Exploring Elixir’s OTP Supervision Trees
When building robust and fault-tolerant concurrent applications, the Elixir programming language has a secret weapon – OTP Supervision Trees. OTP, which stands for “Open Telecom Platform,” is a set of libraries and design principles that allow developers to build reliable, scalable, and fault-tolerant systems with ease. One of the key components of OTP is the Supervision Tree, a hierarchical structure that manages the lifecycle of processes and ensures application stability.
In this blog post, we will take a deep dive into Elixir’s OTP Supervision Trees, understand their importance in building resilient applications, and explore how they can be used to create a solid foundation for concurrent systems.
1. Introduction to OTP and Supervision Trees
OTP (Open Telecom Platform) is not just a single library or framework; it’s a collection of battle-tested tools and conventions for building concurrent, distributed, and fault-tolerant applications in Elixir. It is based on the Actor model, where processes communicate by exchanging messages, and each process is isolated, lightweight, and independent.
Supervision Trees are a fundamental concept in OTP, designed to handle failures gracefully and ensure that applications can recover from errors without catastrophic consequences. A Supervision Tree is a hierarchical structure where each node is a process, and processes are organized into a tree-like topology.
2. The Need for Supervision Trees
In concurrent applications, failures are inevitable. Processes may crash due to bugs, external dependencies might become unavailable, or resources could be exhausted. Handling these failures is crucial to maintaining the overall stability of the system. That’s where Supervision Trees come into play.
Instead of trying to avoid failures altogether, Supervision Trees embrace the philosophy of “let it crash.” When a process crashes, it’s automatically restarted by its supervisor, which ensures that the system remains in a known state and continues to function as expected.
3. Anatomy of a Supervision Tree
3.1. Supervisors
Supervisors are processes responsible for managing other processes. They are the internal nodes of the Supervision Tree and define the strategies for restarting their child processes. If a child process crashes, the supervisor decides how to handle the failure based on the chosen restart strategy.
In Elixir, supervisors are implemented using the Supervisor behavior and can be either built-in system supervisors or custom supervisors tailored for specific application needs.
3.2. Workers
Workers are leaf nodes of the Supervision Tree. They are the actual processes responsible for performing the application’s tasks. Workers can be any Elixir process, such as GenServers, Tasks, or any custom process fulfilling a particular role.
3.3. Children
In the context of Supervision Trees, the term “children” refers to both supervisors and workers. Each supervisor is a child of its parent supervisor, forming the hierarchical structure of the tree. The children’s relationship defines the process lifecycle management within the application.
4. Starting a Supervision Tree
To create a Supervision Tree, we need to define a supervisor module that implements the Supervisor behavior. Let’s take a simple example of a key-value store, where we want to manage multiple key-value pairs as worker processes.
elixir defmodule KeyValueWorker do use GenServer def start_link(key) do GenServer.start_link(__MODULE__, key, name: String.to_atom(key)) end # GenServer callbacks here (init, handle_call, etc.) end defmodule KeyValueSupervisor do use Supervisor def start_link do Supervisor.start_link(__MODULE__, [], name: __MODULE__) end def init(_) do children = [ {KeyValueWorker, "key1"}, {KeyValueWorker, "key2"}, {KeyValueWorker, "key3"} ] supervise(children, strategy: :one_for_one) end end
In this example, KeyValueWorker is a worker process implemented using GenServer. KeyValueSupervisor is the supervisor that manages these workers using the supervise/2 function.
The supervise/2 function takes a list of child specifications and a restart strategy. In this case, we are using the :one_for_one strategy, which means that if one of the workers crashes, only that specific worker will be restarted.
To start the Supervision Tree, we can call:
elixir {:ok, _} = KeyValueSupervisor.start_link
Now, the Supervision Tree is up and running, and if any of the key-value workers crash, they will be automatically restarted.
5. Restart Strategies
When a child process crashes, supervisors need to decide how to handle the failure. OTP provides several restart strategies to choose from, based on the desired behavior of the application.
5.1. One-for-One
The :one_for_one strategy dictates that if a child process crashes, only that specific child is restarted. The rest of the children in the tree remain unaffected.
This strategy is suitable when the children are independent and their failures do not affect each other. For example, in a web server scenario, each client connection could be managed by a separate worker, and if one connection crashes, it won’t impact the others.
elixir defmodule OneForOneSupervisor do use Supervisor def init(_) do children = [ supervisor(MyWorker, []), supervisor(AnotherWorker, []) ] supervise(children, strategy: :one_for_one) end end
5.2. One-for-All
The :one_for_all strategy takes a different approach. If a child process crashes, all the other children in the tree will be terminated, and then all of them will be restarted together.
