Exploring Elixir’s Ecosystem: Libraries and Frameworks
Elixir, a dynamic and functional programming language built on the Erlang VM (Virtual Machine), has been gaining steady popularity for its performance, scalability, and fault-tolerant capabilities. One of the key factors contributing to Elixir’s success is its extensive ecosystem of libraries and frameworks that empower developers to build powerful, reliable, and efficient applications. In this blog post, we’ll take a deep dive into the world of Elixir’s libraries and frameworks, exploring the essential tools that facilitate everything from web development to distributed systems.
Table of Contents
1. Introduction to Elixir’s Ecosystem
Elixir’s ecosystem is a treasure trove of libraries and frameworks that cater to various aspects of software development. Whether you’re building a web application, a distributed system, or diving into machine learning, Elixir has you covered.
2. Web Development Frameworks
2.1. Phoenix Framework: A Robust Web Framework
Phoenix is Elixir’s flagship web framework, known for its speed and reliability. It follows the Model-View-Controller (MVC) architectural pattern and supports real-time features through channels. Let’s take a quick look at a code snippet to see how simple it is to create a basic Phoenix application:
elixir # Creating a new Phoenix project mix phx.new my_app # Generating a new resource mix phx.gen.html Post posts title:string content:text # Running the server mix phx.server
Phoenix emphasizes developer productivity without sacrificing performance. It also provides tools for handling routes, views, and templates, making it an excellent choice for building dynamic web applications.
2.2. Plug: Building Composable Web Modules
Plug is a specification and set of libraries for building composable web modules in Elixir. It enables developers to create reusable components for handling HTTP requests and responses. This modular approach promotes code reusability and maintainability. Here’s a simple example of a Plug module:
elixir defmodule MyPlug do import Plug.Conn def init(options), do: options def call(conn, _opts) do conn |> put_resp_content_type("text/plain") |> send_resp(200, "Hello, Plug!") end end
With Plug, developers have fine-grained control over the request/response lifecycle, making it a versatile tool for customizing web application behavior.
3. Concurrency and Distribution Libraries
3.1. OTP: The Backbone of Elixir’s Concurrency
Elixir’s true power in concurrent and distributed programming lies in the Erlang OTP (Open Telecom Platform). OTP provides a set of abstractions, behaviors, and libraries that simplify the development of fault-tolerant, scalable, and distributed applications. One of the most crucial OTP components is the GenServer.
3.2. GenServer: Building Concurrent Stateful Modules
A GenServer is an OTP behavior that facilitates the creation of concurrent and stateful modules. It manages its own state and allows processes to communicate with it asynchronously. Here’s a simplified example of a counter implemented using GenServer:
elixir defmodule CounterServer do use GenServer def init(initial_count) do {:ok, initial_count} end def handle_call(:increment, _from, count) do new_count = count + 1 {:reply, new_count, new_count} end end # Starting the GenServer {:ok, pid} = GenServer.start_link(CounterServer, 0) # Calling GenServer functions {:ok, new_count} = GenServer.call(pid, :increment)
GenServers are the building blocks of many Elixir applications, allowing developers to create reliable and concurrent systems with ease.
4. Database Libraries
4.1. Ecto: Elixir’s SQL and NoSQL Adapter
Ecto is Elixir’s database wrapper that provides a unified API for working with databases, whether they’re SQL or NoSQL. It supports features like schema definition, query composition, and migrations. Let’s look at an example of using Ecto to interact with a PostgreSQL database:
elixir defmodule MyApp.User do use Ecto.Schema schema "users" do field :name, :string field :email, :string timestamps() end end # Querying the database users = MyApp.User |> Ecto.Query.where(name: "Alice") |> MyApp.Repo.all
Ecto abstracts the complexities of database interactions, allowing developers to focus on their application’s logic.
4.2. Redix: A Redis Client for Elixir
Redix is a high-performance Redis client for Elixir that provides a simple and intuitive API for working with Redis databases. It supports various Redis features, including transactions, pub/sub, and pipelining. Here’s an example of using Redix to interact with a Redis database:
elixir {:ok, conn} = Redix.start_link() Redix.command(conn, ["SET", "my_key", "Hello, Redix!"]) value = Redix.command(conn, ["GET", "my_key"])
Redix enables seamless integration with Redis, a popular in-memory data store.
