Introduction to Elixir’s Type Specifications and Dialyzer
In the world of software development, writing robust and reliable code is of paramount importance. As applications grow in complexity, ensuring that code behaves as intended becomes a more challenging task. Elixir, a dynamic and functional programming language built on the Erlang Virtual Machine (BEAM), offers a unique set of tools to enhance code quality and reliability. Two of these tools are Elixir’s Type Specifications and Dialyzer, which provide developers with the means to catch errors, document intentions, and optimize their programs.
Table of Contents
1. Understanding the Need for Type Specifications
Dynamic languages like Elixir provide flexibility and ease of development by allowing variables to change types at runtime. However, this flexibility can lead to unintended errors and runtime crashes. Enter Type Specifications: a feature that allows developers to specify the expected types of variables and function arguments.
2. Benefits of Type Specifications
Type Specifications offer several key benefits:
- Documentation: Type specifications serve as self-documentation for your code. They provide insights into the expected input and output types of functions, making it easier for developers to understand and use your code.
- Error Detection: By declaring expected types, you enable the Elixir compiler to catch type-related errors during development, preventing many runtime issues before they occur.
- Tooling Support: Type annotations enable development tools like Dialyzer to analyze your code more effectively and provide you with valuable insights into potential problems.
3. Annotating Types in Elixir
Elixir’s type specifications are annotations that developers add to their code to define the expected types of variables and function parameters. These annotations use the @type module attribute and follow the “dialyzer” syntax.
3.1. Basic Type Annotations
Let’s look at some basic type annotations:
elixir @type name :: String.t() @type age :: integer() def greet_person(name :: name(), age :: age()) do "Hello, #{name}! You are #{age} years old." end
In this example, we’ve annotated the name parameter as a String.t() and the age parameter as an integer(). These annotations not only provide clarity about the expected input types but also enable tools like Dialyzer to analyze the code for potential issues.
3.2. Custom Types and Union Types
Elixir allows you to create custom types using the @type attribute. You can also define union types that specify multiple possible types for a variable.
elixir @type email :: String.t() @type phone :: String.t() @type contact :: email() | phone() def send_message(contact :: contact(), message :: String.t()) do # Logic for sending messages end
In this example, we’ve defined a custom type contact() that represents either an email or a phone number. The send_message/2 function can accept either type as its first argument.
3.3. Specifying Function Return Types
Type specifications are not limited to function arguments; you can also annotate the return types of functions.
elixir @spec divide(number :: float(), divisor :: float()) :: float() def divide(number, divisor) when divisor != 0 do number / divisor end
In this case, the @spec attribute specifies that the divide/2 function takes two float arguments and returns a float. The additional guard clause ensures that division by zero is avoided.
4. Introducing Dialyzer
While type annotations provide valuable information to developers, they are even more powerful when used in conjunction with Dialyzer – a static analysis tool for Elixir and Erlang code. Dialyzer uses type specifications to analyze code and detect potential errors before runtime.
4.1. How Dialyzer Works
Dialyzer performs a type inference analysis based on the type annotations present in your code. It checks for type inconsistencies, unreachable code, and other potential issues that can lead to runtime errors. The tool doesn’t require running the code; it works solely on the codebase and annotations.
4.2. Running Dialyzer
To run Dialyzer on your Elixir project, you need to use the following command:
bash mix dialyzer
Dialyzer will then analyze your project’s codebase and provide a report detailing any issues it finds.
4.3. Interpreting Dialyzer’s Output
Dialyzer’s output can be a bit daunting at first, but understanding the key components will help you make sense of it:
- Success Typings: These are the types Dialyzer inferred without encountering any errors.
- Warnings: Dialyzer might emit warnings, indicating potential issues that require your attention. These could include incorrect or missing type specifications.
- Errors: If Dialyzer encounters serious inconsistencies or issues, it will report them as errors.
5. Leveraging Elixir’s Type System for Optimization
While type specifications and Dialyzer are invaluable for catching errors, they can also help optimize your codebase. Elixir’s type system allows you to write more efficient code by leveraging pattern matching and eliminating unnecessary runtime checks.
5.1. Pattern Matching and Type Guarantees
Consider the following example:
elixir @type point :: {float(), float()} def distance({x1, y1} :: point(), {x2, y2} :: point()) do :math.sqrt((x2 - x1)**2 + (y2 - y1)**2) end
In this case, the type specification for the point tuple guarantees that the distance/2 function will only receive tuples with two float elements. This knowledge allows the function to confidently access the tuple elements without additional runtime checks.
5.2. Optimizing Performance
Elixir’s pattern matching capabilities, combined with type specifications, can lead to performance improvements. The Erlang Virtual Machine (BEAM) can optimize pattern matching code more effectively, resulting in faster execution times.
Conclusion
Elixir’s Type Specifications and Dialyzer provide a robust set of tools for enhancing code quality, improving documentation, catching errors, and optimizing performance. By incorporating type annotations into your codebase and utilizing Dialyzer’s static analysis, you can create more reliable and efficient Elixir applications. Embracing these tools not only results in better software but also accelerates development cycles by catching issues early in the process. So, dive into the world of Elixir’s type system and Dialyzer, and take your coding skills to new heights.
Table of Contents
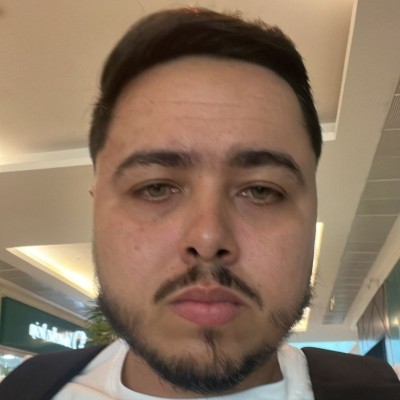
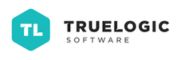