Unlocking the Power of Functional Programming: An In-Depth Look at Elixir
If you are seeking a functional programming language that offers concurrency, fault tolerance, and scalability, you should consider Elixir. A dynamic, functional language designed for building scalable and maintainable applications, Elixir is a popular choice among organizations looking to hire Elixir developers. Elixir leverages the Erlang VM, which is known for running low-latency, distributed, and fault-tolerant systems.
Elixir’s features align perfectly with today’s software requirements, especially in the area of IoT (Internet of Things) and building real-time applications. For businesses looking to capitalize on these trends, choosing to hire Elixir developers can lead to robust and efficient software solutions. This blog post aims to provide a comprehensive overview of functional programming with Elixir, underscoring why you might want to consider hiring Elixir developers for your next project.
1. Understanding Functional Programming
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions, which avoids changing-state and mutable data. It emphasizes the application of functions, in contrast with the procedural programming style, which emphasizes changes in state.
Elixir, being a functional language, provides excellent support for handling lists, tuples, recursion, higher-order functions, and pattern matching. Let’s delve into these concepts:
1.1 Lists and Tuples
A list in Elixir is a collection of elements enclosed within square brackets and separated by commas. For example:
```elixir list = [1, 2, 3, 4, 5] ```
You can perform various operations on lists such as adding elements, removing elements, and so on.
A tuple in Elixir is similar to a list but enclosed within curly brackets, like:
```elixir tuple = {1, 2, 3, 4, 5} ```
Tuples are often used to return additional information from a function, besides its main result.
1.2 Recursion
In Elixir, loops are achieved through recursion. Recursive functions are functions that call themselves to achieve a result. Here’s an example of a recursive function that computes the factorial of a number:
```elixir defmodule Math do def factorial(0), do: 1 def factorial(n), do: n * factorial(n-1) end ```
In the code above, we have two function clauses. The first clause (`factorial(0)`) is the base case, and the second clause is the recursive case, which calls itself until the base case is reached.
1.3 Higher-Order Functions
Higher-order functions are a key concept in functional programming. They can take one or more functions as arguments or return a function as a result. Elixir, being a functional language, naturally supports higher-order functions.
For instance, the `map` function is a common higher-order function that applies a given function to each item of a list:
```elixir list = [1, 2, 3, 4, 5] Enum.map(list, fn x -> x * 2 end) # Output: [2, 4, 6, 8, 10] ```
In the code above, `Enum.map` is the higher-order function that takes a list and a function as arguments. The function `fn x -> x * 2 end` is applied to each item of the list.
1.4 Pattern Matching
Pattern matching is another powerful feature in Elixir that allows a pattern to be matched against data structures. Here’s a basic example:
```elixir {a, b, c} = {:hello, "world", 42} # a would be :hello, b would be "world", and c would be 42 ```
Pattern matching is extensively used in function definitions, control structures, and assignments.
2. Elixir’s Benefits in Functional Programming
Elixir holds several benefits for functional programming, including the following:
2.1 Immutable Data Structures
In Elixir, all values are immutable. This means once a variable is bound to a value, it cannot be changed. This is key in functional programming as it prevents side effects.
2.2 Concurrency
Elixir provides excellent support for concurrency. It allows thousands of lightweight processes to be run simultaneously. This makes it ideal for real-time systems and high-availability systems.
2.3 Tooling
Elixir comes with fantastic tools like Mix for managing projects, Hex for package management, and ExUnit for testing. This enhances productivity and makes the development process smoother.
2.4 Scalability
One of Elixir’s standout features is its scalability. Given that it runs on the Erlang virtual machine, it can handle very large numbers of simultaneous connections. This feature makes it ideal for applications like web servers and databases that need to be highly responsive to many users at once.
2.5 Fault Tolerance
Elixir also has built-in mechanisms for creating self-healing systems. It uses the “let it crash” philosophy, where individual processes are allowed to fail without bringing down the whole system. When a process crashes, a supervisor process is notified and can take corrective action, such as restarting the failed process. This makes Elixir an excellent choice for building resilient, always-on applications.
2.6 Interoperability
Elixir is not a siloed language. It was developed to work harmoniously with Erlang. This means you can call Erlang functions directly from Elixir, offering great interoperability. Furthermore, Elixir can interface with other languages via the NIF (Native Implemented Functions) feature. This is beneficial in scenarios where a portion of your application requires heavy computations and could benefit from being written in a lower-level language such as C or Rust.
2.7 Metaprogramming
Elixir’s metaprogramming capabilities are another powerful aspect. Elixir code compiles to an abstract syntax tree (AST), which can be manipulated programmatically. This opens the door for creating domain-specific languages or extending the language itself. Elixir’s own testing library, ExUnit, and its build tool, Mix, are both excellent examples of how metaprogramming can be used to build powerful and expressive tools.
2.8 Developer Experience
Elixir is not just powerful but also fun to work with. It features a clear syntax that is easy to read and write. This makes it an attractive choice for organizations looking to hire Elixir developers. Moreover, its tooling ecosystem, including the built-in Mix build tool and the IEx interactive shell, offers a seamless and enjoyable developer experience. The documentation is first-class, making it easier for hired Elixir developers to get up to speed with the language and its libraries. So, when you hire Elixir developers, you are investing in a team that can quickly adapt and contribute to your projects effectively.
Conclusion
Functional programming with Elixir opens up a new paradigm with robust, efficient, and concurrent systems. By opting to hire Elixir developers, you can simplify the coding process leveraging higher-order functions, pattern matching, recursion, and other functional features. With the support of Erlang’s virtual machine, these skilled developers can produce highly reliable and fault-tolerant applications. If you are looking to dive into functional programming or need to create an application that’s efficient and scalable, hiring Elixir developers could be your perfect solution.
Table of Contents
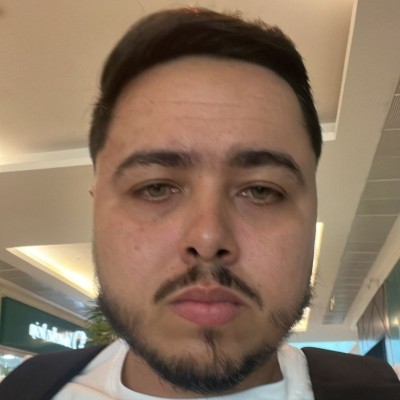
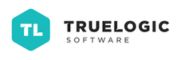