Using Elixir for High-Performance Computing
In the world of computing, performance is a critical factor that often drives technological advancements. High-performance computing (HPC) involves solving complex problems and executing tasks that demand extensive computational power in the shortest time possible. Traditionally, languages like C, C++, and Fortran have been preferred for HPC due to their low-level control over hardware resources. However, a new contender has emerged on the scene that challenges this status quo: Elixir. This functional programming language, built on the Erlang VM, brings a unique set of features that make it an excellent choice for high-performance computing tasks.
1. The Power of Elixir’s Concurrency Model
Concurrency lies at the heart of Elixir’s design philosophy. Elixir’s concurrency model is built around lightweight processes, also known as “actors,” which are much lighter than operating system threads. These processes communicate through message passing, allowing them to work independently and asynchronously. This model lends itself well to parallelism, a key requirement for high-performance computing.
In Elixir, creating a new process is as simple as spawning one using the spawn function:
elixir pid = spawn(fn -> # Your computation code here end)
This inherent support for lightweight processes makes it easy to distribute workloads across multiple cores and nodes, leveraging modern multi-core processors to their fullest potential. By embracing concurrency, Elixir enables seamless execution of tasks that can be parallelized, resulting in significant performance gains.
2. Fault Tolerance: A Game-Changer for HPC
High-performance computing systems often deal with massive datasets and complex calculations. With such complexity, failures are inevitable. Elixir, however, excels in building fault-tolerant systems. The Erlang VM upon which Elixir is built was designed for telecommunication systems, where reliability is paramount. It employs a “let it crash” philosophy, meaning that when a process encounters an unrecoverable error, it’s simply terminated and a new process is spawned in its place.
This approach may seem counterintuitive at first, but it greatly simplifies error handling and recovery. In an HPC context, this means that if a process encounters a fatal error, the system can quickly recover by restarting that specific process, rather than shutting down the entire application. This fault tolerance mechanism is essential for maintaining uninterrupted computations, especially in long-running simulations or calculations.
3. Scalability to Meet Growing Demands
One of the challenges in high-performance computing is adapting to varying workloads. Elixir’s architecture is designed for scalability from the ground up. The supervision tree pattern, a fundamental concept in Elixir, enables you to build systems that can automatically scale based on demand.
In an HPC scenario, this can be a game-changer. Imagine a scenario where incoming computational tasks increase suddenly due to a spike in demand. Elixir’s supervision tree can dynamically create and manage new processes to handle these tasks without manual intervention. This elasticity ensures that the system can scale up and down seamlessly, adapting to the required performance levels.
4. Leveraging Elixir’s Functional Paradigm
Elixir’s functional programming paradigm brings its own set of advantages to high-performance computing. Immutability is a core concept in functional programming, where data structures are not modified once created. This property simplifies concurrent programming, as there are no concerns about shared mutable state and potential race conditions.
Elixir’s pipelines and higher-order functions encourage a declarative coding style. This can lead to more readable and maintainable code, which is crucial in HPC where optimizing performance while ensuring correctness is paramount.
elixir data |> preprocess() |> calculate() |> postprocess()
The ease of composing functions in a pipeline allows for clear separation of concerns and facilitates parallelism without sacrificing code clarity.
5. Real-World Use Cases
Elixir’s applicability in high-performance computing is not just theoretical. Several real-world applications and projects have successfully harnessed its power:
5.1. Numerical Simulations
Elixir’s lightweight processes and efficient concurrency model make it a solid choice for running complex numerical simulations. Whether it’s simulating physical phenomena, financial models, or scientific experiments, Elixir’s capabilities can significantly reduce simulation times.
5.2. Data Analysis and Machine Learning
Elixir can be used to build scalable data processing pipelines for tasks like data cleansing, feature engineering, and model training. While it may not directly compete with languages like Python or R for machine learning libraries, it can still handle data preprocessing tasks efficiently in parallel.
5.3. Distributed Computing
Elixir’s built-in support for distributed systems makes it well-suited for tasks that require processing across multiple machines. MapReduce-style algorithms, distributed databases, and distributed rendering are areas where Elixir’s strengths shine.
6. Challenges and Considerations
While Elixir offers many advantages for high-performance computing, it’s important to consider the trade-offs and challenges:
- Learning Curve: Elixir’s syntax and concurrency model might be unfamiliar to developers coming from imperative or object-oriented backgrounds. However, the learning curve is often offset by the benefits it brings.
- Library Availability: While Elixir has a growing ecosystem of libraries, it might not have the extensive set of specialized libraries that languages like C++ or Python offer for specific HPC tasks.
- Performance Overhead: Although Elixir’s concurrency model is efficient, it introduces some overhead compared to languages that can interface more directly with low-level hardware resources.
Conclusion
Elixir’s unique combination of concurrency, fault tolerance, scalability, and functional programming principles makes it a compelling choice for high-performance computing. Its lightweight processes enable parallelism, fault tolerance ensures robustness, and scalability caters to changing workloads. While it may not replace traditional HPC languages in all scenarios, Elixir opens up new possibilities and solutions for demanding computational tasks. As the field of high-performance computing continues to evolve, Elixir stands ready as a powerful tool in the arsenal of developers and researchers pushing the boundaries of computation.
In a landscape where performance is paramount, Elixir emerges as a language that challenges conventions and offers a fresh perspective on how high-performance computing can be achieved. Whether it’s tackling complex simulations, processing vast datasets, or building distributed systems, Elixir’s strengths are poised to make a lasting impact on the future of computation.
Table of Contents
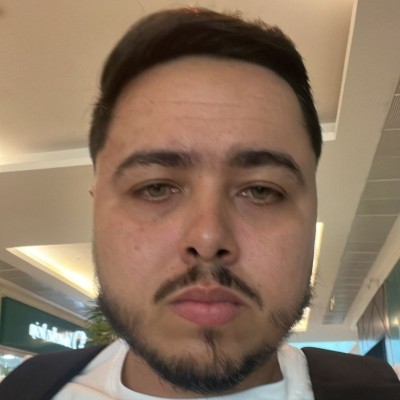
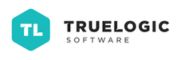