Building Reactive Web Applications with Elixir and Phoenix LiveView
Elixir, with its concurrent and fault-tolerant nature, combined with Phoenix LiveView, offers a powerful framework for building reactive web applications. This blog delves into how Elixir and Phoenix LiveView can be leveraged to create dynamic, real-time web experiences, providing practical examples and insights into this modern stack.
Understanding Phoenix LiveView
Phoenix LiveView is a library for building interactive, real-time user interfaces in Elixir applications. It enables developers to create highly responsive web applications without writing JavaScript, leveraging server-side rendering and WebSocket connections to push updates to clients.
Using Elixir and Phoenix LiveView for Reactive Web Applications
Elixir’s concurrency model and Phoenix’s web framework, combined with LiveView, provide a robust foundation for developing reactive web applications. Below are key aspects and examples of how to use these technologies effectively.
1. Setting Up a Phoenix LiveView Project
To get started with Phoenix LiveView, you need to set up a Phoenix project and add the necessary dependencies.
Example: Creating a New Phoenix Project
```bash mix phx.new my_reactive_app cd my_reactive_app ```
Add `phoenix_live_view` to your mix.exs file:
```elixir defp deps do [ {:phoenix, "~> 1.6.0"}, {:phoenix_live_view, "~> 0.17.0"}, Other dependencies ] end ```
Run `mix deps.get` to fetch the new dependencies.
2. Creating a LiveView
LiveViews are used to manage real-time updates and interactions within your application. You create a LiveView module and a corresponding template to define the dynamic content.
Example: Building a LiveView for a Real-Time Chat
LiveView Module (`lib/my_reactive_app_web/live/chat_live.ex`):
```elixir defmodule MyReactiveAppWeb.ChatLive do use Phoenix.LiveView def render(assigns) do ~L""" <div id="chat"> <div id="messages"> <%= for message <- @messages do %> <p><%= message %></p> <% end %> </div> <input type="text" phx-debounce="500" phx-keyup="send_message" placeholder="Type your message..."> </div> """ end def mount(_params, _session, socket) do {:ok, assign(socket, messages: [])} end def handle_event("send_message", %{"value" => message}, socket) do {:noreply, update(socket, :messages, &([message | &1]))} end end ```
Template (`lib/my_reactive_app_web/templates/live/chat_live.html.leex`):
```html <%= live_render(@conn, MyReactiveAppWeb.ChatLive) %> ```
3. Handling Real-Time Updates
Phoenix LiveView provides a way to push updates to the client without requiring a full page refresh. You can use LiveView’s `handle_event` and `handle_info` functions to manage real-time data and interactions.
Example: Updating LiveView with New Data
In the Chat LiveView example above, the `handle_event` function is used to capture and display new messages in real-time. This function updates the `:messages` assign in the LiveView, which automatically triggers a re-render on the client side.
4. Integrating with External APIs
You might need to integrate your LiveView with external APIs to fetch or push data. Elixir’s `HTTPoison` or `Tesla` libraries can be used for this purpose.
Example: Fetching Data from an External API
```elixir defmodule MyReactiveAppWeb.DataFetcher do use HTTPoison.Base def fetch_data do case get("https://api.example.com/data") do {:ok, %{body: body}} -> {:ok, Jason.decode!(body)} {:error, _reason} -> {:error, "Failed to fetch data"} end end end ```
Incorporate this function into your LiveView to update the data displayed in real-time.
5. Optimizing Performance
Performance is crucial for reactive web applications. Phoenix LiveView is designed to be efficient, but you can further optimize performance by minimizing the amount of data sent to the client and using efficient querying and data handling practices.
Example: Debouncing Input Events
Debouncing user input can reduce the number of events processed by the server. In the chat example, the `phx-debounce` attribute is used to limit the frequency of `phx-keyup` events.
Conclusion
Elixir and Phoenix LiveView offer a compelling stack for building reactive web applications with real-time features. By leveraging these technologies, you can create dynamic and interactive web experiences with less reliance on client-side JavaScript and more focus on server-side logic.
Further Reading:
Table of Contents
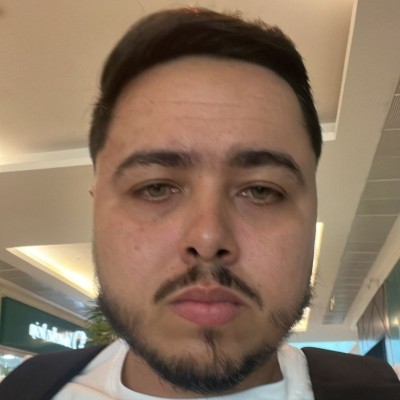
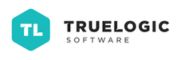