How does error handling work in Elixir?
Error handling in Elixir is a robust and fault-tolerant process designed to handle unexpected issues gracefully, ensuring the stability and reliability of Elixir applications. Elixir adopts a philosophy of “let it crash” and leverages processes and supervisors for managing errors effectively. Here’s how error handling works in Elixir:
- Processes and Isolation: Elixir runs concurrent code in lightweight processes, not to be confused with OS-level processes. These processes are isolated from each other, meaning that if one process encounters an error or crashes, it doesn’t affect other processes. This isolation allows for fault tolerance.
- Supervisors: Supervisors are special processes responsible for monitoring and managing other processes. When an error occurs within a supervised process, the supervisor can decide how to handle it. Supervisors can restart the failed process, terminate it, or take custom actions based on defined strategies.
- Process Linking: Processes in Elixir can be linked to each other. If a linked process crashes, it sends an exit signal to its linked processes. This allows for clean shutdowns and helps propagate errors to higher-level supervisors.
- Pattern Matching and Expected Failures: Elixir encourages pattern matching and returning tuples to indicate the outcome of functions. For example, a function might return `{:ok, result}` in case of success and `{:error, reason}` in case of failure. This convention makes it clear when and where errors can occur in your code.
- Exception Handling: While Elixir prefers “let it crash” and supervisors to manage errors, you can still use traditional exception handling with `try`, `catch`, and `rescue` blocks for exceptional cases where recovery or specific error handling is necessary.
- Logging and Monitoring: Elixir applications often include robust logging and monitoring mechanisms to track and analyze errors in real-time. This proactive approach allows developers to identify and resolve issues promptly.
Error handling in Elixir relies on processes, supervisors, and a fault-tolerant design philosophy to create resilient systems. By embracing failure as a natural part of software development and using supervision strategies, Elixir applications can gracefully recover from errors, ensuring high availability and system reliability.
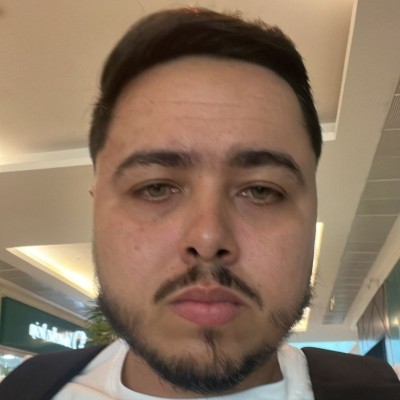
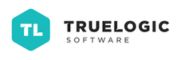