How to implement WebSockets in Phoenix?
Implementing WebSockets in Phoenix is a powerful way to add real-time features and interactivity to your web applications. Phoenix provides seamless support for WebSockets through its built-in PubSub system and the Phoenix Channels library. Here’s a step-by-step guide on how to implement WebSockets in Phoenix:
- Set Up a Phoenix Application:
Start by creating a Phoenix application or use an existing one. Ensure you have Phoenix installed and set up.
- Generate a Channel:
Use the `mix phx.gen.channel` command to generate a new channel. For example:
```bash mix phx.gen.channel ChatRoom ```
This will create a ChatRoom channel, along with associated files for handling WebSocket communication.
- Modify the Channel Module:
In the generated `chat_room_channel.ex` file (located in `lib/your_app_web/channels`), define the behavior of your WebSocket channel. You can specify event handlers, join behavior, and message handling logic in this module.
- Mount the Channel in the Router:
In your `lib/your_app_web/endpoint.ex` file, ensure that the Phoenix router is set up to mount the channel. Add the following line inside the `socket/1` function:
```elixir channel "room:chat", YourAppWeb.ChatRoomChannel ```
- JavaScript Client Code:
In your front-end JavaScript code, establish a WebSocket connection to the specified channel. Phoenix provides a JavaScript library that you can include in your assets. Import it, and then connect to the channel as follows:
```javascript import { Socket } from "phoenix"; let socket = new Socket("/socket", { params: { token: window.userToken } }); socket.connect(); let channel = socket.channel("room:chat", {}); channel.join() .receive("ok", (resp) => { console.log("Joined successfully", resp); }) .receive("error", (resp) => { console.log("Unable to join", resp); }); ```
- Message Broadcasting:
Use the `broadcast/3` function in your channel module to send messages to all connected clients. Clients can then handle incoming messages and update the user interface in real-time.
- Authentication (Optional):
If your WebSocket functionality requires authentication, you can use tokens or session data to identify and authorize users when they connect to the channel.
- Testing and Debugging:
Phoenix provides testing utilities for WebSocket channels. Write tests to ensure that your WebSocket functionality behaves as expected.
- Deployment:
Deploy your Phoenix application with WebSocket support to a hosting platform of your choice. Ensure that WebSocket connections are allowed through any proxy or firewall.
By following these steps, you can successfully implement WebSockets in Phoenix and enable real-time communication in your web applications. Phoenix Channels abstract many of the complexities of WebSocket communication, making it easier to build interactive and collaborative features in your Phoenix projects.
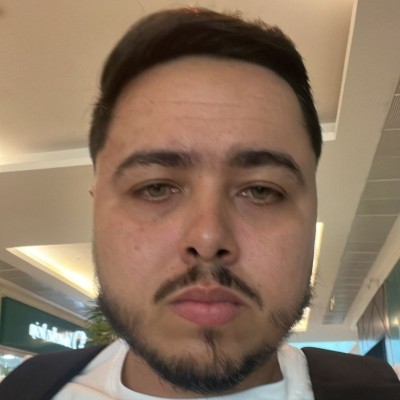
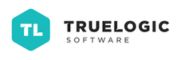