How to implement a RESTful API in Phoenix?
Creating a RESTful API in Phoenix, a popular Elixir web framework, is a straightforward process. Here are the key steps to implement a RESTful API:
- Set Up Your Phoenix Project:
Start by creating a new Phoenix project using the `mix phx.new` command. Choose the `–no-ecto` option if you don’t need a database for your API. This will generate the basic project structure.
- Define Your Routes:
In the `lib/your_app_web/router.ex` file, define the API routes using the `scope` and `resources` functions. For example:
```elixir scope "/api", YourAppWeb do pipe_through :api resources "/items", ItemController end ```
This sets up routes for CRUD operations on items, assuming you have an `ItemController`.
- Create Controllers:
Generate controllers for your API resources using `mix phx.gen.json`. For example:
```bash mix phx.gen.json Items Item items name:string description:string ```
This command creates a controller with CRUD actions (index, show, create, update, delete) and a corresponding context for managing items.
- Implement Actions:
In your generated controller (`lib/your_app_web/controllers/item_controller.ex`), implement actions to handle requests. You’ll have functions like `index`, `show`, `create`, `update`, and `delete`, which respond to HTTP requests and interact with your data.
- Define Your Data Model:
If your API requires data storage, define your data model using Ecto schemas and migrations. If not, you can skip this step.
- Configure CORS (Cross-Origin Resource Sharing):
To allow requests from different origins, configure CORS settings in your `config/config.exs` file. Add the necessary CORS middleware and specify allowed origins, headers, and methods.
- Request and Response Handling:
Use the `conn` struct to handle requests and responses. Phoenix provides functions like `conn.params`, `conn.body_params`, and `conn.resp` for parsing incoming data and constructing responses.
- Testing:
Write tests for your API endpoints using ExUnit. Phoenix provides a testing framework that allows you to simulate HTTP requests and assert the expected responses.
- Authentication and Authorization:
Implement authentication and authorization mechanisms if your API requires them. You can use libraries like Guardian and Ueberauth for authentication.
- Documentation:
Consider generating API documentation using tools like Swagger or ExDoc to help consumers understand how to interact with your API.
- Deployment:
Deploy your Phoenix application to a hosting platform of your choice, such as Heroku, AWS, or a dedicated server.
By following these steps, you can create a RESTful API in Phoenix that handles HTTP requests, interacts with your data (if needed), and follows REST conventions for resource management. Phoenix’s structured architecture and helpful features make it a robust choice for building APIs in Elixir.
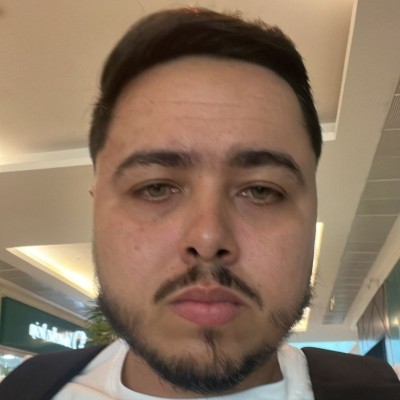
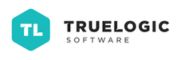