Exploring Elixir’s Registry: Dynamic Process Groups
In the world of concurrent programming, managing dynamic process groups efficiently is crucial for building scalable and resilient systems. Elixir, a functional programming language built on the Erlang VM, offers robust tools for this purpose, with the `Registry` module being a standout feature. This article explores how Elixir’s Registry can be leveraged to manage dynamic process groups effectively, with practical examples to illustrate its capabilities.
Understanding Elixir’s Registry
Elixir’s Registry is a module that provides a simple and flexible mechanism for managing dynamic process groups. It allows processes to register themselves under a unique name, facilitating easy lookup and interaction with those processes. This is particularly useful in scenarios where processes need to be dynamically grouped and managed, such as in distributed systems and fault-tolerant applications.
Using Elixir’s Registry for Dynamic Process Management
Elixir’s Registry module offers several features that make it suitable for managing dynamic process groups. Below are some key aspects and code examples demonstrating how to use the Registry for dynamic process management.
1. Setting Up a Registry
The first step in using Elixir’s Registry is to set it up. You can define and start a Registry process as part of your application’s supervision tree.
Example: Defining and Starting a Registry
```elixir In your application's supervision tree defmodule MyApp.Application do use Application def start(_type, _args) do children = [ {Registry, keys: :unique, name: MyApp.Registry} Other children ] opts = [strategy: :one_for_one, name: MyApp.Supervisor] Supervisor.start_link(children, opts) end end ```
2. Registering Processes
Once the Registry is set up, you can register processes under unique names. This allows other processes to look up and interact with these registered processes.
Example: Registering a Process
```elixir defmodule MyProcess do use GenServer def start_link(name) do GenServer.start_link(__MODULE__, name, name: name) end def init(name) do {:ok, name} end def handle_call(:get_name, _from, name) do {:reply, name, name} end end Registering a process {:ok, _pid} = MyProcess.start_link({MyApp.Registry, "my_process"}) ```
3. Looking Up Registered Processes
You can look up registered processes using the names under which they were registered. This is useful for interacting with processes dynamically.
Example: Looking Up and Interacting with a Registered Process
```elixir defmodule ProcessLookup do def get_process_name(pid) do case GenServer.call(pid, :get_name) do {:ok, name} -> IO.puts("Process name: {name}") :error -> IO.puts("Process not found") end end end Example usage ProcessLookup.get_process_name({MyApp.Registry, "my_process"}) ```
4. Using Registry for Dynamic Process Groups
Registry is particularly powerful for managing dynamic process groups. You can use it to group related processes and perform operations on those groups.
Example: Creating and Managing a Dynamic Process Group
```elixir defmodule DynamicGroup do def start_process(name) do {:ok, pid} = MyProcess.start_link(name) Registry.register(MyApp.Registry, name, pid) end def get_process(name) do case Registry.lookup(MyApp.Registry, name) do [{pid, _}] -> {:ok, pid} [] -> :error end end end Example usage DynamicGroup.start_process("group_process_1") {:ok, pid} = DynamicGroup.get_process("group_process_1") ```
Conclusion
Elixir’s Registry module provides a powerful mechanism for managing dynamic process groups, enhancing concurrency and fault tolerance in applications. By setting up a Registry, registering processes, looking them up, and managing process groups, you can build scalable and resilient systems in Elixir. Leveraging these capabilities effectively will lead to more robust and maintainable applications.
Further Reading:
Table of Contents
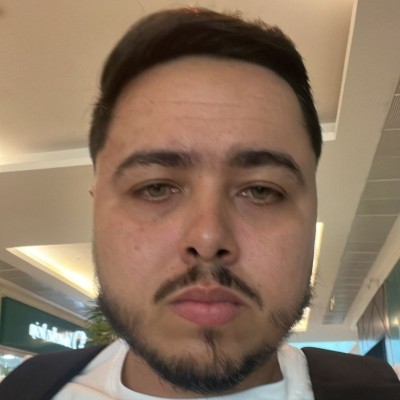
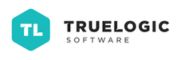