5. Testing and Validation
5.1. ExUnit: Elixir’s Testing Framework
ExUnit is Elixir’s built-in testing framework that simplifies the process of writing and running tests. It provides expressive syntax and powerful features for unit testing, integration testing, and more. Here’s a basic example of an ExUnit test:
elixir defmodule MathTest do use ExUnit.Case test "adding numbers" do result = Math.add(2, 3) assert result == 5 end end
ExUnit encourages a test-driven development approach, ensuring code reliability and maintainability.
5.2. ESpec: BDD-style Testing for Elixir
ESpec is a behavior-driven development (BDD) testing framework for Elixir that emphasizes human-readable and descriptive tests. It allows developers to define specifications using clear language. Here’s an example of an ESpec test:
elixir defmodule MathSpec do use ESpec it "adds two numbers" do expect(Math.add(2, 3)).to eq(5) end end
ESpec enhances the collaboration between developers and stakeholders by providing tests in a more natural language format.
6. Messaging and Communication
6.1. Broadway: Data Processing and Pipelining
Broadway is a library for building concurrent, data-driven, and event-driven data processing pipelines in Elixir. It excels in scenarios where you need to process large amounts of data efficiently. Here’s a simplified example of a Broadway pipeline:
elixir defmodule MyPipeline do use Broadway defp handle_event(event, _metadata, _config) do transformed_event = transform(event) {:ok, transformed_event} end end
6.2. Broadway.start_link(MyPipeline, name: MyPipeline)
Broadway simplifies complex data processing tasks and enables efficient use of system resources.
6.3. AMQP Clients: RabbitMQ Integration
Elixir’s ecosystem includes various libraries for integrating with AMQP (Advanced Message Queuing Protocol) systems like RabbitMQ. These libraries enable you to build robust and distributed messaging systems. Here’s an example using the AMQP library to publish a message:
elixir {:ok, conn} = AMQP.Connection.open AMQP.Channel.open(conn) |> AMQP.Exchange.declare("my_exchange", :fanout) |> AMQP.Basic.publish("my_exchange", "", "Hello, AMQP!") AMQP.Connection.close(conn)
These libraries enable seamless communication between different parts of your application and external services.
7. Machine Learning and Data Science
7.1. Tensorflex: Elixir Bindings for TensorFlow
Tensorflex is an Elixir package that provides bindings to the TensorFlow library, enabling machine learning and deep learning capabilities in Elixir applications. It allows developers to build and deploy machine learning models. Here’s a simple example of using Tensorflex to load and run a pre-trained model:
elixir {:ok, model} = Tensorflex.Model.load("path/to/model") input = %{input_key: input_data} output = Tensorflex.Model.run(model, input)
Tensorflex brings the power of TensorFlow to the Elixir ecosystem, opening doors for machine learning applications.
7.2. Numerix: Numerical Computing in Elixir
Numerix is a library that brings numerical computing capabilities to Elixir. It provides functionality for working with arrays, linear algebra, and mathematical operations. Here’s an example of using Numerix to perform matrix multiplication:
elixir matrix_a = Numerix.Matrix.new([[1, 2], [3, 4]]) matrix_b = Numerix.Matrix.new([[5, 6], [7, 8]]) result = Numerix.Matrix.multiply(matrix_a, matrix_b)
Numerix enhances Elixir’s capabilities in handling complex numerical computations.
Conclusion
Elixir’s ecosystem of libraries and frameworks is a testament to its versatility and power as a programming language. From web development to distributed systems, testing to machine learning, Elixir offers a wealth of tools that streamline development and foster innovation. By exploring and utilizing these libraries and frameworks, developers can unlock Elixir’s full potential and create robust, scalable, and cutting-edge applications. So, whether you’re a seasoned Elixir developer or just embarking on your journey, the Elixir ecosystem has something valuable to offer for everyone. Happy coding!
Table of Contents
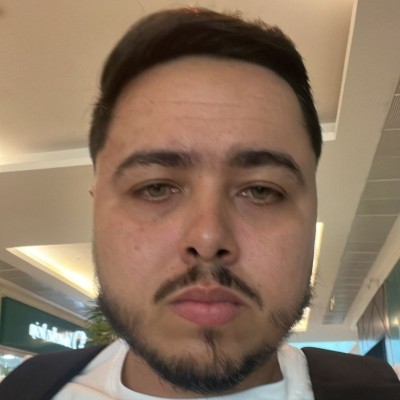
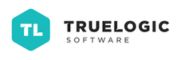