Building a Real-Time Auction System with Elixir and Phoenix Channels
Elixir, known for its concurrency and fault-tolerance, combined with Phoenix Channels, offers a robust solution for real-time web applications. This article explores how to leverage Elixir and Phoenix Channels to build a real-time auction system, providing practical examples and detailed explanations of each step.
Understanding Real-Time Auction Systems
A real-time auction system requires real-time updates to ensure all participants have current information. This involves real-time bidding, live updates of auction items, and instant notifications for auction events. Phoenix Channels, part of the Phoenix framework, is designed to handle such requirements efficiently with WebSocket support and real-time messaging.
Setting Up the Project
To get started, you’ll need to set up a Phoenix project with Elixir. Follow these steps:
1. Install Elixir and Phoenix:
Ensure you have Elixir and Phoenix installed. If not, you can find installation instructions on the [Phoenix website](https://www.phoenixframework.org/).
2. Create a New Phoenix Project:
```bash mix phx.new auction_system --no-ecto cd auction_system ```
3. Install Dependencies:
```bash mix deps.get ```
Implementing Real-Time Features
1. Creating a Live Auction Channel
Channels in Phoenix allow you to push real-time updates to clients. Let’s create a channel for the auction system.
Example: Auction Channel
```elixir # lib/auction_system_web/channels/auction_channel.ex defmodule AuctionSystemWeb.AuctionChannel do use AuctionSystemWeb, :channel def join("auction:lobby", _payload, socket) do {:ok, socket} end def handle_in("bid", %{"amount" => amount}, socket) do broadcast!(socket, "new_bid", %{amount: amount}) {:noreply, socket} end end ```
Example: Channel Setup in Endpoint
```elixir # lib/auction_system_web/endpoint.ex socket "/socket", AuctionSystemWeb.UserSocket, websocket: true, longpoll: false ```
2. Building the Frontend with LiveView
Phoenix LiveView enables interactive, real-time user interfaces without needing JavaScript. Use LiveView to handle real-time updates in the auction UI.
Example: Auction LiveView
```elixir # lib/auction_system_web/live/auction_live.ex defmodule AuctionSystemWeb.AuctionLive do use Phoenix.LiveView def render(assigns) do ~L""" <div> <h1>Auction</h1> <div id="bids"> <%= for bid <- @bids do %> <p><%= bid.amount %></p> <% end %> </div> <button phx-click="place_bid">Place Bid</button> </div> """ end def handle_event("place_bid", _params, socket) do # Logic to place a bid and broadcast to channel {:noreply, socket} end def handle_info({:new_bid, bid}, socket) do # Update the LiveView with new bid {:noreply, assign(socket, :bids, [bid | socket.assigns.bids])} end end ```
3. Handling Real-Time Updates
Use Phoenix Channels to push updates to the client side whenever a bid is placed. Here’s how to integrate channel messages with your LiveView.
Example: JavaScript Client
```javascript // assets/js/socket.js import { Socket } from "phoenix"; let socket = new Socket("/socket", { params: { userToken: window.userToken } }); socket.connect(); let channel = socket.channel("auction:lobby", {}); channel.join() .receive("ok", () => console.log("Joined auction channel")) .receive("error", (reason) => console.log("Failed to join channel", reason)); channel.on("new_bid", payload => { console.log("New bid received", payload); // Update the UI with new bid }); ```
Integrating with a Database (Optional)
While this guide focuses on real-time features, integrating with a database can be useful for persistent storage and more complex operations. If needed, you can use Ecto with Phoenix for database management.
Conclusion
Building a real-time auction system with Elixir and Phoenix Channels offers a powerful way to handle high-performance, real-time interactions. By leveraging the concurrency and fault-tolerance of Elixir and the real-time capabilities of Phoenix Channels, you can create a dynamic and responsive auction platform. This guide provides the foundational steps and code examples to help you get started with developing your own real-time auction system.
Further Reading:
Table of Contents
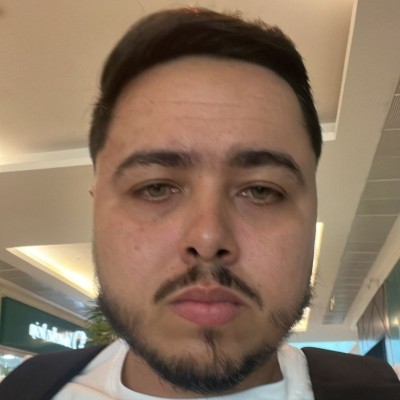
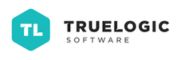