Exploring Elixir’s Pattern Matching Abilities
Elixir, a dynamic and functional programming language built on the Erlang virtual machine, has gained increasing popularity due to its concurrency model, fault tolerance, and scalability. One of its standout features is its robust pattern matching capabilities. Pattern matching in Elixir goes beyond simple comparisons; it’s a cornerstone of the language that enhances code clarity, enables powerful error handling, and promotes the functional programming paradigm. In this blog post, we’ll delve into the world of Elixir’s pattern matching, understand its various applications, and explore how it contributes to writing clean and efficient code.
1. Introduction to Pattern Matching in Elixir
1.1 What is Pattern Matching?
Pattern matching is a powerful technique that allows developers to compare data structures against predefined patterns. It goes beyond simple equality checks and opens the door to intricate comparisons. Elixir’s pattern matching supports a wide range of structures, from basic data types to more complex composite types.
1.2 Basic Pattern Matching Syntax
In Elixir, the basic pattern matching syntax is straightforward. The = symbol is used to match the left-hand side (pattern) with the right-hand side (data). Let’s take a look at a simple example:
elixir case {42, "Elixir"} do {42, language} -> IO.puts("The answer is 42 and the language is #{language}") _ -> IO.puts("Pattern doesn't match") end
In this example, the pattern {42, language} matches the tuple {42, “Elixir”}. The variable language is assigned the value “Elixir”, and the first branch of the case expression is executed.
2. Pattern Matching for Variable Assignment
2.1 Matching Single Values
Elixir’s pattern matching isn’t limited to complex data structures. It’s also used for matching single values. Take a look at this example:
elixir value = 10 case value do 5 -> IO.puts("Value is 5") 10 -> IO.puts("Value is 10") _ -> IO.puts("Value doesn't match") end
In this case, the pattern 10 matches the value stored in the variable value, and the second branch of the case expression is executed.
2.2 Destructuring Data Structures
Pattern matching becomes especially powerful when dealing with data structures like tuples and lists. Consider the following example:
elixir tuple = {100, 200} case tuple do {x, y} when x > y -> IO.puts("First element #{x} is greater than second element #{y}") {x, y} when x < y -> IO.puts("First element #{x} is less than second element #{y}") _ -> IO.puts("Pattern doesn't match") end
In this snippet, the pattern {x, y} matches the tuple {100, 200}. The variables x and y are assigned the values 100 and 200 respectively, enabling us to perform conditional checks within the branches.
2.3 Ignoring Values with Underscore
Elixir provides the underscore _ as a placeholder for values you want to ignore during pattern matching. This is particularly useful when you’re only interested in certain parts of a data structure. For instance:
elixir case {42, "Elixir"} do {42, _} -> IO.puts("The answer is 42, and the language is irrelevant") _ -> IO.puts("Pattern doesn't match") end
In this example, we’re only concerned with the fact that the first element is 42, and we’re ignoring the second element.
3. Pattern Matching in Function Definitions
3.1 Multiple Function Clauses
Pattern matching is closely tied to Elixir’s function definitions. You can define multiple function clauses with different patterns to handle various cases. Consider this function that calculates the factorial:
elixir defmodule MathUtils do def factorial(0), do: 1 def factorial(n), do: n * factorial(n - 1) end
Here, the function factorial/1 has two clauses. The first clause matches when the argument is 0 and returns 1. The second clause matches any other value of n and calculates the factorial recursively.
3.2 Guards for Fine-grained Control
Guards are additional conditions that can be applied to function clauses for more fine-grained control over pattern matching. Let’s say we want to define a function that categorizes numbers into “small,” “medium,” and “large” based on their values:
elixir defmodule NumberCategorizer do def classify(n) when n < 10, do: "small" def classify(n) when n >= 10 and n < 100, do: "medium" def classify(n) when n >= 100, do: "large" end
Here, the guards when n < 10, when n >= 10 and n < 100, and when n >= 100 allow us to precisely classify the input n into the appropriate category.
