Exploring Elixir’s Task Module: Concurrent Asynchronous Programming
1. Exploring Elixir’s Task Module: Concurrent Asynchronous Programming
In the world of modern software development, concurrency is not just a nice-to-have feature; it’s a necessity. As applications become more complex and user demands increase, efficiently managing multiple tasks simultaneously becomes crucial. Elixir, with its robust concurrency model built on the Erlang Virtual Machine (BEAM), offers developers a powerful toolset to tackle concurrent programming challenges. One of the key components of Elixir’s concurrency toolbox is the Task module.
2. Understanding Elixir’s Task Module
The Task module in Elixir provides a convenient way to spawn and manage lightweight asynchronous processes. These processes run concurrently, allowing developers to execute multiple tasks simultaneously and leverage the full power of multicore systems. With tasks, developers can perform I/O operations, CPU-bound computations, and parallelize workloads with ease.
3. Getting Started with Tasks
To start leveraging the Task module in Elixir, let’s dive into some practical examples:
- Simple Task Spawning
```elixir task = Task.async(fn -> do_some_work() end) ```
This code asynchronously executes the `do_some_work()` function in a separate process, returning a task struct immediately. The task struct can be used to monitor the task’s progress or retrieve its result asynchronously.
- Handling Task Results
```elixir {:ok, result} = Task.await(task) ```
The `Task.await/1` function blocks the current process until the task completes, returning the result or raising an exception if the task fails. This enables seamless integration of asynchronous tasks into synchronous code.
- Error Handling
```elixir task = Task.async(fn -> potentially_failing_operation() end) case Task.await(task) do {:ok, result} -> handle_result(result) {:error, reason} -> handle_error(reason) end ```
Elixir’s Task module provides robust error handling mechanisms, allowing developers to gracefully handle failures and recover from errors in concurrent workflows.
4. Real-World Use Cases
Let’s explore some real-world scenarios where Elixir’s Task module shines:
4.1. Parallelizing HTTP Requests
When building web applications, it’s common to fetch data from multiple APIs simultaneously. With Elixir’s Task module, developers can easily parallelize HTTP requests, reducing overall response times and improving application performance. HTTPoison – https://hexdocs.pm/httpoison/HTTPoison.html is a popular HTTP client library in Elixir that seamlessly integrates with tasks, enabling concurrent HTTP requests out of the box.
4.2. Distributed Computing
Elixir’s concurrency model is inherently distributed, making it an excellent choice for building distributed systems and microservices. By leveraging tasks and Elixir’s built-in distribution mechanisms, developers can distribute workloads across multiple nodes in a cluster, achieving high availability and fault tolerance.
4.3. Background Job Processing
Task modules are well-suited for background job processing in web applications. Whether it’s sending emails, generating reports, or performing resource-intensive computations, tasks enable developers to offload work to separate processes, ensuring that the main application remains responsive and scalable.
Conclusion
Elixir’s Task module offers a powerful yet simple way to handle concurrent asynchronous programming, empowering developers to build highly responsive, scalable, and fault-tolerant systems. By mastering the Task module and embracing Elixir’s concurrency model, developers can unlock new possibilities in building robust distributed applications.
Ready to dive deeper into concurrent programming with Elixir? Check out the official Elixir documentation – https://elixir-lang.org/getting-started/tasks-and-processes.html for more insights and best practices.
Happy coding!
Table of Contents
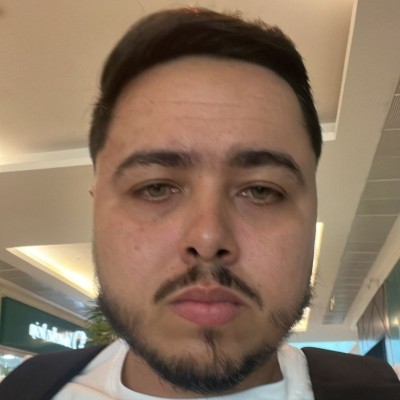
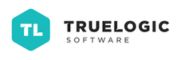