Exploring Elixir’s Metaprogramming Capabilities
Elixir is a versatile and powerful programming language known for its robustness, concurrency model, and functional paradigm. One of its unique features that sets it apart from other languages is its metaprogramming capabilities. Metaprogramming allows code to manipulate and generate other code during runtime, offering a wealth of possibilities for developers.
In this blog, we will take a deep dive into Elixir’s metaprogramming capabilities and explore how it empowers developers to write code that writes code. From macros to reflection, we will examine various tools and techniques that make Elixir a playground for advanced developers seeking elegant solutions to complex problems.
1. Understanding Metaprogramming in Elixir
Metaprogramming is the process of writing code that manipulates or generates code at compile-time or runtime. In Elixir, metaprogramming revolves around manipulating abstract syntax trees (AST) and leveraging macros to extend the language’s capabilities.
2. Macros: The Building Blocks
In Elixir, macros are functions that take the AST as input and return modified AST as output. During compilation, macros are expanded into their corresponding AST, making them an integral part of the compilation process. This allows developers to add custom syntax, define domain-specific languages (DSLs), and abstract repetitive patterns.
Let’s take a look at a simple example of a macro that enhances Elixir’s if statement:
elixir defmodule CustomConditions do defmacro unless(condition, do: block) do quote do if !unquote(condition) do unquote(block) end end end end defmodule MyModule do import CustomConditions def example_function(value) do unless value == 0 do IO.puts("Value is not zero!") end end End
In this example, we’ve defined a CustomConditions module with a macro called unless. This macro takes an expression as its first argument and a block of code as its second argument. The macro then generates the corresponding if statement with the negation of the provided expression.
By importing the CustomConditions module, we can now use the unless macro in MyModule. This enhances Elixir’s capabilities, making code more expressive and readable.
3. Metaprogramming with Macros: Use Cases
3.1 Domain-Specific Languages (DSLs)
Elixir’s metaprogramming capabilities enable the creation of domain-specific languages tailored to specific problem domains. DSLs allow developers to express complex ideas in a concise and intuitive manner, leading to more maintainable and human-readable code.
One such example is the ecto library, which provides a DSL for working with databases in Elixir. The ecto library defines macros that allow developers to write queries using Elixir syntax, making database interactions more idiomatic and less error-prone.
elixir # Ecto DSL example query = from p in Post, where: p.author == "John Doe", order_by: p.published_at, select: p.title
3.2 Code Generation
Metaprogramming in Elixir enables developers to generate boilerplate code or repetitive constructs. This simplifies code maintenance and reduces duplication. By generating code at compile-time, you ensure that any changes to the generated code are reflected across the entire application.
Consider a scenario where you need to define multiple structs for different types of products. Instead of manually writing repetitive struct definitions, you can use metaprogramming to generate the code dynamically.
elixir defmodule ProductGenerator do defmacro generate_struct(product_type) do quote do defstruct unquote(product_type) end end end defmodule MyModule do import ProductGenerator product_types = [:book, :electronics, :clothing] Enum.each(product_types, fn product_type -> generate_struct(product_type) end) end
In this example, we define a ProductGenerator module with a macro called generate_struct. This macro generates a struct definition based on the given product_type. The MyModule then uses the generate_struct macro to generate struct definitions for multiple product types.
3.3 Polymorphism and Flexibility
Metaprogramming can help in creating more flexible and polymorphic code. Through macros, you can define generic functions that adapt their behavior based on the input arguments.
elixir defmodule MathOperations do defmacro define_operation(name, operator) do quote do def unquote(name)(a, b) do unquote(operator)(a, b) end end end end defmodule Calculator do import MathOperations define_operation add, &(&1 + &2) define_operation subtract, &(&1 - &2) end
In this example, the MathOperations module defines a macro define_operation that generates functions for addition and subtraction. By leveraging metaprogramming, we can easily extend the calculator to include new operations without duplicating code.
4. Reflection: Introspecting Elixir Code
Reflection is another essential aspect of Elixir’s metaprogramming capabilities. It allows you to examine and modify the structure of your code during runtime. Elixir provides several built-in functions that enable reflection, such as __ENV__, Module.get_attribute/2, and Code.eval_quoted/3.
4.1 Using __ENV__ for Compile-Time Decisions
__ENV__ is a special variable in Elixir that provides access to the current compilation environment during macro expansion. It allows you to make compile-time decisions based on the context in which a macro is used.
elixir defmodule Debug do defmacro debug_info(msg) do if Mix.env() == :dev do quote do IO.inspect(unquote(msg)) end else quote do :ok end end end end defmodule MyApp do require Debug def some_function do Debug.debug_info("Debug message") # Rest of the function end end
In this example, the debug_info macro checks the current environment using Mix.env/0. If the environment is set to :dev, it includes an IO.inspect/1 statement, which helps developers debug their code during development. If the environment is not :dev, it simply returns :ok, effectively removing the debugging code in production.
5. Limitations and Considerations
While metaprogramming in Elixir is a powerful tool, it comes with its set of challenges and considerations:
- Debugging Complexity: Macros can introduce complexity to the codebase, making it harder to debug and understand the flow of execution.
- Readability and Maintainability: Overuse of metaprogramming can lead to obscure code that is difficult to read and maintain. Use it judiciously, and prioritize clarity.
- Compile-Time Errors: Errors in macros can be challenging to diagnose since they occur at compile-time. Carefully inspect the generated AST to identify potential issues.
- Version Compatibility: Metaprogramming heavily relies on the structure of the AST, and changes in Elixir’s future versions might affect existing macros.
Conclusion
Elixir’s metaprogramming capabilities open up a world of possibilities for developers, empowering them to write code that writes code. Through macros and reflection, you can create domain-specific languages, generate code, and build flexible and expressive applications.
However, metaprogramming is a double-edged sword that requires careful consideration and restraint. Overuse can lead to obscure code, hindering collaboration and maintainability. When used wisely, metaprogramming in Elixir becomes a powerful ally in building robust and efficient applications.
Incorporate metaprogramming into your Elixir projects, experiment with different techniques, and embrace the creativity and productivity it offers. As you delve deeper into the realm of metaprogramming, you’ll discover new ways to elevate your Elixir code and unlock its full potential. Happy coding!
Table of Contents
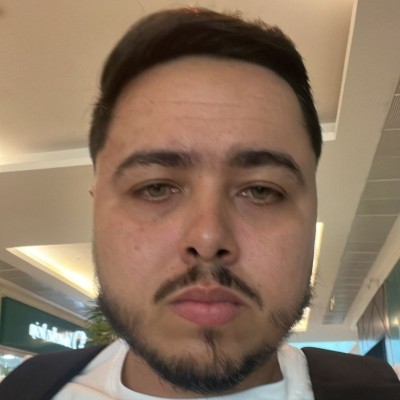
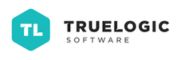