Creating GraphQL APIs with Elixir and Absinthe
GraphQL has gained significant popularity in recent years due to its flexibility, efficiency, and ease of use when it comes to building APIs. Elixir, known for its robustness and performance, is an excellent choice for developing scalable backend systems. When combined with Absinthe, a flexible and feature-rich GraphQL implementation for Elixir, you have a powerful stack for creating GraphQL APIs.
In this blog post, we’ll explore the benefits of using GraphQL, understand the basics of Elixir and Absinthe, and guide you through the process of creating a GraphQL API step by step. By the end of this tutorial, you’ll have a solid understanding of how to architect and implement GraphQL APIs using Elixir and Absinthe.
1. What is GraphQL?
GraphQL is a query language for your APIs, developed by Facebook in 2012 and later open-sourced in 2015. Unlike traditional REST APIs, where the client has limited control over the data returned, GraphQL empowers the client to request precisely the data it needs. This over-fetching and under-fetching problem in REST APIs is eliminated with GraphQL, resulting in more efficient and faster communication between clients and servers.
GraphQL enables a strong-typed schema definition that serves as a contract between the server and the client. Clients can request the specific fields they need, and the server responds with the requested data in a hierarchical structure, making it easier for clients to consume the data.
2. Why Choose Elixir and Absinthe for GraphQL APIs?
2.1 Elixir – The Language for Scalable Systems
Elixir is a functional, concurrent, and fault-tolerant language built on the Erlang Virtual Machine (BEAM). It inherits the battle-tested concurrency model from Erlang, making it an excellent choice for building distributed and fault-tolerant systems. Elixir’s lightweight processes, called “actors,” enable easy scalability and allow for handling a massive number of concurrent connections efficiently.
2.2 Absinthe – Elixir’s GraphQL Implementation
Absinthe is the most popular GraphQL implementation for Elixir. It provides a comprehensive set of tools and macros to define your GraphQL schema using Elixir’s powerful metaprogramming capabilities. Absinthe supports all the features of GraphQL, including queries, mutations, subscriptions, and directives. With clear and concise syntax, Absinthe allows you to build complex and efficient GraphQL APIs with ease.
3. Setting Up the Project
Before diving into the code, ensure you have Elixir and Mix (Elixir’s build tool) installed on your system. Create a new Elixir project using Mix:
bash mix new MyGraphQLAPI cd MyGraphQLAPI
Next, add Absinthe as a dependency in your mix.exs file:
elixir defp deps do [ {:absinthe, "~> 1.6"} # Add other dependencies here as needed ] end
Now, fetch and compile the dependencies:
bash mix deps.get mix deps.compile
4. Defining the Schema
In Absinthe, the schema is the heart of your GraphQL API. It defines the types, queries, and mutations that your API supports. Let’s start by creating a simple schema for a blog application.
Create a new file schema.ex in the lib folder and define the schema as follows:
elixir defmodule MyGraphQLAPI.Schema do use Absinthe.Schema # Define custom types here # Define queries and mutations here # Define the main query type query do # Add queries here end # Define the main mutation type mutation do # Add mutations here end end
In the above code snippet, we’re creating a new module MyGraphQLAPI.Schema and using the Absinthe.Schema macro to set up the necessary boilerplate for our schema.
5. Implementing Resolvers
Resolvers are functions that handle the fetching of data for each field in the schema. They are responsible for executing the logic and returning the data requested by the client.
Let’s define a simple resolver for fetching blog posts. Create a new module resolvers.ex in the lib folder:
elixir defmodule MyGraphQLAPI.Resolvers do # Import necessary modules and dependencies here # Define resolver functions here end
Now, let’s implement a resolver for fetching all blog posts:
elixir defmodule MyGraphQLAPI.Resolvers do # Import necessary modules and dependencies here alias MyGraphQLAPI.BlogPost def fetch_all_blog_posts(_, _) do {:ok, BlogPost.get_all()} end end
In this example, we assume that we have a module BlogPost that handles retrieving blog posts from the database. The resolver fetch_all_blog_posts/2 takes two arguments: the parent object (not used in this case) and the arguments passed in the GraphQL query (also not used here). The resolver simply calls the BlogPost.get_all/0 function and returns the result.
6. Handling Mutations
Mutations in GraphQL are used to modify data on the server-side. Let’s implement a mutation for creating a new blog post.
