Introduction to Elixir Scripting: Automating Tasks
In today’s fast-paced world, automation has become the backbone of modern software development. As developers, we often find ourselves performing repetitive tasks, such as file processing, data manipulation, and system maintenance. These tasks can be time-consuming and error-prone, taking valuable time away from more critical aspects of our projects. Fortunately, Elixir scripting provides an elegant solution to automate these repetitive tasks efficiently.
In this blog post, we will introduce you to Elixir scripting and explore how it can empower you to automate various tasks seamlessly. We will delve into the syntax, key features, and real-world examples, showcasing the power and simplicity of Elixir for automation purposes.
1. Why Choose Elixir for Scripting?
Before diving into the technical details, it’s essential to understand why Elixir is a fantastic choice for scripting and task automation.
1.1. Elixir’s Concurrency Model
Elixir runs on the Erlang Virtual Machine (BEAM), inheriting Erlang’s renowned concurrency capabilities. It allows you to handle multiple tasks concurrently, making it ideal for scripting scenarios where parallel processing can significantly boost performance.
1.2. Fault-Tolerance and Resilience
Elixir follows the “let it crash” philosophy, where individual processes handle errors independently. When an error occurs, only the affected process crashes, leaving the rest of the system unharmed. This approach ensures that your scripts remain robust and continue running despite errors, ensuring high resilience.
1.3. Functional Programming Paradigm
Elixir embraces functional programming, which focuses on immutability and pure functions. This style of programming leads to more predictable and maintainable code, reducing side effects and facilitating easier testing.
1.4. Extensible and Scalable
With the ability to interact with existing Erlang libraries, Elixir provides a vast ecosystem of tools and functionalities. You can leverage these libraries to extend the capabilities of your scripts without reinventing the wheel.
2. Setting Up Elixir
Before we dive into the world of Elixir scripting, let’s ensure you have everything set up on your machine.
3. Installing Elixir
If you haven’t installed Elixir yet, head over to the official website (https://elixir-lang.org/) and follow the installation instructions for your operating system.
4. Verifying the Installation
Once installed, open a terminal or command prompt and run the following command to verify the installation:
elixir elixir --version
If everything is set up correctly, you should see the installed Elixir version printed on the screen.
5. Basics of Elixir Scripting
Now that we have Elixir up and running let’s start with the fundamentals of Elixir scripting.
5.1. Data Types and Variables
Elixir provides a rich set of data types, including integers, floats, booleans, atoms, strings, lists, tuples, and maps. Variables in Elixir are immutable, meaning their value cannot be changed after assignment.
elixir # Integer age = 30 # Float pi = 3.14159 # Boolean is_happy = true # Atom status = :ok # String greeting = "Hello, Elixir!" # List numbers = [1, 2, 3, 4, 5] # Tuple person = { "John", "Doe", 30 } # Map user = %{ name: "Alice", age: 25 }
5.2. Functions
Functions in Elixir are defined using the def keyword. The last expression in a function is considered the return value.
elixir defmodule Math do def add(a, b) do a + b end end IO.puts Math.add(5, 3) # Output: 8
5.3. Pattern Matching
Pattern matching is a powerful feature in Elixir that allows you to destructure data and bind variables based on patterns.
elixir defmodule User do def full_name({first_name, last_name}) do "#{first_name} #{last_name}" end end fullname = User.full_name({"John", "Doe"}) IO.puts fullname # Output: "John Doe"
5.4. Pipe Operator
The pipe operator (|>) is a convenient way to chain functions together, making the code more readable and expressive.
elixir filename |> File.read() |> String.upcase() |> IO.puts()
Elixir Scripting for Automation
Now that we have a grasp of the basics, let’s see how Elixir scripting can be used for automating real-world tasks.
1. File Manipulation
One common automation task is file manipulation. Elixir makes it simple to read, write, and process files.
elixir defmodule FileProcessor do def read_and_process_file(filename) do File.read(filename) |> String.split("\n") |> Enum.map(&String.trim(&1)) end end lines = FileProcessor.read_and_process_file("data.txt")
2. Data Transformation
Another common use case is data transformation. Elixir’s pattern matching and functional programming features make data manipulation a breeze.
elixir defmodule DataProcessor do def transform_data(%{name: name, age: age}) do %{ name: String.upcase(name), age: age + 1 } end end data = %{name: "Alice", age: 30} transformed_data = DataProcessor.transform_data(data)
3. Web Scraping
Elixir can also be used for web scraping tasks, leveraging libraries like HTTPoison and Floki.
elixir defmodule WebScraper do def scrape_website(url) do {:ok, response} = HTTPoison.get(url) {:ok, html} = Floki.parse(response.body) titles = Floki.find(html, "h2.title") Enum.map(titles, &Floki.text(&1)) end end titles = WebScraper.scrape_website("https://example.com")
Conclusion
Elixir scripting is a powerful and elegant solution for automating tasks and streamlining your workflow. Its concurrency model, fault-tolerance, and functional programming paradigm make it an excellent choice for automation purposes. By harnessing the capabilities of Elixir, you can save time, reduce errors, and focus on more critical aspects of your projects. So, start exploring the world of Elixir scripting and unleash the true potential of automation!
Table of Contents
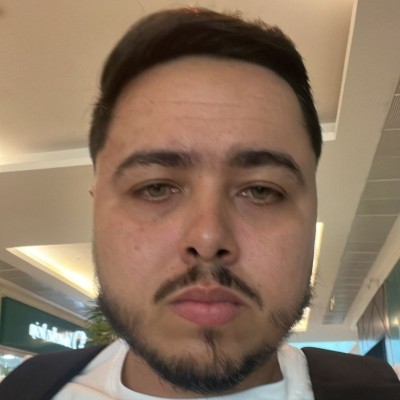
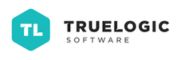