Exploring Elixir’s IO and File Modules: Input/Output Operations
Elixir, known for its concurrency and fault-tolerance, also provides robust tools for handling input and output operations through its IO and File modules. These modules offer powerful capabilities for managing file interactions, reading and writing data, and handling streams. This blog delves into Elixir’s IO and File modules, showcasing practical examples of input and output operations.
Understanding Elixir’s IO and File Modules
Elixir’s IO module is designed for handling input and output operations, including interaction with the console and file streams. The File module provides functions specifically for file manipulation, such as reading from and writing to files. Together, these modules enable efficient data handling and management in Elixir applications.
Using Elixir for File Operations
Elixir offers a straightforward approach to file operations with its File module. Below are key aspects and code examples illustrating how Elixir can be utilized for file management.
1. Reading and Writing Files
Reading from and writing to files are common tasks in many applications. Elixir’s File module simplifies these operations.
Example: Reading a File
To read the contents of a file, use the `File.read/1` function.
```elixir defmodule FileExample do def read_file(file_path) do case File.read(file_path) do {:ok, content} -> IO.puts("File content:\n#{content}") {:error, reason} -> IO.puts("Failed to read file: #{reason}") end end end FileExample.read_file("example.txt") ```
Example: Writing to a File
To write data to a file, use the `File.write/2` function.
```elixir defmodule FileExample do def write_file(file_path, content) do case File.write(file_path, content) do :ok -> IO.puts("File successfully written") {:error, reason} -> IO.puts("Failed to write file: #{reason}") end end end FileExample.write_file("output.txt", "Hello, Elixir!") ```
2. Handling File Streams
Elixir’s File module also supports streaming large files efficiently, allowing you to process data incrementally.
Example: Streaming a File
To stream a file, use the `File.stream!/1` function in conjunction with Enum operations.
```elixir defmodule FileStreamExample do def stream_file(file_path) do File.stream!(file_path) |> Enum.each(fn line -> IO.puts("Line: #{line}") end) end end FileStreamExample.stream_file("large_file.txt") ```
3. Managing File Attributes
Elixir provides functions for querying and managing file attributes, such as checking if a file exists or retrieving its size.
Example: Checking File Existence
To check if a file exists, use the `File.exists?/1` function.
```elixir defmodule FileAttributesExample do def check_file_exists(file_path) do if File.exists?(file_path) do IO.puts("File exists") else IO.puts("File does not exist") end end end FileAttributesExample.check_file_exists("example.txt") ```
Integrating IO and File Modules with Streams
Elixir’s IO module can be used to handle input and output operations in a more interactive manner, such as reading from the console or writing to standard output.
Example: Reading from Standard Input
To read user input from the console, use the `IO.gets/1` function.
```elixir defmodule IOExample do def read_input do IO.write("Enter some text: ") input = IO.gets("") |> String.trim() IO.puts("You entered: #{input}") end end IOExample.read_input() ```
Example: Writing to Standard Output
To write data to standard output, use the `IO.puts/1` function.
```elixir defmodule IOExample do def print_message(message) do IO.puts("Message: #{message}") end end IOExample.print_message("Hello, Elixir World!") ```
Conclusion
Elixir’s IO and File modules offer powerful tools for handling input and output operations efficiently. Whether you’re reading from or writing to files, streaming data, or managing file attributes, these modules provide the necessary functionality for effective data management in your Elixir applications. By leveraging these capabilities, you can streamline your file operations and enhance your application’s performance.
Further Reading:
Table of Contents
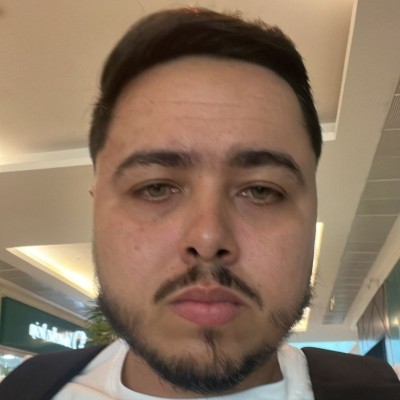
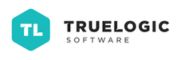