3.3 Handling Edge Cases with Pattern Matching
Pattern matching also excels at handling edge cases. Let’s say we want to write a function that calculates the nth Fibonacci number:
elixir defmodule Fibonacci do def fib(0), do: 0 def fib(1), do: 1 def fib(n) when n > 1, do: fib(n - 1) + fib(n - 2) end
In this example, the function fib/1 has three clauses. The first two clauses handle the base cases where n is 0 or 1. The third clause, with the guard when n > 1, performs the recursive calculation for larger values of n.
4. Pattern Matching in Lists and Tuples
4.1 Matching List Contents
Pattern matching can be used to extract and manipulate elements within lists. Let’s say we want to implement a function that prints the elements of a list:
elixir defmodule ListPrinter do def print_list([]), do: IO.puts("List is empty") def print_list([head | tail]) do IO.puts("Element: #{head}") print_list(tail) end end
Here, the function print_list/1 has two clauses. The first clause matches an empty list and prints a corresponding message. The second clause matches a non-empty list, extracting the head and tail using the pattern [head | tail] and recursively printing the remaining elements.
4.2 Extracting Tuple Elements
Pattern matching simplifies the extraction of data from tuples as well. Let’s implement a function that calculates the area and perimeter of a rectangle:
elixir defmodule RectangleUtils do def area({width, height}), do: width * height def perimeter({width, height}), do: 2 * (width + height) end
In this example, the patterns {width, height} in the function clauses allow us to extract the dimensions of the rectangle and perform the corresponding calculations.
4.3 Recursive Pattern Matching
Pattern matching is particularly useful for recursive data structures like lists. Let’s say we want to implement a function that sums up the elements of a nested list:
elixir defmodule NestedListSum do def sum([]), do: 0 def sum([head | tail]) when is_list(head), do: sum(head) + sum(tail) def sum([head | tail]), do: head + sum(tail) end
Here, the first clause matches an empty list and returns 0. The second clause matches a list where the head is another list, recursively calculating the sum of the nested list and continuing with the tail. The third clause matches a list with a non-list head, simply adding the head to the sum of the tail.
5. Pattern Matching in Control Structures
5.1 Case Statements for Complex Matching
Elixir’s case statement allows for more complex pattern matching scenarios. Consider a simple example of a traffic light simulator:
elixir defmodule TrafficLight do def describe_color("R"), do: "Red light, stop!" def describe_color("Y"), do: "Yellow light, prepare to stop" def describe_color("G"), do: "Green light, go!" def describe_color(color), do: "Unknown color: #{color}" end
Here, the case statement is used to match different color codes and provide appropriate descriptions.
5.2 Leveraging Pattern Matching in Cond Expressions
Elixir’s cond expression provides a way to chain multiple conditions and perform pattern matching at each step. Let’s say we want to categorize weather conditions:
elixir defmodule WeatherAnalyzer do def analyze("sunny"), do: "Enjoy the sunshine!" def analyze("rainy"), do: "Don't forget your umbrella" def analyze("cloudy"), do: "Could rain, better be prepared" def analyze(_), do: "Weather conditions unknown" end
In this example, the cond expression enables us to handle various weather conditions effectively.
5.3 Error Handling and Pattern Matching
Pattern matching can enhance error handling by providing clear and targeted error messages. Consider a function that calculates the square root of a number, but only for positive inputs:
elixir defmodule SafeMath do def sqrt(n) when n >= 0, do: :math.sqrt(n) def sqrt(n), do: {:error, "Cannot calculate square root of negative number"} end
By matching the condition n >= 0 in the first clause, we ensure that only non-negative numbers are processed for square root calculations.
6. Pattern Matching in Map Structures
6.1 Matching Map Keys and Values
Pattern matching isn’t limited to tuples and lists—it extends to map structures as well. Let’s say we want to analyze different types of vehicles:
elixir defmodule VehicleAnalyzer do def analyze(%{type: "car", brand: brand}), do: "This is a #{brand} car" def analyze(%{type: "bike"}), do: "This is a bike" def analyze(%{type: _}), do: "Unknown vehicle type" end
In this case, we’re matching the keys and values within a map to provide relevant information about the vehicle.
