Introduction to Elixir’s OTP GenStateMachine for Stateful Processes
In the world of concurrent and distributed systems, managing state efficiently is crucial. Elixir, with its robust OTP framework, offers powerful tools for handling stateful processes. One such tool is the `GenStateMachine` behavior, which provides a way to manage state in a scalable and fault-tolerant manner. This blog explores how to use `GenStateMachine` for state management and offers practical examples to get you started.
Understanding Elixir’s OTP GenStateMachine
`GenStateMachine` is part of Elixir’s OTP framework and provides a behavior for building state machines. State machines are crucial for managing complex state transitions and maintaining a clean separation of state logic from the rest of your application. With `GenStateMachine`, you can define state transitions, handle events, and maintain state in a predictable and manageable way.
Using GenStateMachine for Stateful Processes
Elixir’s `GenStateMachine` is designed to make state management in concurrent processes straightforward. Here’s a look at some key aspects and code examples demonstrating how `GenStateMachine` can be used.
1. Defining a State Machine
To use `GenStateMachine`, you first need to define your state machine module. This involves specifying your initial state, handling state transitions, and managing events.
Example: Creating a Simple State Machine
```elixir defmodule MyStateMachine do use GenStateMachine, callback_mode: :state_functions def start_link(initial_state) do GenStateMachine.start_link(__MODULE__, initial_state, name: __MODULE__) end def init(initial_state) do {:ok, initial_state} end def handle_event(:ping, state) do {:next_state, state, "Pong"} end def handle_event(:stop, state) do {:stop, :normal, state} end end ```
In this example, `MyStateMachine` starts with an initial state and has two events: `:ping` and `:stop`. The `handle_event/2` function manages these events, transitioning between states and handling cleanup.
2. Managing State Transitions
State transitions are a core part of `GenStateMachine`. You can define different states and transitions based on events or conditions.
Example: Handling State Transitions
```elixir defmodule CounterStateMachine do use GenStateMachine, callback_mode: :state_functions def start_link(initial_count) do GenStateMachine.start_link(__MODULE__, initial_count, name: __MODULE__) end def init(initial_count) do {:ok, initial_count} end def handle_event(:increment, count) do {:next_state, count + 1, "Incremented"} end def handle_event(:decrement, count) do {:next_state, count - 1, "Decremented"} end end ```
In this `CounterStateMachine`, events `:increment` and `:decrement` adjust the count state accordingly.
3. Handling State Persistence
For state persistence, `GenStateMachine` can be combined with other OTP components like `GenServer` and `Agent`. This allows you to save state data across restarts or crashes.
Example: Using Agent for Persistence
```elixir defmodule PersistentCounter do use GenServer def start_link(_) do GenServer.start_link(__MODULE__, 0, name: __MODULE__) end def init(initial_count) do {:ok, initial_count} end def handle_call(:get, _from, state) do {:reply, state, state} end def handle_cast(:increment, state) do new_state = state + 1 {:noreply, new_state} end def handle_cast(:decrement, state) do new_state = state - 1 {:noreply, new_state} end end ```
Here, `PersistentCounter` uses `GenServer` to handle count operations, demonstrating how to combine state management with persistence.
4. Monitoring and Supervision
`GenStateMachine` can be supervised using OTP’s supervision trees. This ensures that state machines are monitored and restarted if they fail.
Example: Adding Supervision
```elixir defmodule StateMachineSupervisor do use Supervisor def start_link(_) do Supervisor.start_link(__MODULE__, :ok, name: __MODULE__) end def init(:ok) do children = [ {MyStateMachine, 0} ] Supervisor.init(children, strategy: :one_for_one) end end ```
In this example, `StateMachineSupervisor` supervises `MyStateMachine`, ensuring that it is restarted if it crashes.
Conclusion
Elixir’s `GenStateMachine` provides a powerful way to manage state in concurrent applications. By defining state transitions, managing events, and integrating with other OTP components, you can build robust and fault-tolerant stateful processes. Leveraging `GenStateMachine` effectively will help you create scalable and reliable applications.
Further Reading:
Table of Contents
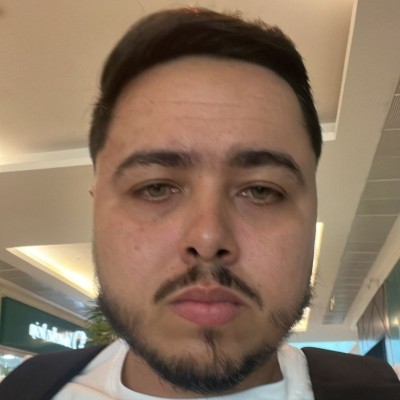
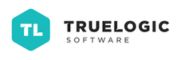