Understanding Elixir’s GenEvent Behavior for Event Handling
Event-driven programming is a fundamental concept in modern software development, enabling systems to respond to various asynchronous events efficiently. Elixir, a functional programming language built on the Erlang VM, offers a powerful tool for managing events through its GenEvent behavior. In this article, we’ll delve into Elixir’s GenEvent behavior, exploring its features, use cases, and providing code samples to help you grasp its capabilities effectively.
1. Understanding GenEvent Behavior
At its core, the GenEvent behavior is an event manager that facilitates event handling in Elixir applications. It provides a framework for defining, subscribing to, and emitting events within a system. GenEvent is part of the :gen_event module, which is one of the “OTP behaviors” that Elixir developers can utilize to build concurrent and fault-tolerant applications.
2. Features of GenEvent Behavior
GenEvent behavior offers several key features that make it a powerful tool for event handling:
- Decoupling Components: GenEvent allows you to decouple components within your application by providing a mechanism for communication without direct dependencies between them.
- Multiple Handlers: One of the unique features of GenEvent is its ability to support multiple event handlers. This means that different parts of your application can react to the same event in distinct ways.
- Dynamic Handler Management: Handlers can be added or removed dynamically at runtime, enabling flexibility in responding to changing requirements.
- Fallback Handlers: You can define a fallback handler that will be triggered if none of the registered handlers match an emitted event. This ensures that no event goes unnoticed or unhandled.
- Synchronous and Asynchronous Handling: GenEvent supports both synchronous and asynchronous event handling, allowing you to choose the appropriate approach based on the context of your application.
3. Use Cases of GenEvent Behavior
GenEvent behavior can be applied to various scenarios, enhancing the organization and efficiency of your Elixir applications:
- Logging and Monitoring: Use GenEvent to manage logging and monitoring events. Different handlers can capture and process logs for various purposes, such as debugging, analytics, or auditing.
- Notifications: Implement a notification system using GenEvent to notify users or other parts of the system when specific events occur, such as new data arrivals or critical errors.
- Plugin Systems: Build extensible applications with a plugin architecture. GenEvent can be used to manage events related to dynamically loaded plugins, allowing the main application to respond to plugin-specific events.
- State Management: Employ GenEvent to manage changes in state within your application. By emitting state change events, different components can stay synchronized and react accordingly.
- Distributed Systems: In distributed systems, GenEvent can help manage communication between nodes. Events emitted on one node can trigger reactions on other nodes, enabling coordination across a network of interconnected processes.
4. Using GenEvent: A Practical Example
Let’s dive into a practical example to better understand how to utilize GenEvent behavior in an Elixir application. Imagine a system that involves multiple sensors reporting temperature data. We want to handle these temperature readings and trigger alerts if the temperature exceeds certain thresholds.
Step 1: Defining the Event Handler
First, let’s define an event handler module that will process temperature events:
elixir defmodule TemperatureHandler do use GenEvent def handle_event({:temperature, sensor_id, temperature}, state) do if temperature > 100 do IO.puts("Alert! High temperature detected at sensor #{sensor_id}.") end {:ok, state} end end
In this module, we’re using the GenEvent behavior with the use GenEvent macro. We define a handle_event/2 function that reacts to the {:temperature, sensor_id, temperature} event tuple. If the temperature exceeds 100, an alert message is printed.
Step 2: Creating the Event Manager
Next, let’s create an event manager that will oversee the temperature events:
elixir defmodule TemperatureEventManager do def start_link do GenEvent.start_link(__MODULE__, []) end def emit_temperature(sensor_id, temperature) do GenEvent.notify(__MODULE__, {:temperature, sensor_id, temperature}) end def handle_event({:temperature, _sensor_id, _temperature}, state) do IO.puts("Event received by TemperatureEventManager.") {:ok, state} end end
Here, we define the TemperatureEventManager module. The start_link/0 function initializes the event manager. The emit_temperature/2 function is used to emit temperature events. The handle_event/2 function is a fallback handler that acknowledges the receipt of events.
Step 3: Putting It All Together
Now, let’s see how we can use our event handling system:
elixir {:ok, _pid} = TemperatureEventManager.start_link() GenEvent.add_handler(TemperatureEventManager, TemperatureHandler, nil) TemperatureEventManager.emit_temperature("sensor1", 98) TemperatureEventManager.emit_temperature("sensor2", 105)
In this example, we start the TemperatureEventManager, add the TemperatureHandler as an event handler, and then emit temperature events for two sensors. The handler will react to the high temperature event from “sensor2” and trigger an alert.
Conclusion
Elixir’s GenEvent behavior is a versatile tool for managing events in your applications. Its ability to decouple components, support multiple handlers, and provide dynamic handler management makes it a powerful choice for various use cases, from logging and notifications to state management and distributed systems. By following the principles and examples outlined in this article, you can harness the capabilities of GenEvent to build robust and responsive Elixir applications.
Table of Contents
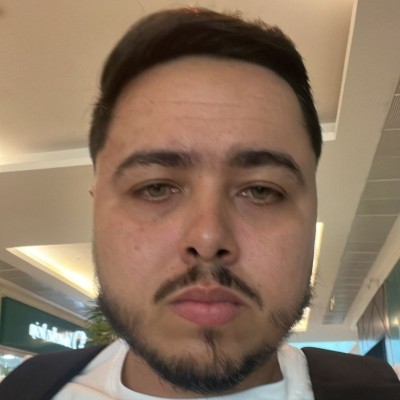
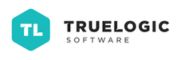