Building a RESTful JSON API with Elixir and Cowboy
In the ever-evolving landscape of web development, building robust and efficient APIs is paramount. With the rise of Elixir, a functional programming language known for its scalability and fault tolerance, developers are empowered to craft high-performance applications. In this guide, we’ll delve into the process of constructing a RESTful JSON API using Elixir and Cowboy, a blazingly fast HTTP server.
1. Why Elixir and Cowboy?
Elixir, built on the Erlang Virtual Machine (BEAM), offers concurrency and fault tolerance out of the box. Combined with Cowboy, a lightweight HTTP server, developers can achieve exceptional performance without sacrificing simplicity.
2. Setting Up Your Environment
Before diving into coding, ensure you have Elixir installed on your system. You can install it via Elixir’s official website – https://elixir-lang.org/install.html. Additionally, add Cowboy to your project dependencies by including it in your `mix.exs` file:
```elixir defp deps do [ {:cowboy, "~> 2.9"} ] end ```
Run `mix deps.get` to fetch the dependencies.
3. Creating Your Elixir Application
Let’s create a new Elixir application named `MyAPI`:
```bash mix new my_api ```
Navigate to the project directory:
```bash cd my_api ```
4. Implementing the API
Now, let’s define our API endpoints. We’ll start with a simple “Hello World” endpoint.
Create a module named `MyAPI.Router`:
```elixir defmodule MyAPI.Router do use Cowboy.Router dispatch(:_, [ {:_, %{"path" => ["hello"]}, :hello_handler} ] ) def hello_handler(conn, _opts) do send_resp(conn, 200, "Hello, World!") end end ```
Next, define the application supervision tree in `lib/my_api/application.ex`:
```elixir defmodule MyAPI.Application do use Application def start(_type, _args) do children = [ {Cowboy, MyAPI.Router, []} ] opts = [strategy: :one_for_one, name: MyAPI.Supervisor] Supervisor.start_link(children, opts) end end ```
5. Running Your API
Start your application with:
```bash mix run --no-halt ```
Visit `http://localhost:4000/hello` in your browser, and you should see “Hello, World!” displayed.
Conclusion
In this tutorial, we’ve explored the process of building a RESTful JSON API using Elixir and Cowboy. Leveraging Elixir’s concurrency model and Cowboy’s efficiency, we can construct highly performant APIs with ease.
Ready to dive deeper into Elixir and Cowboy? Check out the official documentation for [Elixir – https://hexdocs.pm/elixir and Cowboy – https://ninenines.eu/docs/en/cowboy/2.9/guide/.
Start building your next-generation APIs today with Elixir and Cowboy!
External References:
- Elixir – Official Documentation – https://hexdocs.pm/elixir
- Cowboy – Official Documentation – https://ninenines.eu/docs/en/cowboy/2.9/guide/
- Installing Elixir – https://elixir-lang.org/install.html
Table of Contents
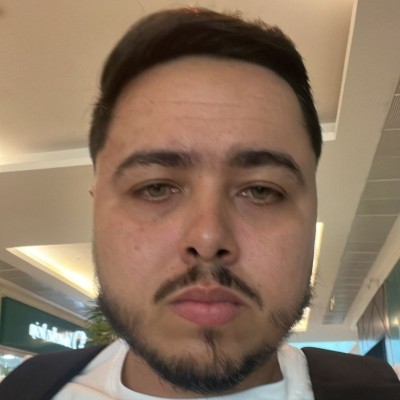
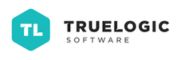