6.2 Updating Map Values Conditionally
Pattern matching can also be used to update map values conditionally. Consider a scenario where you want to implement a discount mechanism for different products:
elixir defmodule DiscountManager do def apply_discount(%{product: "shoes", price: price} = item) when price >= 50 do %{item | price: price * 0.8} end def apply_discount(item), do: item end
In this example, the pattern match (%{product: “shoes”, price: price} = item) extracts the product and price fields from the map and applies a discount if the price is above a certain threshold.
6.3 Combining Pattern Matching with Map Updates
Pattern matching can be combined with map updates to create powerful transformations. Let’s say we want to implement a function that increments the quantity of a product in a shopping cart:
elixir defmodule ShoppingCart do def increment_quantity(cart, product) do case Map.update!(cart, product, 1, fn quantity -> quantity + 1 end) do {:ok, updated_cart} -> updated_cart {:error, reason} -> IO.puts("Error: #{reason}") cart end end end
Here, we’re using pattern matching within the Map.update!/3 function to modify the quantity of the specified product in the cart.
7. Pattern Matching in Concurrency and Processes
7.1 Message Pattern Matching in Processes
Elixir’s pattern matching extends to its concurrency model and processes. When dealing with message passing between processes, pattern matching enables selective handling of messages. Consider a simple message dispatcher:
elixir defmodule MessageDispatcher do def handle_messages do receive do {:info, message} -> IO.puts("Info: #{message}") handle_messages() {:warning, message} -> IO.puts("Warning: #{message}") handle_messages() {:error, message} -> IO.puts("Error: #{message}") handle_messages() _ -> IO.puts("Unknown message") handle_messages() end end end
Here, different types of messages are pattern matched and processed accordingly.
7.2 Ensuring Correct Message Handling
Pattern matching in processes ensures that the right process handles the right messages. Consider a scenario with multiple workers processing tasks:
elixir defmodule TaskManager do def start_link(worker_count) do {:ok, _pid} = Task.Supervisor.start_link(__MODULE__, worker_count: worker_count) end def init(%{worker_count: worker_count}) do children = Enum.map(1..worker_count, fn _ -> {TaskWorker, []} end) {:ok, %{worker_count: worker_count}, children} end def dispatch_task(pid, task) do Task.Supervisor.async_nolink(pid, fn _ -> TaskWorker.process_task(task) end) end end defmodule TaskWorker do def process_task(task) do # Process the task here end end
In this example, the TaskManager ensures that each task is dispatched to an available TaskWorker process, leveraging pattern matching to ensure proper message distribution.
7.3 Supervision Trees and Fault Tolerance
Elixir’s pattern matching plays a crucial role in supervision trees, a fundamental concept for building fault-tolerant systems. A supervisor process uses pattern matching to match specific error types and take corrective actions. For instance:
elixir defmodule WorkerSupervisor do use Supervisor def start_link do Supervisor.start_link(__MODULE__, []) end def init(_args) do children = [ worker(TaskWorker, [], restart: :permanent, max_restarts: 3, max_seconds: 5) ] supervise(children, strategy: :one_for_one) end end
Here, the supervisor is configured to restart a failing TaskWorker up to three times within a five-second window. The supervisor pattern matches the error condition to determine the appropriate response.
Conclusion
Elixir’s pattern matching abilities elevate the language to new heights, enabling developers to write elegant, efficient, and error-resistant code. From variable assignments and function definitions to control structures, data structures, and concurrency handling, pattern matching is a central feature that empowers developers to create expressive and maintainable programs. By exploring Elixir’s pattern matching capabilities, you’ve gained a powerful tool to tackle complex programming challenges while adhering to the principles of functional programming. So go forth, leverage pattern matching, and unlock the full potential of Elixir for your next project!
Table of Contents
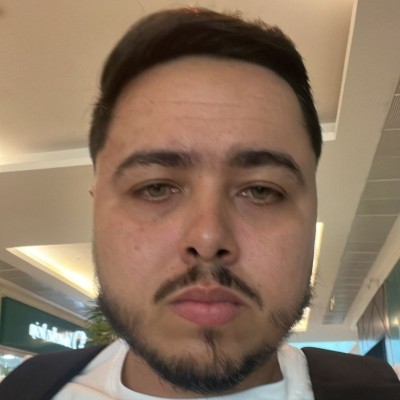
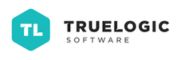