Exploring Elixir’s Ecto Associations: Relationships in Databases
Ecto is a powerful library for working with databases in Elixir, providing a robust way to handle database interactions, including complex relationships between entities. In this article, we’ll explore how to use Ecto’s association features to manage relationships in databases effectively. We’ll cover various types of associations and provide practical code examples to demonstrate how to implement them.
Understanding Ecto Associations
In relational databases, associations represent the relationships between different entities. Ecto, Elixir’s database wrapper and query generator, simplifies the process of defining and working with these relationships. Ecto supports several types of associations: `has_one`, `has_many`, and `belongs_to`. Understanding these associations is crucial for designing effective database schemas and querying related data.
1. Defining Associations
Ecto allows you to define relationships between schemas (i.e., database tables) using macros. These associations are used to represent different types of relationships such as one-to-one, one-to-many, and many-to-many.
Example: Defining a One-to-Many Association
Assume we have two schemas: `Author` and `Book`, where an author can have many books.
```elixir defmodule MyApp.Author do use Ecto.Schema import Ecto.Changeset schema "authors" do field :name, :string has_many :books, MyApp.Book timestamps() end def changeset(author, attrs) do author |> cast(attrs, [:name]) |> validate_required([:name]) end end defmodule MyApp.Book do use Ecto.Schema import Ecto.Changeset schema "books" do field :title, :string belongs_to :author, MyApp.Author timestamps() end def changeset(book, attrs) do book |> cast(attrs, [:title, :author_id]) |> validate_required([:title, :author_id]) end end ```
In this example, an `Author` schema has many `Book` schemas, and each `Book` belongs to an `Author`.
2. Querying Associated Data
Ecto allows you to easily query associated data using the `preload` function. This is useful when you want to fetch related records along with the main record.
Example: Preloading Associated Records
Here’s how to fetch an author along with all their books.
```elixir defmodule MyApp.AuthorQuery do import Ecto.Query, warn: false alias MyApp.Repo alias MyApp.Author def get_author_with_books(author_id) do Author |> Repo.get(author_id) |> Repo.preload(:books) end end ```
In this code, `Repo.preload(:books)` loads all books related to the author identified by `author_id`.
3. Managing Many-to-Many Relationships
Ecto also supports many-to-many relationships, where multiple records in one schema can be associated with multiple records in another schema. This is managed using a join table.
Example: Many-to-Many Relationship
Suppose we have a `Student` and a `Course`, where students can enroll in many courses, and each course can have many students.
```elixir defmodule MyApp.Student do use Ecto.Schema import Ecto.Changeset schema "students" do field :name, :string many_to_many :courses, MyApp.Course, join_through: "enrollments" timestamps() end def changeset(student, attrs) do student |> cast(attrs, [:name]) |> validate_required([:name]) end end defmodule MyApp.Course do use Ecto.Schema import Ecto.Changeset schema "courses" do field :title, :string many_to_many :students, MyApp.Student, join_through: "enrollments" timestamps() end def changeset(course, attrs) do course |> cast(attrs, [:title]) |> validate_required([:title]) end end ```
In this example, `Student` and `Course` schemas are linked through the `enrollments` join table.
4. Implementing Changesets with Associations
When updating or inserting data involving associations, you’ll need to handle changesets properly to ensure that the related data is correctly managed.
Example: Inserting a New Author with Books
Here’s how to insert an author with associated books.
```elixir defmodule MyApp.AuthorService do alias MyApp.{Author, Book, Repo} def create_author_with_books(attrs) do %Author{} |> Author.changeset(attrs) |> Ecto.Changeset.put_assoc(:books, attrs[:books]) |> Repo.insert() end end ```
In this code, `put_assoc(:books, attrs[:books])` associates the books with the new author before inserting them into the database.
Conclusion
Ecto’s association features provide a powerful way to manage relationships between entities in your database. By understanding how to define, query, and manage these associations, you can build robust and efficient data models in your Elixir applications. Leveraging these capabilities will enable you to handle complex relationships and ensure your database interactions are both effective and reliable.
Further Reading:
Table of Contents
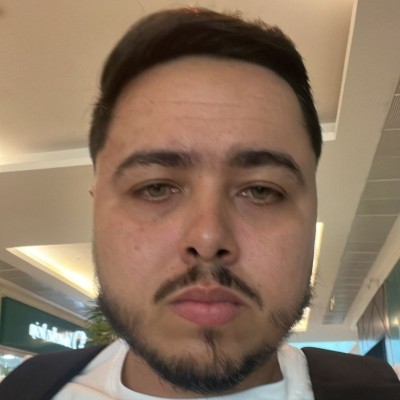
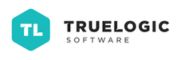