Introduction to Elixir’s GenFSM Behavior for Finite State Machines
In the realm of concurrent and distributed systems, managing state transitions efficiently is paramount. Elixir, with its Erlang heritage, offers robust tools for this purpose. One such tool is the GenFSM behavior, a foundational component for building Finite State Machines (FSMs) in Elixir. In this guide, we’ll explore the fundamentals of GenFSM and how it empowers developers to model complex stateful systems elegantly.
1. Understanding Finite State Machines (FSMs)
At its core, a Finite State Machine is a mathematical abstraction used to represent the behavior of systems with a finite number of states and transitions between them. FSMs find applications in various domains, including computer networking protocols, game development, and workflow management systems.
In Elixir, FSMs are implemented using the GenFSM behavior, which is part of the GenServer module in the OTP (Open Telecom Platform) framework. GenFSM provides a structured approach to define states, handle events, and manage state transitions in a concurrent and fault-tolerant manner.
2. Getting Started with GenFSM
To illustrate how GenFSM works, let’s consider a simple example of a traffic light controller. The controller can be in one of three states: “Green,” “Yellow,” or “Red.” Transitions occur based on predefined events such as “TimerExpired” or “PedestrianCrossing.”
```elixir defmodule TrafficLightController do use GenFSM def start_link do GenFSM.start_link(__MODULE__, :green, name: __MODULE__) end def init(:green) do {:ok, :green, :green} end def handle_event(:green, :timer_expired, :green) do {:next_state, :yellow, :timer.send_interval(3000, :timer_expired)} end # Define transitions for other states and events # State/Event handling functions for Yellow and Red states end ```
3. Key Concepts in GenFSM
- State Representation: Each state in a GenFSM module is represented as an Erlang term. State transitions are defined as functions that specify the next state and any side effects, such as sending messages or updating state variables.
- Event Handling: GenFSM allows developers to define event handlers for each state-event combination. These handlers dictate how the FSM reacts to incoming events and determine the subsequent state.
- Supervision and Fault Tolerance: GenFSM processes can be supervised like any other OTP process, ensuring fault tolerance and system reliability. If a GenFSM process crashes, its supervisor can restart it, maintaining the integrity of the system.
4. Real-World Applications
- Telecommunications: FSMs are commonly used in telecommunication systems for call setup, teardown, and call state management.
- Game Development: FSMs are integral to game AI, controlling character behavior, quest progress, and game state transitions.
- Workflow Management: Businesses leverage FSMs to model complex business processes, such as order processing and inventory management.
Conclusion
Elixir’s GenFSM behavior provides a powerful abstraction for building Finite State Machines, enabling developers to manage state transitions efficiently in concurrent, fault-tolerant systems. By understanding the core concepts and applying them to real-world scenarios, developers can unlock the full potential of GenFSM in their Elixir projects.
For further reading,
GenFSM documentation – https://hexdocs.pm/elixir/GenFSM.html
Elixir’s OTP framework – https://elixir-lang.org/getting-started/mix-otp/introduction-to-mix.html
Elixir’s concurrency model – https://elixir-lang.org/getting-started/concurrency.html
Table of Contents
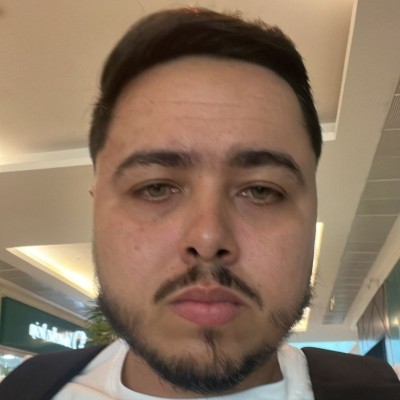
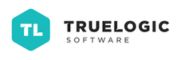