Understanding Elixir’s Immutability and Data Structures
In the world of programming, the quest for more efficient and reliable systems is ongoing. Developers are always in search of tools and languages that can help them achieve these goals. Elixir, a functional and concurrent programming language, has gained prominence for its unique approach to immutability and data structures. In this blog post, we’ll delve into the concepts of immutability and data structures in Elixir, understanding why they are crucial, and how they contribute to the language’s reliability and performance.
Table of Contents
1. The Essence of Immutability
1.1. What is Immutability?
Immutability is a core principle in functional programming, and it refers to the property of data that cannot be changed after creation. In simpler terms, once data is created, it remains constant and cannot be modified. This might seem counterintuitive at first, especially coming from an imperative programming background where mutable variables are the norm. However, immutability brings several advantages that contribute to the reliability and predictability of code.
In an immutable programming paradigm, instead of modifying existing data, new data is created based on the existing data. This ensures that the original data remains unchanged and can be safely shared across different parts of a program. This approach leads to fewer bugs related to unexpected side effects and race conditions, as the data is not altered concurrently by different parts of the program.
1.2. Immutability in Elixir
Elixir, as a functional programming language, fully embraces the concept of immutability. All data structures in Elixir are immutable by default. This means that once a data structure is created, its contents cannot be modified. Instead, any operation that seems like it would modify the structure actually returns a new copy of the structure with the desired changes.
Let’s look at an example to illustrate immutability in Elixir:
elixir list1 = [1, 2, 3] list2 = List.append(list1, 4) IO.inspect(list1) # Output: [1, 2, 3] IO.inspect(list2) # Output: [1, 2, 3, 4]
In this example, list1 remains unchanged even after appending an element to list2. This ensures that list1 is still intact and can be used elsewhere without any unexpected modifications.
2. Exploring Elixir Data Structures
Elixir provides a variety of data structures that leverage immutability to offer both efficiency and reliability in programming.
2.1. Lists
Lists in Elixir are ordered collections of elements. They are defined using square brackets and can hold any type of data. Lists are particularly efficient for prepending elements, but not as efficient for appending elements.
elixir fruits = ["apple", "banana", "orange"]
2.2. Tuples
Tuples are similar to lists but have a fixed number of elements and are enclosed in curly braces. They are often used when you need to group a small, fixed set of elements together.
elixir point = {5, 10}
2.3. Maps
Maps are key-value data structures that allow any data type to be used as keys or values. They provide fast look-up times and are commonly used for storing and retrieving data.
elixir user = %{name: "Alice", age: 30}
2.4. Keyword Lists
Keyword lists are lists of key-value pairs. They are used when the same key needs to appear multiple times in a collection.
elixir options = [{:color, "red"}, {:size, "medium"}]
2.5. Structs
Structs are similar to maps, but they are defined using a module and provide compile-time checks for the structure of the data. They are often used to represent more complex data types.
elixir defmodule Person do defstruct name: "", age: 0 end person = %Person{name: "Bob", age: 25}
3. Benefits of Immutability and Elixir Data Structures
3.1. Reliable Parallelism
One of the significant advantages of immutability is that it makes parallel programming significantly easier. Since data cannot be changed once created, multiple processes or threads can operate on the same data without the fear of unexpected modifications. This makes it easier to write concurrent and parallel code, a feature that Elixir takes full advantage of due to its actor-based concurrency model.
3.2. Enhanced Robustness
Immutability contributes to code robustness by minimizing the chances of bugs caused by unintended side effects. In languages with mutable data, changes made to a data structure can propagate unexpectedly, causing bugs that are challenging to trace. In contrast, in Elixir, the immutability of data structures ensures that once data is created, it remains consistent throughout its lifetime.
3.3. Efficient Memory Usage
Immutable data structures can also lead to more efficient memory usage. Since data is not modified in place, new versions of the data can share memory with the original data, reducing the need for copying large amounts of data. This approach is known as structural sharing and is a key factor in making immutable data structures memory-efficient.
4. Embracing the Functional Paradigm
Elixir’s emphasis on immutability and functional programming aligns with the broader functional programming paradigm. In functional programming, functions are treated as first-class citizens, and the focus is on writing pure functions that don’t have side effects. Elixir encourages this paradigm by providing powerful tools and abstractions that support functional programming techniques.
5. Case Study: Concurrent Data Processing
Let’s consider a simple case study to highlight the benefits of immutability and Elixir’s data structures in concurrent data processing.
Imagine a scenario where multiple users are submitting orders simultaneously to an e-commerce system. Each order includes the item, quantity, and the user’s details. To process these orders concurrently, we can represent each order as a map and store them in a collection. Thanks to immutability, different processes can operate on this collection without worrying about data corruption.
elixir defmodule OrderProcessor do def process_orders(orders) do Enum.each(orders, fn order -> # Process the order and update inventory # ... end) end end orders = [ %{item: "phone", quantity: 2, user: "user1"}, %{item: "laptop", quantity: 1, user: "user2"}, %{item: "headphones", quantity: 3, user: "user3"} ] OrderProcessor.process_orders(orders)
In this example, the process_orders/1 function processes each order concurrently, taking advantage of Elixir’s lightweight processes. The immutability of the order data ensures that each process can work independently without interfering with others, resulting in reliable and efficient concurrent data processing.
6. Tips for Effective Elixir Programming
- Embrace immutability: Leverage the power of immutability by designing your programs with a focus on creating new data rather than modifying existing data.
- Choose the right data structure: Select the appropriate data structure for your needs. Lists, tuples, maps, keyword lists, and structs each have their strengths and use cases.
- Master pattern matching: Elixir’s pattern matching capabilities are a cornerstone of functional programming. Utilize pattern matching to extract and manipulate data with ease.
- Understand concurrency: Study Elixir’s concurrency model, and use lightweight processes to design highly concurrent and fault-tolerant applications.
- Practice functional programming: Embrace the functional paradigm by writing pure functions and minimizing side effects. This leads to more predictable and maintainable code.
Conclusion
Immutability and data structures are at the heart of Elixir’s design philosophy. By emphasizing these principles, Elixir provides a robust, concurrent, and highly reliable environment for building applications. The immutability of data structures not only enhances parallelism but also leads to more predictable code that is less prone to bugs caused by unintended side effects. As you continue your journey with Elixir, understanding and harnessing the power of immutability and data structures will undoubtedly be instrumental in crafting efficient and resilient software systems.
Table of Contents
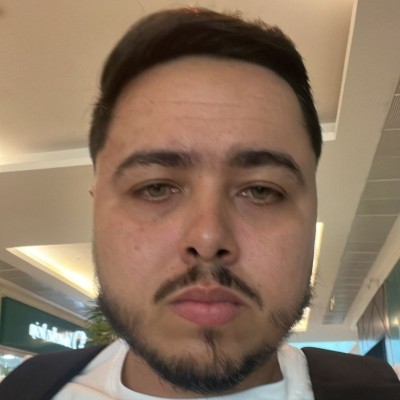
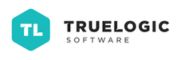