Introduction to Elixir’s ETS: In-Memory Key-Value Store
Elixir’s ETS (Erlang Term Storage) is a built-in, highly performant in-memory key-value store that offers flexible storage options for data. ETS is designed to provide quick access to data with minimal latency, making it an essential component for high-performance applications that require rapid data retrieval and manipulation.
Using ETS for Data Storage and Retrieval
ETS is well-suited for scenarios where data needs to be accessed frequently and quickly. Its robust features and integration with Elixir and Erlang applications make it a valuable tool for building scalable and efficient systems. Below are some key aspects and code examples demonstrating how to use ETS in Elixir.
1. Creating and Managing ETS Tables
ETS tables are the primary way to store and manage data in ETS. You can create different types of tables with various options to suit your needs.
Example: Creating and Inserting Data into an ETS Table
Here’s how to create a simple ETS table and insert data into it.
```elixir defmodule MyApp do def create_table do :ets.new(:my_table, [:set, :public, :named_table]) end def insert_data do :ets.insert(:my_table, {1, "Value 1"}) :ets.insert(:my_table, {2, "Value 2"}) end def fetch_data(key) do case :ets.lookup(:my_table, key) do [{^key, value}] -> {:ok, value} [] -> {:error, "Not found"} end end end # Usage MyApp.create_table() MyApp.insert_data() IO.inspect(MyApp.fetch_data(1)) # Output: {:ok, "Value 1"} ```
2. Querying and Updating Data
ETS provides efficient querying and updating mechanisms that can handle large volumes of data. You can perform various operations such as lookup, match, and delete.
Example: Querying and Updating Data
Here’s an example of querying and updating records in an ETS table.
```elixir defmodule MyApp do def update_data(key, new_value) do case :ets.lookup(:my_table, key) do [{^key, _old_value}] -> :ets.insert(:my_table, {key, new_value}) {:ok, "Updated successfully"} [] -> {:error, "Not found"} end end def delete_data(key) do :ets.delete(:my_table, key) end end # Usage MyApp.update_data(1, "Updated Value 1") IO.inspect(MyApp.fetch_data(1)) # Output: {:ok, "Updated Value 1"} MyApp.delete_data(1) IO.inspect(MyApp.fetch_data(1)) # Output: {:error, "Not found"} ```
3. Using ETS for Concurrent Access
ETS supports concurrent access, allowing multiple processes to read and write data simultaneously. This makes it ideal for applications with high concurrency requirements.
Example: Concurrent Access to ETS
Here’s an example of using ETS with concurrent processes.
```elixir defmodule ConcurrentApp do def start do :ets.new(:concurrent_table, [:set, :public, :named_table]) spawn(fn -> insert_data() end) spawn(fn -> insert_data() end) end defp insert_data do for i <- 1..10 do :ets.insert(:concurrent_table, {i, "Value #{i}"}) :timer.sleep(100) end end end # Usage ConcurrentApp.start() :timer.sleep(2000) IO.inspect(:ets.tab2list(:concurrent_table)) # Output: List of inserted records ```
4. Integrating ETS with Applications
ETS can be integrated into Elixir applications to manage state, cache data, or handle various data-related tasks. It is commonly used in conjunction with GenServers to maintain state across processes.
Example: Integrating ETS with a GenServer
Here’s how you might use ETS with a GenServer to manage application state.
```elixir defmodule MyGenServer do use GenServer def start_link(_) do GenServer.start_link(__MODULE__, [], name: __MODULE__) end def init(_) do :ets.new(:genserver_table, [:set, :public, :named_table]) {:ok, %{}} end def handle_call({:get, key}, _from, state) do value = case :ets.lookup(:genserver_table, key) do [{^key, value}] -> value [] -> nil end {:reply, value, state} end def handle_cast({:put, key, value}, state) do :ets.insert(:genserver_table, {key, value}) {:noreply, state} end end # Usage {:ok, _pid} = MyGenServer.start_link([]) GenServer.cast(MyGenServer, {:put, 1, "Value 1"}) IO.inspect(GenServer.call(MyGenServer, {:get, 1})) # Output: "Value 1" ```
Conclusion
Elixir’s ETS provides a versatile and high-performance solution for in-memory data storage and access. With its ability to handle large datasets, support for concurrent operations, and integration with Elixir applications, ETS is a powerful tool for developers building scalable and efficient systems. Leveraging ETS effectively can significantly enhance the performance and reliability of your Elixir applications.
Further Reading:
Table of Contents
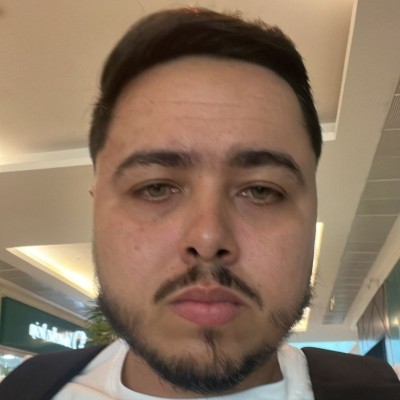
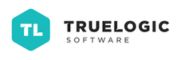