Introduction to Elixir’s Plug: Building Modular Web Applications
Elixir is renowned for its concurrent, fault-tolerant nature, making it a powerful language for building scalable applications. One of its key components for web development is Plug, a specification and library for composable modules in web applications. This blog explores how Plug can be utilized to create modular and efficient web applications in Elixir.
Understanding Elixir’s Plug
Plug is a specification for composing and building web applications in Elixir. It provides a standardized interface for handling HTTP requests and responses, allowing for modularity and extensibility in web application development. Plug’s design enables developers to create reusable components, making it easier to manage and scale web applications.
Using Plug for Modular Web Application Development
Plug’s flexibility and composability make it an excellent choice for developing modular web applications. Below are key aspects and code examples demonstrating how to leverage Plug in Elixir for building web applications.
1. Creating a Simple Plug Module
To start using Plug, you need to create a module that implements the Plug behavior. This module will handle HTTP requests and perform operations such as request logging, parameter parsing, or authentication.
Example: Creating a Logging Plug
Here’s a simple Plug module that logs request details:
```elixir defmodule MyApp.LoggerPlug do import Plug.Conn def init(opts), do: opts def call(conn, _opts) do IO.puts("Request received: #{inspect(conn.method)} #{inspect(conn.request_path)}") conn end end ```
2. Composing Plugs in a Plug Pipeline
Plug supports composing multiple modules into a pipeline, allowing for sequential processing of requests.
Example: Defining a Plug Pipeline
You can define a pipeline in your Phoenix application or a simple Plug-based web server.
```elixir defmodule MyApp.Router do use Plug.Router plug MyApp.LoggerPlug plug :match plug :dispatch get "/" do send_resp(conn, 200, "Hello, world!") end match _ do send_resp(conn, 404, "Not Found") end end ```
3. Handling HTTP Requests with Plug
Plug enables you to define how requests should be handled, including routing and response generation.
Example: Basic Request Handling
In the example above, the `MyApp.Router` module uses Plug to handle HTTP GET requests to the root path. You can expand this to handle more complex routing and request processing.
```elixir defmodule MyApp.Router do use Plug.Router plug :match plug :dispatch get "/" do send_resp(conn, 200, "Welcome to MyApp!") end post "/data" do data = Plug.Conn.read_body(conn) send_resp(conn, 200, "Received data: #{data}") end end ```
4. Integrating Plug with Phoenix Framework
Phoenix is a popular web framework in the Elixir ecosystem that integrates seamlessly with Plug. You can use Plug modules in Phoenix controllers, routers, and pipelines.
Example: Using Plug in Phoenix
In a Phoenix application, you can include custom Plug modules in your router or endpoint:
```elixir defmodule MyApp.Endpoint do use Phoenix.Endpoint, otp_app: :my_app plug MyApp.LoggerPlug plug Plug.Logger plug Plug.Static, at: "/", from: :my_app, gzip: true plug Plug.Session, store: :cookie, key: "_my_app_key", signing_salt: "random_salt" plug MyApp.Router end ```
Conclusion
Elixir’s Plug offers a robust framework for building modular and scalable web applications. By leveraging Plug’s capabilities, developers can create reusable components, manage request handling efficiently, and integrate seamlessly with the Phoenix framework. This modular approach not only simplifies development but also enhances the maintainability and scalability of web applications.
Further Reading:
Table of Contents
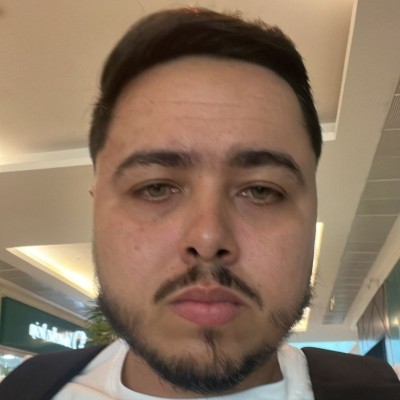
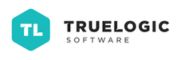