Building a Distributed Pub/Sub System with Elixir and Redis
Distributed pub/sub (publish/subscribe) systems are crucial for handling real-time messaging and event-driven architectures. Elixir, known for its concurrency and fault-tolerant features, combined with Redis, a powerful in-memory data structure store, provides a robust solution for building scalable and efficient pub/sub systems. This article explores how to construct a distributed pub/sub system using Elixir and Redis, highlighting practical examples and implementation strategies.
Understanding Pub/Sub Architecture
Pub/Sub is a messaging pattern where publishers send messages to channels, and subscribers receive messages from channels they are interested in. This decouples the message producers from the consumers, allowing for scalable and flexible communication. In a distributed environment, achieving efficient communication across multiple nodes becomes critical.
Using Elixir and Redis for Pub/Sub
Elixir is built on the Erlang VM, which provides excellent support for concurrency and distributed computing. Redis, with its built-in pub/sub support, complements Elixir’s strengths by offering a simple yet powerful messaging system. Below are the key components and code examples for building a pub/sub system with Elixir and Redis.
1. Setting Up Redis for Pub/Sub
Redis supports pub/sub out-of-the-box, making it straightforward to set up for messaging purposes. You can install Redis from its official website or use a package manager.
Example: Basic Pub/Sub Operations in Redis
To test Redis pub/sub capabilities, you can use the Redis CLI.
```bash # Open a terminal and run the Redis CLI redis-cli # Subscribe to a channel SUBSCRIBE my_channel # In another terminal, publish a message to the channel redis-cli PUBLISH my_channel "Hello, World!" ```
2. Connecting Elixir to Redis
Elixir can interact with Redis using libraries like `redix`. Add the `redix` dependency to your `mix.exs` file:
```elixir defp deps do [ {:redix, "~> 1.1"} ] end ```
Then, you can start a Redis connection and subscribe to channels.
Example: Connecting and Subscribing with Elixir
```elixir defmodule PubSubExample do use Redix, otp_app: :my_app def start_link do Redix.start_link("redis://localhost:6379") end def subscribe(channel) do Redix.command(:redix, ["SUBSCRIBE", channel]) end def handle_message({:message, channel, message}) do IO.puts("Received message on #{channel}: #{message}") end end ```
3. Publishing Messages in Elixir
To publish messages, you can use Redis commands from Elixir. Here’s how to publish a message to a Redis channel.
Example: Publishing Messages from Elixir
```elixir defmodule PubSubPublisher do use Redix, otp_app: :my_app def publish(channel, message) do Redix.command(:redix, ["PUBLISH", channel, message]) end end ```
4. Building a Distributed Pub/Sub System
Elixir’s distributed nature allows you to scale your pub/sub system across multiple nodes. You can use Elixir’s distributed features to connect nodes and share messages seamlessly.
Example: Setting Up Distributed Nodes
In your `mix.exs` file, configure your application to use distributed Elixir nodes:
```elixir defmodule MyApp.Application do use Application def start(_type, _args) do children = [ {PubSubExample, []} ] opts = [strategy: :one_for_one, name: MyApp.Supervisor] Supervisor.start_link(children, opts) end end ```
Run your application on different nodes:
```bash iex --sname node1 -S mix iex --sname node2 -S mix ```
5. Handling Fault Tolerance and Reliability
Elixir’s supervision tree can manage failures and ensure that your pub/sub system remains operational. Implementing supervisors and workers helps maintain system stability.
Example: Supervisor Setup
```elixir defmodule PubSubSupervisor do use Supervisor def start_link(_) do Supervisor.start_link(__MODULE__, :ok, name: __MODULE__) end def init(:ok) do children = [ {PubSubExample, []} ] Supervisor.init(children, strategy: :one_for_one) end end ```
Conclusion
Building a distributed pub/sub system with Elixir and Redis allows you to create scalable and efficient messaging solutions. Elixir’s concurrency and fault-tolerance capabilities, combined with Redis’s robust pub/sub features, offer a powerful toolkit for real-time communication. By leveraging these technologies, you can build a resilient and scalable pub/sub system that meets your messaging needs.
Further Reading:
Table of Contents
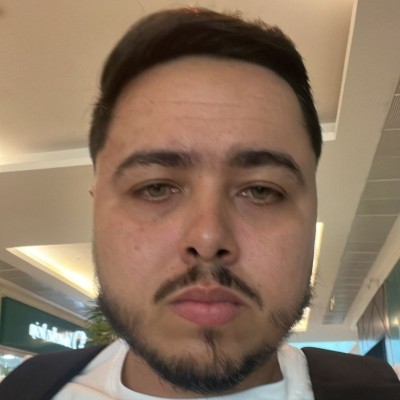
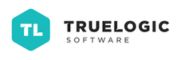