Elixir Streams: Laziness and Efficiency in Action
In the world of functional programming, Elixir has gained significant popularity for its elegant syntax, fault-tolerant architecture, and robust concurrency model. One of the key features that makes Elixir stand out is its powerful implementation of streams. Elixir Streams are not just another data structure; they are a fundamental concept that brings laziness and efficiency to data processing. In this blog, we’ll take a deep dive into Elixir Streams, understanding their underlying principles and exploring real-world use cases that showcase their prowess in action.
1. Understanding Elixir Streams
At its core, a stream in Elixir is an enumerable that produces values on demand. Unlike eager data collections like lists or maps, which compute all values immediately, streams utilize a lazy evaluation strategy. This means that values are computed only when they are needed, leading to optimized memory usage and improved performance for large datasets.
2. Laziness as a Virtue
Elixir Streams follow a lazy evaluation strategy, which means that computations are deferred until the moment they are actually needed. This approach brings several benefits:
2.1. Memory Efficiency
In scenarios where you’re dealing with massive datasets, eager loading of all data into memory can lead to memory exhaustion. Streams, on the other hand, only keep a small portion of the data in memory at a time, allowing you to process even large datasets with limited memory resources.
2.2. On-the-Fly Processing
Streams allow you to process data on-the-fly, enabling you to begin working with the results before all values are computed. This is particularly useful when dealing with infinite sequences or when you’re interested in the first few results.
3. Efficiency Unleashed
Elixir Streams not only embrace laziness but also offer efficiency through their composability and pipeline-based processing.
3.1. Pipeline Processing
Streams can be easily pipelined, enabling you to perform a sequence of transformations on the data. This approach encourages a functional programming style and improves code readability. Each transformation is applied one by one, and since streams are lazy, unnecessary computations are avoided.
elixir stream = Stream.iterate(1, &(&1 * 2)) |> Stream.filter(&(&1 <= 16)) |> Stream.map(&(&1 * 3)) Enum.to_list(stream) # Output: [3, 6, 12]
In this example, the stream is first generated with an infinite sequence of powers of 2, then filtered to retain values less than or equal to 16, and finally each value is tripled. Only the required transformations are performed as we consume the stream.
3.2. Infinite Sequences
Elixir Streams can represent infinite sequences with ease, thanks to their lazy nature. Consider generating an infinite stream of Fibonacci numbers:
elixir fibonacci = Stream.unfold({0, 1}, fn {a, b} -> {{a, b}, {b, a + b}} end) Enum.take(fibonacci, 10) # Output: [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
Here, the stream generates Fibonacci numbers indefinitely, and we use Enum.take/2 to limit the output to the first 10 numbers.
4. Real-World Use Cases
Let’s explore practical scenarios where Elixir Streams shine, demonstrating their laziness and efficiency in action.
4.1. Log Parsing
When dealing with log files, which can be enormous, using Elixir Streams to parse and extract specific information can drastically improve performance. Streams process the log file line by line, avoiding the need to load the entire file into memory. This can be particularly beneficial when working with log files that are too large to fit in memory.
elixir File.stream!("access.log") |> Stream.flat_map(&String.split(&1, " ")) |> Stream.filter(&String.starts_with?(&1, "GET")) |> Enum.count()
In this example, the stream reads an access log file, splits each line into words, filters out only the lines starting with “GET,” and finally counts the occurrences of such lines.
4.2. Data Transformation
Streams are excellent for transforming data while keeping memory usage in check. Imagine you have a large CSV file that needs to be transformed into another format. Streams can be used to read the CSV file line by line, transform the data, and write it to the new format, all without loading the entire dataset into memory.
elixir File.stream!("data.csv") |> CSV.decode(headers: true) |> Stream.map(&transform_function/1) |> CSV.encode(headers: true) |> Stream.into(File.stream!("transformed_data.csv"))
Here, the stream decodes the CSV file, applies a transformation function to each row, encodes the transformed data back to CSV format, and writes it to a new file.
Conclusion
Elixir Streams bring a unique blend of laziness and efficiency to data processing. Their lazy evaluation strategy ensures memory efficiency and enables on-the-fly processing, making them ideal for dealing with large datasets and infinite sequences. By embracing pipeline-based processing, Elixir Streams offer a clean and composable way to manipulate data. Real-world use cases like log parsing and data transformation showcase how Streams can significantly enhance performance while maintaining a small memory footprint.
Whether you’re dealing with big data, log files, or any scenario that requires optimized data processing, Elixir Streams prove to be a valuable tool in your functional programming toolkit. By leveraging their laziness and efficiency, you can create elegant and high-performance solutions that handle complex data processing tasks with ease. So, dive into the world of Elixir Streams, and unlock the power of lazy yet efficient data manipulation.
Table of Contents
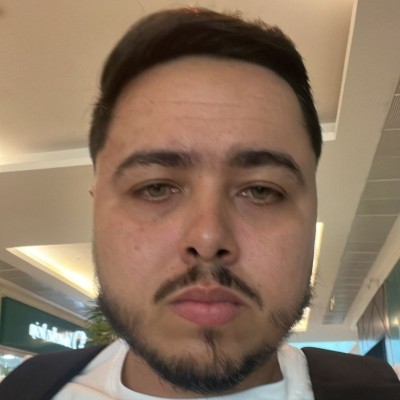
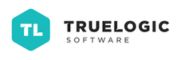