Building GraphQL APIs with Elixir and Sangria
In the world of modern web development, efficiency, scalability, and flexibility are paramount. As technology evolves, developers seek tools and frameworks that streamline processes while offering robust functionality. One such combination gaining traction in the development community is Elixir and Sangria for building GraphQL APIs. In this post, we’ll explore why this duo is a powerful choice and provide insights into leveraging their capabilities for crafting dynamic and efficient APIs.
1. Why Elixir and Sangria?
1.1 Elixir: The Language of Scalability and Reliability
Elixir, known for its concurrency, fault-tolerance, and scalability, runs on the Erlang VM, making it a top choice for building robust and highly performant applications. With its functional programming paradigm and lightweight processes, Elixir excels in handling concurrent operations, making it ideal for applications with high traffic and real-time requirements.
1.2 Sangria: The GraphQL Framework for Scala and Elixir
Sangria is a GraphQL implementation for Scala and Elixir, offering a type-safe and efficient way to build GraphQL APIs. Leveraging Elixir’s metaprogramming capabilities, Sangria provides a seamless integration with Elixir applications, allowing developers to define GraphQL schemas and resolvers with ease.
2. Getting Started: Setting Up Your Elixir Environment
Before diving into building GraphQL APIs with Elixir and Sangria, ensure you have Elixir installed on your system. You can follow the official installation guide – https://elixir-lang.org/install.html
Once installed, you’re ready to kickstart your Elixir project.
2. Integrating Sangria into Your Elixir Project
To incorporate Sangria into your Elixir project, you’ll need to add it as a dependency in your `mix.exs` file. Here’s an example:
```elixir defp deps do [ {:sangria, "~> 2.0"}, # Add other dependencies as needed ] end ```
After adding the dependency, fetch the package by running `mix deps.get`. With Sangria successfully integrated, you can now start defining your GraphQL schema.
3. Defining Your GraphQL Schema
In Sangria, defining a GraphQL schema involves specifying types, queries, mutations, and resolvers. Let’s create a simple schema for a blog application:
```elixir defmodule MyApp.Schema do use Sangria.Schema query do field :posts, list_of(:post) do resolve &MyApp.Resolvers.list_posts/2 end end object :post do field :id, :id field :title, :string field :content, :string end end ```
In this schema, we define a query to fetch a list of posts and specify the fields for the `post` type.
4. Implementing Resolvers
Resolvers are functions responsible for fetching data for GraphQL queries. Let’s implement a resolver to fetch posts:
```elixir defmodule MyApp.Resolvers do def list_posts(_args, _info) do # Logic to fetch posts from the database MyApp.Posts.list_posts() end end ```
5. Running Your GraphQL Server
With the schema and resolvers in place, it’s time to run your GraphQL server. You can use the `Absinthe` library, which provides tools for defining GraphQL schemas and handling HTTP requests. Here’s a basic setup:
```elixir defmodule MyApp.Web.Router do use MyApp.Web, :router pipeline :api do plug :accepts, ["json"] end scope "/api" do pipe_through :api forward "/graphql", Absinthe.Plug, schema: MyApp.Schema end end ```
Conclusion
Building GraphQL APIs with Elixir and Sangria offers a powerful combination for modern web development. With Elixir’s concurrency and fault-tolerance and Sangria’s type-safe GraphQL implementation, developers can create highly performant and flexible APIs to meet the demands of today’s applications.
If you’re interested in diving deeper into Elixir and Sangria, check out the official documentation:
– Elixir Documentation – https://hexdocs.pm/elixir/
– Sangria Documentation – https://sangria-graphql.github.io/sangria/
Start harnessing the power of Elixir and Sangria to supercharge your GraphQL API development today!
Table of Contents
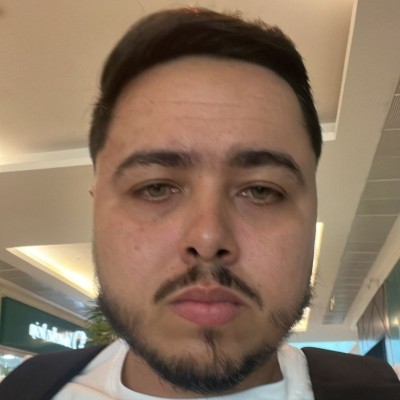
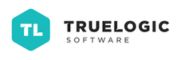