Using OTP GenStage for Event-Driven Processing in Elixir
In the realm of concurrent and distributed programming, Elixir stands as a robust choice with its unique concurrency model built on top of the battle-tested Erlang Virtual Machine (BEAM). Elixir’s OTP (Open Telecom Platform) framework provides a wealth of tools to build fault-tolerant, scalable, and highly performant applications. One of these powerful tools is GenStage, which enables event-driven processing and lays the foundation for building reactive and efficient systems.
1. Introduction to GenStage
When building applications that require efficient handling of large volumes of data or events, GenStage emerges as a vital component in your Elixir toolkit. GenStage provides a flexible and composable approach to designing event-driven data processing pipelines. Whether you’re dealing with real-time analytics, data transformation, or managing a stream of events from various sources, GenStage empowers you to model and manage the flow of data with ease.
2. Understanding GenStage Concepts
Before diving into the nitty-gritty of using GenStage, let’s establish a clear understanding of its core concepts.
2.1. Producer-Consumer Model
At the heart of GenStage lies the producer-consumer model. This pattern involves one or more data producers generating events or data and one or more consumers processing these events. GenStage provides an abstraction to define producers and consumers as separate stages in your data processing pipeline.
2.2. Demand-Driven Flow Control
GenStage employs a demand-driven flow control mechanism. Consumers signal to producers how much data they are ready to process. Producers then generate data based on the consumer’s demand. This approach ensures that consumers are not overwhelmed with data they can’t handle, promoting efficient resource utilization.
2.3. Stages and Batches
In GenStage, a stage represents a processing unit responsible for producing or consuming data. Stages can be linked together to form a processing pipeline. Each stage can operate in different modes, such as a producer, a consumer, or both.
Batches are subsets of data that producers generate in response to consumer demands. This batching mechanism enables the efficient movement of data through the pipeline while minimizing latency.
3. Benefits of Using GenStage
Integrating GenStage into your Elixir applications offers a plethora of advantages:
3.1. Scalability
GenStage enables horizontal scaling by distributing the workload across multiple stages and processes. This is particularly beneficial when dealing with high-throughput systems that need to handle a large number of events concurrently.
3.2. Back Pressure Handling
The demand-driven nature of GenStage helps prevent overload and system crashes by naturally applying back pressure. If a consumer stage is overwhelmed, it simply requests fewer events, allowing the system to maintain stability.
3.3. Fault Tolerance
Elixir’s OTP architecture, combined with GenStage, offers built-in fault tolerance. If a stage crashes, it can be restarted independently without affecting the entire pipeline. Supervision strategies ensure that your system can recover gracefully from failures.
3.4. Real-time Responsiveness
GenStage facilitates real-time data processing by allowing you to control the rate of event generation and consumption. This responsiveness is crucial for applications requiring up-to-date insights, such as monitoring systems or live dashboards.
4. Implementing GenStage: A Practical Example
Let’s walk through a practical example of implementing GenStage in Elixir. Suppose we’re building a real-time analytics system that processes user activity events and generates aggregated statistics.
4.1. Define the Event Struct
Start by defining the structure of the event you’ll be processing:
elixir defmodule UserActivityEvent do @enforce_keys [:user_id, :event_type, :timestamp] defstruct [:user_id, :event_type, :timestamp] end
4.2. Implement the Producer Stage
The producer stage generates user activity events from a source, such as a message queue or a database:
elixir defmodule UserActivityProducer do use GenStage def start_link(_) do GenStage.start_link(__MODULE__, :ok) end def init(:ok) do {:producer, %{}} end def handle_demand(demand, state) do events = fetch_events(demand) {events, state} end defp fetch_events(demand) do # Fetch `demand` number of events from the data source # and convert them to UserActivityEvent structs end end
4.3. Implement the Consumer Stage
The consumer stage aggregates user activity events and generates statistics:
elixir defmodule UserActivityConsumer do use GenStage def start_link(_) do GenStage.start_link(__MODULE__, :ok) end def init(:ok) do {:consumer, %{}} end def handle_events(events, _from, state) do statistics = process_events(events) # Send statistics to another system or module {:noreply, [], state} end defp process_events(events) do # Process the events and generate statistics end end
4.4. Connecting the Stages
Finally, connect the producer and consumer stages to form the GenStage pipeline:
elixir {:ok, _} = UserActivityProducer.start_link([]) {:ok, _} = UserActivityConsumer.start_link([]) GenStage.sync_subscribe(UserActivityConsumer, to: UserActivityProducer, max_demand: 100)
Conclusion
OTP GenStage opens up a world of possibilities for building responsive, scalable, and fault-tolerant Elixir applications. By embracing its producer-consumer model and demand-driven flow control, you can efficiently handle event-driven data processing challenges. The real-world example we’ve explored here is just the tip of the iceberg; GenStage’s flexibility allows you to design complex processing pipelines tailored to your application’s unique requirements. So, whether you’re building real-time analytics, stream processing, or any event-driven system, GenStage equips you with the tools to create systems that excel in performance and maintainability.
Incorporating GenStage into your Elixir development toolkit unlocks the potential to build highly concurrent and responsive applications that effortlessly handle the demands of the modern digital landscape. With its powerful abstractions and built-in scalability features, GenStage is a game-changer for event-driven processing in Elixir. Start experimenting with GenStage today and witness firsthand the transformation it brings to your applications. Happy coding!
Table of Contents
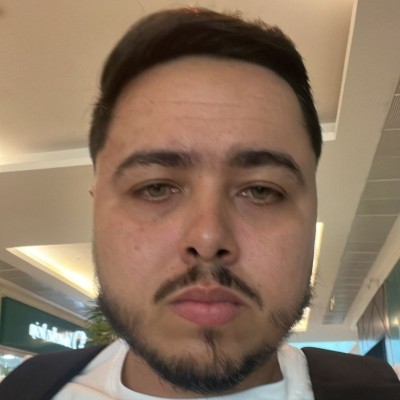
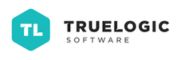