Exploring Elixir’s Registry: Dynamic Process Groups
Elixir’s Logger is a powerful tool for application logging, providing developers with flexible and efficient ways to monitor and debug their applications. Effective logging is crucial for diagnosing issues, understanding application behavior, and maintaining system health. This article explores advanced techniques for using Elixir’s Logger to enhance your application’s observability.
Using Elixir’s Logger for Advanced Logging
Elixir’s Logger module offers a rich set of features for logging, including custom log levels, metadata, and backend integrations. Here, we delve into advanced logging techniques to help you get the most out of Elixir’s Logger.
1. Configuring Logger Levels and Backends
Elixir’s Logger allows you to configure different logging levels and backends. This flexibility helps in controlling the verbosity of logs and directing them to various outputs.
Example: Configuring Logger with Different Backends
You can configure the Logger to output logs to the console, a file, or even a remote logging service. Below is an example of configuring multiple backends in the `config/config.exs` file.
```elixir config :logger, backends: [:console, {LoggerFileBackend, :file_log}], level: :info config :logger, :file_log, path: "log/app.log", level: :debug ```
In this configuration, logs are output to both the console and a file, with different levels of verbosity for each backend.
2. Adding Metadata to Logs
Metadata enhances log entries with additional context, making it easier to track down issues. You can add metadata to logs using the `metadata` option.
Example: Adding Metadata to Log Messages
```elixir require Logger defmodule MyApp do def process_request(request) do Logger.metadata(request_id: request.id) Logger.info("Processing request", request_id: request.id) # Process the request :ok end end ```
In this example, each log entry includes a `request_id` to trace specific requests through the system.
3. Customizing Log Formats
Elixir’s Logger supports customizing log formats to match your requirements. You can define a custom log format to include specific information.
Example: Customizing Log Format
```elixir config :logger, format: "$time $metadata[$level] $message\n", metadata: [:request_id] ```
In this configuration, logs are formatted to include a timestamp, log level, and message, with the `request_id` metadata included.
4. Using Log Aggregation Services
Integrating with log aggregation services can centralize your logs and provide advanced analysis capabilities. Elixir’s Logger can be configured to send logs to external services.
Example: Integrating with a Log Aggregation Service
```elixir config :logger, backends: [{LoggerRemoteBackend, :remote_log}], level: :info config :logger, :remote_log, url: "https://logs.example.com", token: "your_token_here" ```
In this setup, logs are sent to a remote service for centralized management and analysis.
5. Handling Log Rotation and Archiving
Managing log file size and rotation is essential for maintaining performance and storage. You can use libraries or custom logic to handle log rotation.
Example: Rotating Logs Using LoggerFileBackend
```elixir config :logger, :file_log, path: "log/app.log", level: :debug, format: "$time $metadata[$level] $message\n", max_size: 10_000_000, # 10 MB max_files: 5 ```
This configuration rotates log files when they reach 10 MB and keeps up to 5 archived files.
Conclusion
Elixir’s Logger module provides a robust framework for logging, offering advanced features to tailor logging to your application’s needs. From configuring multiple backends and adding metadata to customizing formats and integrating with external services, Elixir’s Logger can significantly enhance your application’s monitoring and debugging capabilities.
Further Reading:
Table of Contents
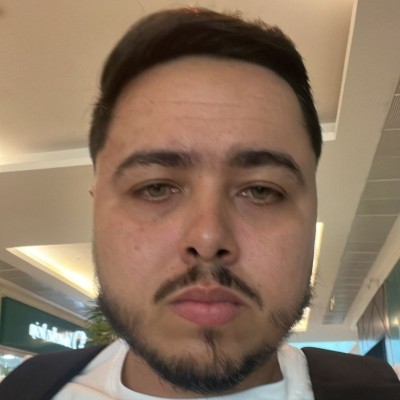
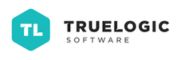