Optimizing Elixir Applications for Performance
In the realm of software development, performance optimization is a critical aspect that can make or break the success of an application. Elixir, with its robust concurrency model and fault-tolerant design, offers a unique platform for building high-performance applications. However, like any other programming language, Elixir applications can benefit from optimization techniques to enhance their efficiency further. In this article, we’ll explore several strategies for optimizing Elixir applications to achieve superior performance.
1. Understanding Elixir’s Concurrency Model:
Elixir’s concurrency model, based on the actor model and implemented through lightweight processes, allows developers to build highly concurrent and scalable systems. Leveraging this model efficiently is crucial for optimizing performance. Utilize Elixir’s `Task` module for parallelizing tasks, and employ OTP (Open Telecom Platform) behaviors like `GenServer` and `GenStage` for building robust, concurrent components within your application.
2. Minimizing Memory Consumption:
Memory management plays a significant role in the performance of any application. In Elixir, minimizing memory consumption can be achieved through various techniques:
- Use binaries and streams instead of lists for processing large datasets, as they are more memory-efficient.
- Employ ETS (Erlang Term Storage) for storing large, frequently accessed data in-memory, reducing the need for memory allocation and garbage collection.
- Optimize recursive functions to avoid unnecessary memory usage, such as tail-call optimization.
3. Profiling and Benchmarking:
Profiling and benchmarking are indispensable tools for identifying performance bottlenecks and optimizing Elixir applications:
- Utilize Erlang’s built-in tools like `:observer` and `:recon` for monitoring system metrics, process information, and memory usage.
- Use third-party libraries like `exprof` and `benchee` for profiling and benchmarking specific parts of your codebase, enabling you to pinpoint areas that require optimization.
4. Optimizing Database Queries:
Database interactions often constitute a significant portion of an application’s execution time. To optimize database queries in Elixir:
- Leverage Ecto’s query optimizations, such as preloading associations and using `Repo.transaction` for bulk operations, to minimize the number of database roundtrips.
- Utilize database indexes effectively to improve query performance, especially for frequently accessed columns or complex queries.
5. Caching and Memoization:
Caching frequently accessed data can significantly reduce computation time and improve overall application performance:
- Use libraries like `Cachex` or `ConCache` to implement in-memory caching for frequently accessed data, reducing the need for redundant computations.
- Implement memoization techniques to cache the results of expensive function calls, ensuring that subsequent invocations with the same arguments return the cached result.
6. Optimizing Network Communication:
Efficient network communication is crucial, especially in distributed systems. To optimize network communication in Elixir:
- Utilize binary protocols like Protocol Buffers or MessagePack for serializing and deserializing data, reducing overhead and improving performance.
- Implement connection pooling and asynchronous communication patterns to minimize latency and maximize throughput in network-intensive applications.
Conclusion:
Optimizing Elixir applications for performance requires a holistic approach encompassing various aspects such as concurrency, memory management, profiling, database interactions, caching, and network communication. By understanding and leveraging Elixir’s unique features and employing optimization techniques tailored to specific use cases, developers can unlock the full potential of their applications and deliver exceptional performance to users.
Table of Contents
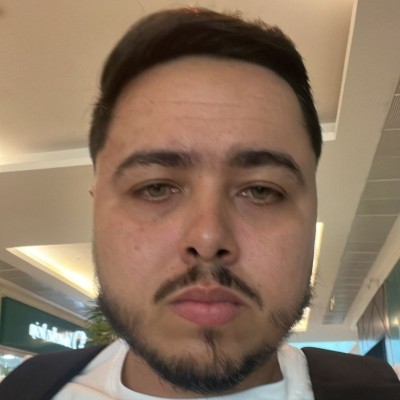
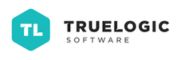