This strategy is useful when the children are interdependent, and the system needs to be in a consistent state. For example, in a database connection pool, if one connection fails, it’s better to restart all connections to ensure a clean state.
elixir defmodule OneForAllSupervisor do use Supervisor def init(_) do children = [ worker(MyWorker, []), worker(AnotherWorker, []) ] supervise(children, strategy: :one_for_all) end end
5.3. Rest-for-One
The :rest_for_one strategy is a combination of :one_for_one and :one_for_all. When a child process crashes, all the child processes that were started after it will be terminated, and then all the terminated children and the crashed child will be restarted.
This strategy is suitable for scenarios where the children have dependencies, but not all of them are affected by a single failure. For example, in a group of worker processes processing a queue, if one worker crashes, it’s likely that the workers processing the subsequent tasks will also fail due to the shared queue becoming corrupted.
elixir defmodule RestForOneSupervisor do use Supervisor def init(_) do children = [ worker(MyWorker, []), worker(AnotherWorker, []), worker(DependentWorker, []) ] supervise(children, strategy: :rest_for_one) end end
5.4. Rest-for-All
The :rest_for_all strategy is rarely used but still worth mentioning. When a child process crashes, all the child processes will be terminated, and then all of them will be restarted together.
elixir defmodule RestForAllSupervisor do use Supervisor def init(_) do children = [ worker(MyWorker, []), worker(AnotherWorker, []), worker(ThirdWorker, []) ] supervise(children, strategy: :rest_for_all) end end
6. Dynamic Supervisors
So far, we have seen examples of static Supervision Trees, where the child processes are defined at compile-time. However, Elixir’s OTP allows us to create dynamic Supervisors as well, where we can add and remove child processes at runtime.
Dynamic Supervisors provide the flexibility to adapt the Supervision Tree to changing requirements or situations. For instance, if you have a system that generates worker processes to handle incoming requests, a dynamic Supervisor can be a great fit.
Let’s take a look at how to implement a dynamic Supervisor in Elixir:
elixir defmodule DynamicSupervisorExample do use DynamicSupervisor def start_link do DynamicSupervisor.start_link(__MODULE__, [], name: __MODULE__) end def add_worker(key) do DynamicSupervisor.start_child(__MODULE__, {KeyValueWorker, [key]}) end def remove_worker(key) do worker = List.first(Enum.filter(children(__MODULE__), fn {_, pid, _} -> elem(pid, 1) == String.to_atom(key) end)) DynamicSupervisor.terminate_child(__MODULE__, elem(worker, 1)) end end
In this example, we use the DynamicSupervisor behavior instead of the regular Supervisor. The start_link/0 function starts the dynamic Supervisor. We can then use the add_worker/1 and remove_worker/1 functions to add and remove workers from the Supervisor at runtime.
7. Handling Failures in Supervision Trees
Handling failures is the core strength of Supervision Trees. When a process crashes, supervisors are equipped with restart strategies to respond effectively to the failure. However, not all failures should be handled the same way.
Supervisors provide an option to specify the maximum restart frequency and restart intensity to avoid excessive restarts, which could lead to an unstable system. The :max_restarts and :max_seconds options can be set in the supervise/2 function to define the limits for restarting children.
elixir defmodule LimitedRestartsSupervisor do use Supervisor def init(_) do children = [ worker(MyWorker, []), worker(AnotherWorker, []) ] restart = %{ restart: :transient, shutdown: 5000, type: :worker } supervise(children, strategy: :one_for_one, max_restarts: 5, max_seconds: 3600, restart: restart) end end
In this example, we set the restart strategy for the workers to :transient. It means that if a worker crashes, it will not be restarted immediately. Instead, it will be restarted only if five or fewer restarts occur within one hour (3600 seconds).
Choosing the appropriate restart strategy and setting the right limits depends on the specific requirements of your application. By customizing these options, you can fine-tune the failure handling mechanism of your Supervision Tree.
8. Application Design with Supervision Trees
One of the key aspects of building robust applications with Supervision Trees is designing the tree’s hierarchy. The way you organize supervisors and workers impacts the fault-tolerance and resilience of your application.
8.1. Creating Hierarchies
Supervision Trees can be nested to form hierarchies of supervisors and workers. The top-level supervisor usually manages application-level processes, and lower-level supervisors manage specific parts of the application.
For instance, in a web application, you might have a top-level supervisor managing processes related to the HTTP server, such as connection acceptors and request handlers. Then, you can have lower-level supervisors managing separate parts of the application, like user authentication, database interactions, and background job processing.
