Elixir Q & A
How to define variables in Elixir?
In Elixir, defining variables is straightforward, and it follows a consistent syntax. Variables in Elixir are names bound to values, and they are used to store and reference data. Here’s how you can define variables in Elixir:
- Variable Naming Rules: Variables in Elixir must start with a lowercase letter and can contain letters, numbers, underscores, and the `@` symbol. They can’t start with a number or contain special characters like hyphens.
- Variable Assignment: To define a variable, you use the `=` operator, which is used for assignment in Elixir (not for equality testing). For example, to define a variable named `age` and assign it the value `30`, you would write `age = 30`.
- Immutability: It’s important to note that once you’ve assigned a value to a variable, you cannot change it. Elixir enforces immutability, which means that the value associated with a variable remains constant throughout its scope. If you need to modify data, you create a new variable with the updated value.
- Rebinding Variables: You can rebind variables within the same scope. For example, if you have `x = 5` and later write `x = 10`, you are reassigning `x` to a new value. The previous value of `x` is effectively shadowed within the current scope.
- Scope: Variables have scope within the block of code where they are defined. For instance, a variable defined within a function is only accessible within that function unless explicitly passed to another function or returned.
Here’s a simple example:
```elixir age = 30 name = "Alice" IO.puts("Hello, #{name}! You are #{age} years old.") ```
In this code, we’ve defined two variables, `age` and `name`, and used them to print a message. Understanding how to define and work with variables is fundamental to Elixir programming and forms the basis for manipulating data within your code.
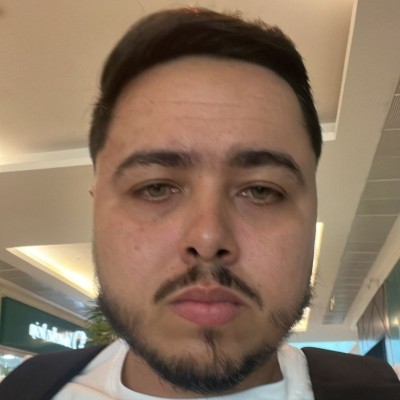
Previously at
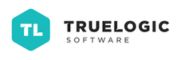
Tech Lead in Elixir with 3 years' experience. Passionate about Elixir/Phoenix and React Native. Full Stack Engineer, Event Organizer, Systems Analyst, Mobile Developer.