Getting Started with Elixir’s Phoenix Framework
Elixir’s Phoenix Framework is a robust and high-performance web development framework built on the functional programming language, Elixir. If you’re looking to build scalable and fault-tolerant web applications, Phoenix is the perfect choice. In this blog, we’ll take you through the essential steps to get started with Phoenix, from installation to creating your first web application. Let’s dive in!
1. What is Elixir’s Phoenix Framework?
Elixir’s Phoenix Framework is a full-stack web framework built on top of the Elixir language. It follows the model-view-controller (MVC) architectural pattern and embraces functional programming principles. Phoenix is designed for high concurrency and performance, making it an ideal choice for building real-time applications.
2. Prerequisites for Getting Started
Before diving into Phoenix, you need to set up your development environment. Ensure you have the following components installed:
2.1. Installing Elixir:
Phoenix requires Elixir, so the first step is to install it on your system. You can find installation instructions on the official Elixir website.
2.2. Setting Up Phoenix:
Once you have Elixir installed, you can install Phoenix using the mix package manager. Open your terminal and run the following command:
bash mix archive.install hex phx_new
This command installs the Phoenix mix archive, enabling you to generate new Phoenix projects with ease.
3. Creating a New Phoenix Project
Now that you have Phoenix installed, let’s create a new project.
3.1. Generating a New Project:
Use the following command to create a new Phoenix application:
bash mix phx.new my_app
Replace my_app with the desired name of your application. Phoenix will set up the project structure and fetch the necessary dependencies.
3.2. Understanding the Project Structure:
Phoenix projects follow a well-defined structure to maintain code organization. Here’s an overview of the essential directories:
- config: Contains configuration files for different environments.
- lib: Holds your application-specific code.
- priv: Contains private files, like static assets.
- web: Contains web-related components like controllers, views, and templates.
- test: Holds test files.
4. Building Your First Web Application
Now that we have our project set up, let’s start building a basic web application.
4.1. Creating Routes:
In Phoenix, routes define how URLs are mapped to controllers and actions. Open the file web/router.ex and add a new route like this:
elixir get "/", PageController, :index
This route maps the root URL to the index action of PageController.
4.2. Implementing Controllers and Views:
Controllers handle incoming requests and interact with the application’s models. Views, on the other hand, manage templates and render data to be sent to the client. Let’s generate a controller and a view:
bash mix phx.gen.html Accounts User users name:string email:string
This command generates a User model, a UserController, and related views for creating, updating, and deleting users.
4.3. Working with Templates:
Phoenix uses EEx (Embedded Elixir) templates for rendering HTML. You can find the templates in the web/templates directory. Customize the generated templates according to your application’s needs.
5. Managing Data with Ecto
Ecto is Phoenix’s built-in database wrapper that enables you to work with databases easily.
5.1. Setting Up the Database:
In your Phoenix project, locate the file config/dev.exs and configure your database settings, such as the database name, username, and password.
elixir config :my_app, MyApp.Repo, adapter: Ecto.Adapters.Postgres, username: "your_username", password: "your_password", database: "my_app_dev", hostname: "localhost"
5.2. Defining and Migrating Models:
In the web/models directory, you’ll find the file user.ex. This is where you define the schema for the User model. Once the schema is defined, run the following command to create the database table:
bash mix ecto.create mix ecto.migrate
5.3. Querying the Database:
With the database set up, you can now perform CRUD operations on the User model. Use Ecto functions like Repo.insert/2, Repo.update/2, Repo.delete/2, and Repo.get/3 to interact with the database.
6. Adding Real-Time Functionality with Channels
Phoenix channels allow you to build real-time features like chat applications, notifications, and more.
6.1. Installing Phoenix Presence:
Presence is an essential feature for tracking user connections. To use it, add phoenix_pubsub and phoenix_presence to your application’s dependencies in mix.exs.
elixir defp deps do [ {:phoenix_pubsub, "~> 2.0"}, {:phoenix_presence, "~> 1.0"} ] end
Then, run mix deps.get to fetch the new dependencies.
6.2. Creating Channels:
In Phoenix, a channel is a communication layer between the client and server. Generate a channel using the following command:
bash mix phx.gen.channel Chat
This creates a ChatChannel that facilitates real-time chat functionality.
6.3. Handling Channel Messages:
In the generated ChatChannel, you can implement various callback functions to handle different channel events like join, handle_in, handle_out, etc.
7. Enhancing the User Experience with LiveView
Phoenix LiveView enables rich, interactive user experiences without writing client-side JavaScript.
7.1. Installing LiveView:
To use LiveView, add it as a dependency in your mix.exs file:
elixir defp deps do [ {:phoenix_live_view, "~> 0.16"} ] end
Then, run mix deps.get to fetch the new dependency.
7.2. Creating LiveViews:
Generate a LiveView using the following command:
bash mix phx.gen.live Page About
This generates a LivePage module for the “about” page.
7.3. Handling User Interactions:
In the generated LiveView module, you can implement event handlers and update functions to respond to user interactions and update the view accordingly.
8. Deploying Your Phoenix Application
To deploy your Phoenix application, follow these steps:
8.1. Preparing for Deployment:
Ensure you have a production configuration set up in the config directory, e.g., config/prod.exs. Adjust database settings, secrets, and other configurations as needed.
8.2. Deploying to Heroku:
Heroku is a popular platform for deploying Elixir applications. Use the following steps to deploy your Phoenix app:
- Create a Heroku account and install the Heroku CLI.
- Set up a Git repository for your Phoenix project if you haven’t already.
- Login to Heroku from your terminal using heroku login.
- Create a new Heroku app with heroku create.
- Push your code to Heroku with git push heroku main.
- Run the database migrations on Heroku using heroku run “mix ecto.migrate”.
Your Phoenix app should now be live on Heroku!
Conclusion:
Elixir’s Phoenix Framework is a powerful tool for building high-performance web applications. In this blog, we covered the basics of getting started with Phoenix, from installation to deploying your application. As you continue your journey with Phoenix, you’ll discover its scalability, real-time capabilities, and elegant code structure that make it an excellent choice for web development projects of all sizes. Happy coding!
Table of Contents
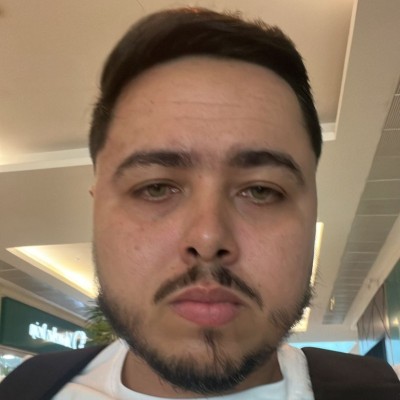
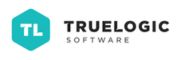