Handling Errors in Elixir: A Guide to Exception Handling
When it comes to writing robust and fault-tolerant software, error handling plays a crucial role. In the world of Elixir programming, a functional and concurrent language built on the Erlang VM, error handling takes on a unique approach. In this guide, we will delve into the art of handling errors in Elixir, exploring its powerful exception handling mechanisms, built-in features, and best practices that enable developers to write resilient code.
1. Introduction to Error Handling in Elixir
1.1 The Role of Error Handling
Error handling is an essential aspect of software development that ensures the graceful recovery from unexpected situations. In Elixir, error handling aligns with the language’s functional programming philosophy. Instead of relying solely on exceptions, Elixir encourages using pattern matching, supervision trees, and isolated processes to create resilient applications.
1.2 Functional and Concurrency Paradigm
Elixir’s functional programming principles make error handling elegant and predictable. Immutability and pure functions reduce the chances of unexpected side effects. Furthermore, Elixir’s concurrency model, based on lightweight processes (not OS threads), enables isolating code and preventing errors from propagating across the system.
2. Exception Handling Basics
2.1 try, catch, and rescue Blocks
Elixir provides the try, catch, and rescue constructs for handling exceptions. The try block wraps the code that might raise an exception, while the catch block handles exceptions raised within the try block. The rescue block is used to catch specific exceptions.
elixir try do File.read!("non_existent_file.txt") catch _ -> IO.puts("An error occurred") end
2.1 Raising Exceptions with raise Function
Developers can use the raise function to explicitly raise exceptions. This function takes an exception type and an optional message. This technique is useful for indicating exceptional conditions in code.
elixir defmodule Math do def divide(a, 0) do raise "Cannot divide by zero" end def divide(a, b) do a / b end End
3. Pattern Matching for Error Matching
3.1 Creating Custom Error Types
Elixir allows developers to define their own error types by creating modules that implement the Exception behavior. This approach is more structured than using plain atoms for error identification.
elixir defmodule MyApp.CustomError do defexception message: "A custom error occurred" end
3.2 Matching and Handling Specific Errors
Pattern matching shines in Elixir’s error handling strategy. By matching on specific errors, developers can craft precise error handling logic.
elixir try do MyApp.perform_operation() catch MyApp.CustomError -> IO.puts("Custom error occurred") RuntimeError -> IO.puts("Runtime error occurred") end
4. Built-in Error Handling Mechanisms
4.1 Processes and Isolates
Elixir’s concurrency model relies on isolated processes. If a process crashes, it doesn’t affect others. This isolation is key to building fault-tolerant systems.
elixir spawn(fn -> IO.puts("This is a separate process") File.read!("non_existent_file.txt") end)
4.2 Linking and Monitoring Processes
Processes can be linked to each other, which means if one process crashes, linked processes also receive a termination signal. Monitoring goes a step further, allowing processes to monitor others without being linked.
elixir pid = spawn(fn -> ... end) Process.link(pid) # Linking processes Process.monitor(pid) # Monitoring processes
5. Supervisor Strategies for Fault Tolerance
5.1 One-for-One Supervision
Supervisors are central to Elixir’s fault-tolerant design. In a one-for-one strategy, when a supervised process crashes, only that process is restarted.
elixir defmodule MyApp.Supervisor do use Supervisor def start_link do Supervisor.start_link(__MODULE__, []) end def init([]) do children = [ worker(MyApp.Worker, []) ] supervise(children, strategy: :one_for_one) end end
5.2 One-for-All Supervision
In a one-for-all strategy, if a process crashes, all supervised processes are restarted. This approach is suitable for scenarios where inter-process dependencies are critical.
elixir supervise(children, strategy: :one_for_all)
5.3 Dynamic Supervision
Elixir also supports dynamic supervision, where you can start and supervise processes dynamically during runtime, adapting to changing system conditions.
6. Best Practices for Resilient Code
6.1 Fail Fast and Explicit Error Handling
Following the principle of “fail fast,” Elixir developers emphasize handling errors as close to the source as possible. This prevents errors from cascading through the system.
6.2 Using Supervisors for Process Management
Leveraging supervisors is crucial for building fault-tolerant systems. They ensure processes are properly restarted or managed in case of failures.
6.3 Logging and Monitoring Errors
Implementing comprehensive logging and monitoring mechanisms helps developers identify and resolve issues before they impact users.
7. Beyond Exception Handling: Elixir’s Concurrency Model
7.1 Isolation and Message Passing
Elixir’s processes are lightweight and isolated, making it easy to build concurrent and parallel systems without the complexities of traditional threading models.
7.2 Managing State with Immutable Data
Elixir’s immutability ensures that processes can’t directly modify each other’s data, reducing the chances of errors caused by shared state.
Conclusion
Error handling in Elixir is a combination of well-structured exception handling, isolated processes, and robust supervision strategies. By embracing these features and best practices, developers can create resilient, fault-tolerant systems that gracefully recover from unexpected situations. Elixir’s unique approach to error handling reinforces its reputation as a language that excels in building reliable and concurrent applications.
Table of Contents
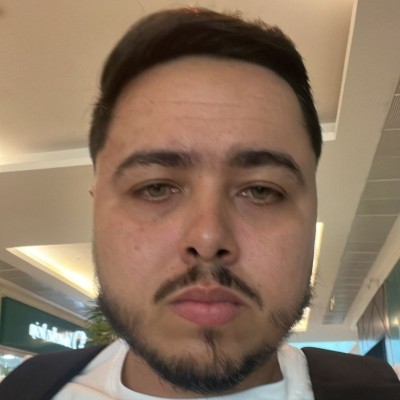
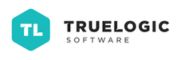