Concurrency Made Easy with Elixir’s GenServer
In the world of modern software development, the demand for highly responsive and scalable applications has led to a surge in the importance of concurrency. Concurrency, the ability of a system to handle multiple tasks simultaneously, can greatly enhance the performance and responsiveness of an application. However, with great power comes great complexity. Concurrency can be a double-edged sword, often introducing hard-to-debug issues like race conditions and deadlocks. This is where Elixir’s GenServer comes to the rescue, providing a robust and elegant solution to manage concurrency effortlessly. In this blog post, we’ll dive deep into the world of concurrency and explore how GenServer makes concurrent programming a breeze.
1. Understanding the Need for Concurrency
Before delving into the specifics of GenServer, let’s take a moment to understand why concurrency is so critical in today’s software landscape. Modern applications are expected to handle a multitude of tasks concurrently. Whether it’s serving numerous users simultaneously, processing incoming requests, or managing real-time data streams, concurrency is the backbone that keeps applications responsive and efficient. Without concurrency, an application might become sluggish, unresponsive, and unable to cope with the demands of a dynamic environment.
However, as we venture into the realm of concurrency, we must tread carefully. Traditional approaches to managing concurrency, such as using threads and locks, often lead to complex and error-prone code. This is where Elixir’s GenServer shines as a powerful alternative.
2. Introducing GenServer: Your Concurrency Companion
GenServer is a behavior in Elixir that provides a structured and reliable way to build concurrent, stateful processes. It abstracts away much of the complexity associated with managing concurrency, offering a clear and intuitive API to interact with concurrent processes. GenServer is a fundamental building block in the Erlang VM, upon which Elixir is built, making it a natural fit for building highly scalable and fault-tolerant systems.
2.1 The Anatomy of a GenServer
A GenServer module consists of callback functions that define its behavior. The most commonly used callback functions include:
1. init/1: This function is called when a new GenServer process is started. It initializes the process’s state and returns a tuple containing the initial state and optional extra data.
elixir defmodule MyServer do use GenServer def init(_args) do {:ok, %{}} end end
2. handle_cast/2: This function is used to handle asynchronous messages sent to the GenServer. It updates the state accordingly.
elixir defmodule MyServer do # ... def handle_cast({:update, data}, state) do {:noreply, Map.merge(state, data)} end end
3. handle_call/3: This function is used to handle synchronous requests. It can return a response and an updated state.
elixir defmodule MyServer do # ... def handle_call(:get_state, _from, state) do {:reply, state, state} end end
4. handle_info/2: This function handles miscellaneous messages and events.
elixir defmodule MyServer do # ... def handle_info({:timeout, _timer_ref}, state) do {:noreply, state} end end
2.2 Spawning a GenServer
To start a GenServer process, you use the GenServer.start/3 function, passing in the module name, initial arguments, and options.
elixir {:ok, pid} = GenServer.start(MyServer, initial_args, options)
2.3 Interacting with a GenServer
Once a GenServer process is running, you can interact with it using the GenServer.call/2 and GenServer.cast/2 functions.
- GenServer.call/2: This function sends a synchronous request to the GenServer, waiting for a response.
elixir response = GenServer.call(pid, :get_state)
- GenServer.cast/2: This function sends an asynchronous message to the GenServer.
elixir GenServer.cast(pid, {:update, %{key: value}})
3. Fault Tolerance and Supervision
One of the standout features of Elixir’s GenServer is its seamless integration with fault tolerance and supervision mechanisms. In Elixir, processes are isolated and can fail independently. GenServer leverages this isolation to create robust systems that can recover from failures without affecting other parts of the application.
3.1 The Supervisor-GenServer Duo
In Elixir, supervisors are responsible for monitoring and managing processes. A supervisor ensures that if a process terminates unexpectedly, it can be restarted or dealt with according to a predefined strategy. GenServers, being just processes, can be supervised like any other process.
3.2 Building a Supervision Tree
To create a supervised GenServer, you need to define a supervisor module that specifies the supervision strategy and the children to be supervised. Here’s a simplified example:
elixir defmodule MySupervisor do use Supervisor def start_link do Supervisor.start_link(__MODULE__, []) end def init(_) do children = [ {MyServer, initial_args} ] supervise(children, strategy: :one_for_one) end end
In this example, the supervisor is set up to use a one-for-one strategy, which means that if a child process (in this case, a GenServer) fails, only that specific process will be restarted.
Conclusion
Elixir’s GenServer offers a delightful way to tackle the complexities of concurrent programming. Its abstraction and clear separation of concerns simplify the creation of highly concurrent, responsive, and fault-tolerant systems. By providing a structured way to handle state and messages, GenServer enables developers to focus on the business logic rather than getting bogged down by the intricacies of concurrency management.
In this blog post, we’ve merely scratched the surface of what GenServer can offer. As you delve deeper into Elixir and GenServer, you’ll discover even more features and capabilities that empower you to build robust and efficient concurrent applications. So, embrace the power of Elixir and GenServer, and take your concurrent programming skills to the next level.
Whether you’re a seasoned Elixir developer or just embarking on your journey, GenServer is a tool that will undoubtedly become your go-to companion in conquering the challenges of concurrency. With its intuitive API, fault tolerance, and scalability, GenServer is here to simplify the world of concurrent programming. So, why wait? Dive into Elixir’s GenServer and unlock the true potential of concurrent programming today!
Table of Contents
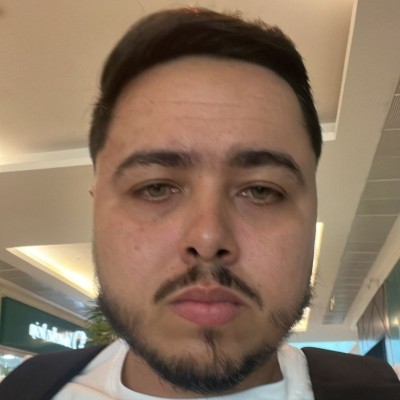
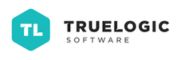