Debugging Elixir Applications: Tips and Techniques
Elixir, a powerful functional programming language built on the Erlang Virtual Machine (BEAM), has gained popularity for its scalability and fault-tolerant nature. However, like any other software, Elixir applications are not immune to bugs and errors. As developers, we must have a solid understanding of debugging techniques to identify and fix issues efficiently. In this blog, we’ll explore essential tips, techniques, and tools to help you debug Elixir applications effectively.
1. Print Debugging: A Timeless Classic
Print debugging, although considered old-fashioned, is still a reliable technique to gain insights into your code’s behavior. In Elixir, you can use the IO.inspect/2 function to print the values of variables at different points in your code.
elixir defmodule MyModule do def some_function(arg) do IO.inspect(arg, label: "Value of arg") # Rest of the code end end
When running your application or tests, you’ll see the labeled value printed to the console. This helps you understand the flow of your program and the values of variables at various stages.
2. Leveraging Elixir’s Logger
Elixir comes with a powerful built-in Logger module that allows you to log messages at different levels of severity. While print debugging clutters the code, using the Logger can provide the same information in a more organized manner. You can configure the Logger to write logs to a file, the console, or other destinations.
elixir # In your config/config.exs or config/dev.exs file config :logger, :console, format: "$time $metadata[$level] $message\n", level: :debug
In your code, you can use the Logger like this:
elixir defmodule MyModule do def some_function(arg) do Logger.debug("Value of arg: #{inspect(arg)}") # Rest of the code end End
By using the Logger, you can fine-tune the level of logging information, making it easier to track down issues without flooding the logs.
3. Debugger: IEx.pry
Elixir offers an interactive debugger through the IEx.pry function, which allows you to set breakpoints in your code and interact with the application during runtime. To use IEx.pry, you need to start your application with the IEx shell enabled:
bash iex -S mix
Then, place IEx.pry in your code where you want the breakpoint to occur:
elixir defmodule MyModule do def some_function(arg) do # Some code here IEx.pry # More code here end End
When the code execution reaches the IEx.pry statement, the application will stop, and you’ll get an interactive shell in the terminal where you can inspect and manipulate the application’s state.
Debugger commands:
- h or h <command>: Show help or help for a specific command.
- c: Continue execution until the next breakpoint.
- n: Execute the current function and stop at the first expression of the next function.
- s: Step into the function call, stopping at the first expression inside the function.
- r: Continue until the current function returns.
- respawn: Restart the current process.
- whereami: Show the current location in the code.
Remember to remove or comment out IEx.pry before deploying your application, as it will halt execution in production.
4. Taking Advantage of Mix Tasks
Elixir’s build tool, Mix, provides several built-in tasks that aid in debugging your applications. One of the most useful tasks is mix xref, which helps you identify unused functions, undefined functions, and other cross-reference related issues. Run the following command to analyze your project:
bash mix xref graph --format stats
This command generates a report highlighting any problematic areas in your application’s codebase.
5. Using :observer.start
The Erlang VM comes with a powerful tool called :observer.start, which gives you valuable insights into the inner workings of your Elixir application. You can start it in the IEx shell by running:
elixir :observer.start()
The Observer provides information about processes, memory usage, system statistics, and much more. It allows you to monitor your application in real-time and pinpoint potential bottlenecks or abnormal behavior.
6. Common Pitfalls and Anti-Patterns
While debugging, it’s crucial to be aware of common pitfalls and anti-patterns that may introduce bugs into your Elixir code. Some common issues to watch out for include:
6.1. Race Conditions
Race conditions occur when multiple processes or threads access and modify shared data simultaneously, leading to unexpected behavior. In Elixir, you can use tools like GenServer and Task to manage concurrency and avoid race conditions.
6.2. Process Leaks
Creating too many processes without properly managing their lifecycles can lead to resource exhaustion. Always monitor and supervise processes to prevent leaks.
6.3. Improper Error Handling
Ignoring or mishandling errors can result in undetected issues and potential crashes. Always implement comprehensive error handling to gracefully recover from errors and prevent application failures.
6.4. Bloated Supervision Trees
Supervision trees are vital for fault tolerance, but excessive layers or unnecessary processes in the supervision tree can make the application complex and difficult to debug.
Conclusion
Debugging Elixir applications effectively is essential for maintaining a robust and reliable codebase. In this blog, we explored various debugging techniques, including print debugging, using the Logger, leveraging IEx.pry, Mix tasks, :observer.start, and being aware of common pitfalls. Armed with these tips and techniques, you are better equipped to squash bugs and build stable Elixir applications.
Happy debugging!
Table of Contents
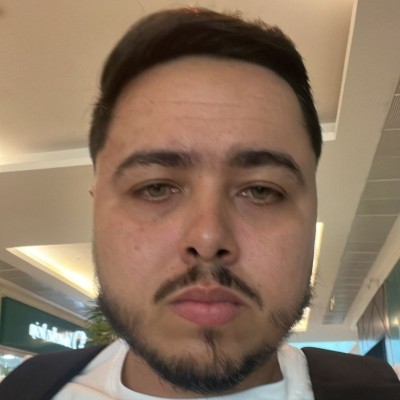
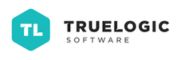