What is pattern matching in Elixir?
Pattern matching is a fundamental and powerful concept in Elixir, central to the language’s syntax and functionality. It allows developers to match values and data structures against patterns, making it easier to destructure and extract information from complex data, control program flow, and write concise and expressive code.
In Elixir, pattern matching is used extensively in various contexts, such as function definitions, case statements, and variable assignments. Here’s how it works:
- Function Definitions: When defining functions in Elixir, you can use pattern matching in the function head to specify different clauses that should be executed based on the input. Elixir will automatically select the appropriate clause based on the provided arguments. For example:
```elixir defmodule MathModule do def add(a, b) do a + b end def add(a, b, c) do a + b + c end end ```
In this example, the `add/2` function will be called for two arguments, and the `add/3` function for three arguments.
- Case Statements: The `case` statement in Elixir is used for branching control flow based on pattern matching. It allows you to match values against patterns and execute specific code blocks based on the match. For instance:
```elixir case result do {:ok, value} -> IO.puts("Success: #{value}") {:error, reason} -> IO.puts("Error: #{reason}") end ```
Here, we’re matching `result` against the patterns `{:ok, value}` and `{:error, reason}` to handle different outcomes.
- Variable Assignment: Pattern matching can be used for assigning values to variables. When the left-hand side of the assignment matches the right-hand side, the variables are bound to the corresponding values. For example:
```elixir {x, y} = {1, 2} ```
Here, `x` will be bound to `1`, and `y` to `2`.
Pattern matching in Elixir not only simplifies code but also enhances code readability and robustness. It enables developers to work with data structures effectively, destructure complex data, and handle different cases elegantly, making Elixir a language well-suited for a wide range of applications, including functional programming, concurrent systems, and data transformation tasks.
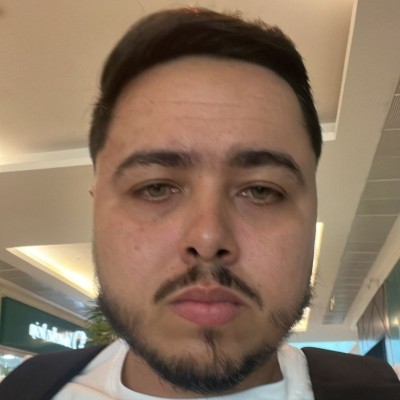
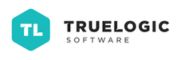