_link(__MODULE__, init_arg, name: :my_consumer) end def init(_init_arg) do {:consumer, []} end def handle_events(events, _from, state) do IO.inspect(events, label: "Received events") {:noreply, [], state} end end # Start the producer and consumer {:ok, _producer} = MyProducer.start_link([]) {:ok, _consumer} = MyConsumer.start_link([]) # Connect the producer and consumer GenStage.sync_subscribe(MyProducer, to: MyConsumer) ```
2. Building a Data Processing Pipeline with GenStage
You can create a data processing pipeline by chaining multiple stages. Each stage can be a producer, consumer, or a combination of both.
Example: Creating a Pipeline
```elixir defmodule MyPipeline do def start do {:ok, producer} = MyProducer.start_link([]) {:ok, processor} = MyProcessor.start_link([]) {:ok, consumer} = MyConsumer.start_link([]) GenStage.sync_subscribe(producer, to: processor) GenStage.sync_subscribe(processor, to: consumer) end end ```
Using Flow for Concurrent Data Processing
Flow is a library built on top of GenStage that simplifies concurrent data processing. It allows you to work with collections of data efficiently, making use of parallel processing.
1. Processing Collections with Flow
Flow provides a high-level API for processing collections of data in parallel. Here’s how to use Flow to process a list of numbers:
Example: Using Flow for Parallel Processing
```elixir defmodule MyFlow do def process do Flow.from_enumerable(1..1000) |> Flow.map(&(&1 2)) |> Flow.filter(&(&1 > 1000)) |> Enum.to_list() |> IO.inspect(label: "Processed Data") end end ```
2. Leveraging Flow for Distributed Processing
Flow can also be used for distributed data processing across multiple nodes. This feature is particularly useful for handling large-scale data.
Example: Using Flow in a Distributed Environment
```elixir defmodule MyDistributedFlow do def process do Flow.from_enumerable(1..1_000_000, max_demand: 10_000) |> Flow.partition(stages: 4, max_demand: 5_000) |> Flow.map(&(&1 2)) |> Flow.filter(&(&1 > 1_000_000)) |> Enum.to_list() |> IO.inspect(label: "Processed Data") end end ```
Conclusion
Elixir’s concurrency primitives, GenStage and Flow, offer powerful tools for managing and processing data efficiently. GenStage allows you to build complex data pipelines with backpressure handling, while Flow provides a high-level API for parallel and distributed data processing. By leveraging these tools, you can enhance the performance and scalability of your Elixir applications.
Further Reading:
Table of Contents
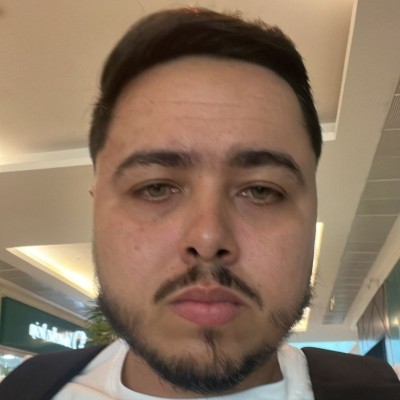
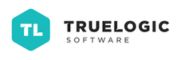