In the MyGraphQLAPI.Resolvers module, add the following code:
elixir defmodule MyGraphQLAPI.Resolvers do # Import necessary modules and dependencies here alias MyGraphQLAPI.BlogPost # ... (previous resolvers) def create_blog_post(_, %{title: title, content: content}) do case BlogPost.create(%{title: title, content: content}) do {:ok, post} -> {:ok, post} {:error, _} -> {:error, "Failed to create a blog post."} end end end
In the above code, we define a resolver create_blog_post/2 that takes the parent object (not used) and the arguments from the GraphQL mutation. The resolver calls BlogPost.create/1 with the provided data, and if the creation is successful, it returns the newly created blog post. Otherwise, it returns an error message.
7. Data Validation and Error Handling
Data validation is crucial in any API. Absinthe provides mechanisms to validate input data and handle errors gracefully.
Let’s add some validation to our create_blog_post/2 resolver:
elixir defmodule MyGraphQLAPI.Resolvers do # Import necessary modules and dependencies here alias MyGraphQLAPI.BlogPost # ... (previous resolvers) def create_blog_post(_, %{title: title, content: content}) do case validate_blog_post_data(title, content) do {:ok, validated_data} -> case BlogPost.create(validated_data) do {:ok, post} -> {:ok, post} {:error, _} -> {:error, "Failed to create a blog post."} end {:error, reason} -> {:error, reason} end end defp validate_blog_post_data(title, content) do case String.trim(title) do "" -> {:error, "Title cannot be empty."} _ -> {:ok, %{title: title, content: content}} end end end
In this example, we added a helper function validate_blog_post_data/2 to ensure that the provided title is not empty. If the data is valid, the function returns {:ok, validated_data}, otherwise, it returns {:error, reason}. The create_blog_post/2 resolver now validates the input before attempting to create a new blog post.
8. Performance Considerations
GraphQL APIs can be susceptible to performance issues if not carefully designed. Here are some performance considerations for your Elixir and Absinthe-based GraphQL APIs:
8.1 Caching
Caching can significantly improve the performance of your GraphQL API by reducing the number of database queries and expensive computations. Consider using a caching mechanism like Redis or ETS (Erlang Term Storage) to cache frequently requested data.
8.2 Batch Loading
Batch loading is a technique to optimize data fetching by combining multiple requests into a single query. This can be particularly useful when resolving associations or relationships between objects. Absinthe supports dataloaders, which can be used for efficient batch loading.
8.3 N+1 Query Problem
The N+1 query problem can occur when resolving a list of objects and fetching additional data for each item individually. This results in multiple database queries, leading to performance issues. Use dataloaders or other batch loading techniques to avoid the N+1 query problem.
9. Authentication and Authorization
Securing your GraphQL API is crucial to protect sensitive data and control access. Elixir and Absinthe offer several approaches to implement authentication and authorization:
9.1 Authentication
You can use middleware in Absinthe to implement authentication mechanisms like JWT (JSON Web Tokens) or OAuth. Middleware allows you to intercept and validate the incoming requests before they reach the resolver functions.
9.2 Authorization
For authorization, you can leverage Elixir’s built-in mechanism of pattern matching and guards. Define custom guards in your resolvers to restrict access to certain data based on user roles or permissions.
10. Testing the GraphQL API
Writing tests for your GraphQL API is essential to ensure its correctness and stability. Absinthe provides tools to help you write unit tests and integration tests for your schema, resolvers, and mutations.
Conclusion
In this blog post, we explored the benefits of using GraphQL and why Elixir with Absinthe is an excellent choice for building powerful GraphQL APIs. We learned how to set up an Elixir project, define a GraphQL schema, implement resolvers, handle mutations, and apply data validation and error handling. Additionally, we discussed performance considerations and touched on authentication and authorization.
With the knowledge gained from this tutorial, you are well-equipped to create flexible, scalable, and secure GraphQL APIs using Elixir and Absinthe. Happy coding!
Remember that this blog post only scratched the surface of what you can achieve with Elixir and Absinthe. Continue to explore the rich ecosystem and documentation to unleash the full potential of your GraphQL APIs.
Table of Contents
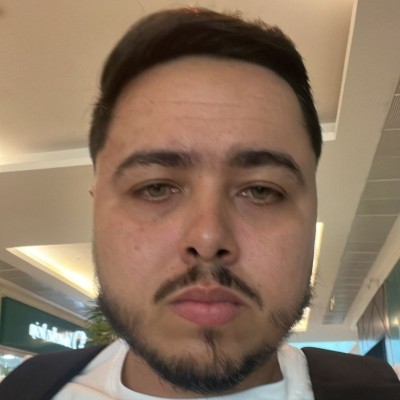
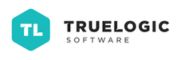