By designing a clear hierarchy, you can ensure that failures are isolated and contained within specific parts of the application, making it easier to identify and resolve issues.
8.2. Monitoring External Resources
Supervision Trees are not limited to managing internal processes. You can also use them to monitor and manage external resources, such as databases, APIs, or other services.
When a supervised external resource becomes unavailable, the supervisor can decide whether to restart it or take other appropriate actions, such as alerting the system administrators. This helps prevent cascading failures and allows the application to recover when external dependencies are restored.
9. Distributed OTP Applications
OTP also provides features for building distributed applications, where processes can run on multiple nodes across a network. Distributed OTP applications can benefit from the same supervision principles as local applications, ensuring fault-tolerance and stability in a distributed environment.
9.1. Node Monitoring
In a distributed system, nodes can join or leave the network dynamically. To handle such situations, OTP allows you to monitor nodes for their availability.
By monitoring nodes, supervisors can detect when a node becomes unavailable, and then decide how to handle the failure. For example, they could restart affected processes, move processes to another node, or take other actions based on the application’s requirements.
9.2. Node Failure Handling
When a node fails, all processes running on that node terminate. To avoid losing critical data or state, OTP provides mechanisms like Distributed Elixir and distributed process groups. These features allow processes to communicate and maintain their state across nodes, even if one node goes down.
By leveraging these features, you can build distributed applications that are resilient to node failures and maintain their functionality even in the face of network partitioning.
10. Best Practices and Tips
Building effective Supervision Trees requires thoughtful design and consideration of various factors. Here are some best practices and tips to keep in mind:
- Keep Supervisors Simple: Avoid complex logic in supervisors. Their main responsibility is to manage the lifecycle of child processes and restart them when necessary.
- Use Multiple Supervisors: Instead of having one massive Supervision Tree, split your application into smaller, manageable pieces with their own supervisors. This makes it easier to understand and maintain the system.
- Understand Restart Strategies: Choose the appropriate restart strategy for each child based on their dependencies and impact on other processes.
- Use Supervision Strategies Wisely: Consider using different supervision strategies (:one_for_one, :one_for_all, :rest_for_one, or :rest_for_all) for different parts of your application based on their characteristics.
- Test Failure Scenarios: Test your Supervision Tree by simulating failure scenarios to ensure it behaves as expected during real-world incidents.
- Keep Dependencies Separate: Avoid interdependent supervisors. If one supervisor manages multiple unrelated processes, consider splitting them into separate supervisors to isolate failures.
- Choose the Right :rest_for_one Children: Be cautious when using :rest_for_one, as a crash in one child can potentially terminate many others. Use it only when it’s necessary and suitable for the scenario.
- Monitor Resource Usage: Supervisors can also monitor the resource usage of their children, allowing them to take action if a process consumes excessive resources.
Conclusion
OTP Supervision Trees are a powerful mechanism that ensures the reliability and fault-tolerance of concurrent Elixir applications. By designing applications around Supervision Trees and employing appropriate restart strategies, developers can build systems that gracefully recover from failures, maintaining overall stability. With this foundation, Elixir developers can confidently explore the world of concurrent programming, knowing that OTP has their back.
Remember, embracing the “let it crash” philosophy and building Supervision Trees can be a paradigm shift in how you approach building fault-tolerant applications. The combination of Elixir’s elegant syntax and the power of OTP makes it a formidable toolset for building concurrent, scalable, and resilient applications. So go ahead, dive into the world of Elixir and OTP Supervision Trees, and unlock the true potential of concurrent programming. Happy coding!
By adopting OTP Supervision Trees, you can confidently build robust and fault-tolerant applications that can withstand failures and keep running efficiently. Elixir’s OTP is a treasure trove of powerful tools that can simplify the complexity of concurrent programming and bring a new level of reliability to your applications. So, start exploring Elixir’s OTP Supervision Trees and take your concurrent programming skills to the next level!
Table of Contents
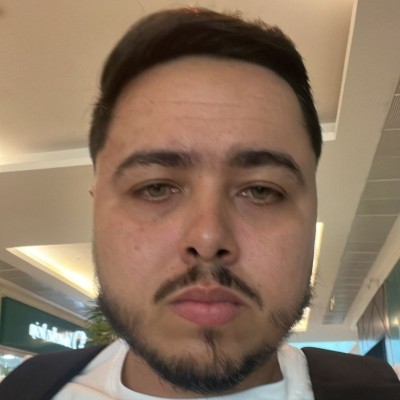
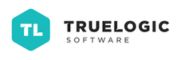