Building Microservices with Elixir and Kubernetes
In the fast-paced world of software development, building applications that can adapt and scale quickly is crucial. Microservices architecture has gained significant traction for its ability to create flexible and independently deployable services. When combined with Elixir, a functional programming language known for its fault tolerance and concurrency handling, and Kubernetes, a powerful container orchestration platform, you have a winning combination for building resilient microservices. In this article, we will dive into the world of microservices, explore the advantages of using Elixir, and see how Kubernetes can further enhance their deployment and management.
1. Microservices Architecture: A Quick Overview
Microservices architecture is an approach where applications are broken down into smaller, self-contained services that can be developed, deployed, and scaled independently. This modular approach offers benefits such as easier maintenance, improved scalability, and the ability to adopt different technologies for different services.
1.1 The Advantages of Microservices
- Scalability: Microservices can be scaled independently, allowing you to allocate resources where they are most needed.
- Technology Flexibility: Different services can use different technologies, languages, and frameworks that suit their requirements.
- Fault Isolation: If one service fails, it doesn’t necessarily impact the entire application, increasing overall system reliability.
- Independent Deployment: Microservices can be deployed and updated individually, reducing the risk of downtime for the entire application.
2. Leveraging Elixir for Microservices
Elixir, a functional programming language built on the Erlang VM, brings several advantages to the table when building microservices.
2.1 Concurrency and Fault Tolerance
Elixir’s concurrency model is based on lightweight processes that are isolated from each other and communicate through message passing. This makes it well-suited for building highly concurrent and fault-tolerant systems.
elixir # Example of spawning a new process in Elixir pid = spawn(fn -> IO.puts("Hello from process #{inspect(self())}") end)
2.2 OTP: The Secret Sauce
Elixir’s true power lies in the Open Telecom Platform (OTP) library, which provides abstractions for building fault-tolerant and distributed systems. OTP behaviors like supervisors and gen servers help manage processes and handle failures gracefully.
elixir # Example of a simple supervisor in Elixir defmodule MyApp.Supervisor do use Supervisor def start_link do Supervisor.start_link(__MODULE__, []) end def init(_) do children = [ worker(MyApp.Worker, []) ] supervise(children, strategy: :one_for_one) end end
2.3 Phoenix Framework for Web Services
For building web services in Elixir, the Phoenix framework offers a solid foundation. It provides tools for handling HTTP requests, managing sockets, and building real-time applications.
elixir # Example of a basic Phoenix route defmodule MyAppWeb.Router do use Phoenix.Router scope "/", MyAppWeb do pipe_through :browser get "/", PageController, :index end end
2.4 Code Maintainability
Pattern matching, immutability, and functional programming principles in Elixir contribute to code that is easier to reason about and maintain. This is especially valuable when dealing with a complex microservices ecosystem.
3. Kubernetes: Orchestrating Microservices
Kubernetes, often abbreviated as K8s, is a container orchestration platform that automates the deployment, scaling, and management of containerized applications.
3.1 Key Kubernetes Concepts
- Pods: The smallest deployable unit in Kubernetes, representing a single instance of a running process. Pods can contain one or more containers.
- Services: Define a set of pods and a policy to access them. Services provide a stable IP address and DNS name, enabling load balancing.
- ReplicaSets: Ensure a specified number of pod replicas are running at all times. If a pod fails, the ReplicaSet replaces it.
yaml # Example of a Kubernetes Pod configuration apiVersion: v1 kind: Pod metadata: name: myapp-pod spec: containers: - name: myapp-container image: myapp-image:v1
3.2 Scaling with Kubernetes
Kubernetes enables both manual and automatic scaling of microservices. Horizontal Pod Autoscalers (HPA) automatically adjust the number of replicas based on CPU usage or other custom metrics.
yaml # Example of a Horizontal Pod Autoscaler configuration apiVersion: autoscaling/v2beta2 kind: HorizontalPodAutoscaler metadata: name: myapp-hpa spec: scaleTargetRef: apiVersion: apps/v1 kind: Deployment name: myapp-deployment minReplicas: 2 maxReplicas: 10 metrics: - type: Resource resource: name: cpu target: type: Utilization averageUtilization: 70
3.3 Deployments for Zero Downtime
Kubernetes Deployments ensure seamless updates and rollbacks of microservices with zero downtime. By creating a new replica set and gradually shifting traffic, users won’t experience interruptions during updates.
yaml # Example of a Kubernetes Deployment configuration apiVersion: apps/v1 kind: Deployment metadata: name: myapp-deployment spec: replicas: 3 selector: matchLabels: app: myapp template: metadata: labels: app: myapp spec: containers: - name: myapp-container image: myapp-image:v2
3.4 Service Discovery and Load Balancing
Kubernetes offers built-in service discovery and load balancing. Services are assigned a DNS name, allowing microservices to communicate with each other using the service name as the hostname.
elixir # Example of Elixir code to connect to another service {:ok, conn} = HTTPoison.start response = HTTPoison.get!("http://other-service:8080/api/resource")
4. Best Practices for Building Microservices with Elixir and Kubernetes
- Keep Services Small: Design services to be focused and single-purpose, ensuring easier maintenance and scalability.
- Use Health Checks: Implement health checks in your microservices to help Kubernetes make informed scaling and routing decisions.
- Handle Failures Gracefully: Elixir’s OTP and Kubernetes’ self-healing capabilities can work in tandem to minimize service disruptions.
- Decentralized Data Management: Aim for decentralized data storage and communication to prevent bottlenecks and failures.
- Monitor and Debug: Utilize Kubernetes monitoring tools and Elixir’s telemetry library to identify and resolve issues promptly.
Conclusion
Building microservices with Elixir and Kubernetes is a powerful approach to creating resilient, scalable, and manageable applications. Elixir’s fault tolerance and concurrency, coupled with Kubernetes’ orchestration capabilities, provide a robust foundation for developing modern software systems. By following best practices and leveraging the strengths of these technologies, developers can create microservices that can adapt and thrive in today’s dynamic software landscape.
Incorporating Elixir and Kubernetes into your microservices architecture isn’t just a technological decision; it’s a strategic move towards building applications that can evolve and excel in the face of change.
So why wait? Start your journey into the world of Elixir and Kubernetes-powered microservices today!
Table of Contents
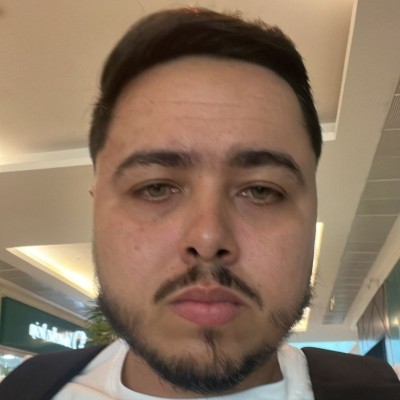
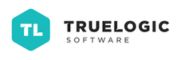