Ruby vs. Elixir: A Comprehensive Comparison for Your Project Selection
When it comes to selecting a programming language for a specific project, many developers face a tough choice. Each language comes with its unique set of features and advantages. Today, we’re delving into an interesting comparison between two dynamically typed languages, Elixir and Ruby. We’ll highlight why you might want to hire Elixir developers or opt for Ruby based on your specific project requirements. This discussion should give you a clearer perspective on which language could be a better fit for your particular needs.
1. Introduction to Ruby
Ruby, developed in the mid-90s by Yukihiro “Matz” Matsumoto, is a high-level, interpreted language. Its primary design philosophy centers around programmer happiness and productivity, placing a significant emphasis on simplicity and elegance. Ruby’s syntax is clean and easy to understand, even for beginners, which has earned it a reputation as an excellent language for newbies in the world of programming.
Ruby is primarily used for web development and comes with a robust and mature ecosystem. Rails, a web development framework built on Ruby, has been instrumental in popularizing the language. Twitter, GitHub, and Airbnb are a few examples of big-name companies that have used Ruby on Rails for their platforms.
```ruby # Ruby code example class HelloWorld def self.hello(name) "Hello, #{name}!" end end puts HelloWorld.hello("World") # Outputs: Hello, World! ```
2. Introduction to Elixir
Elixir, on the other hand, is a relatively young language, created by José Valim in 2011. Running on the Erlang Virtual Machine (BEAM), Elixir boasts excellent concurrent processing abilities and fault tolerance. These characteristics make it particularly well-suited for handling high-traffic, distributed systems, creating a solid case for why businesses might want to hire Elixir developers.
One of Elixir’s flagship frameworks, Phoenix, is a powerful tool for building highly scalable and maintainable web applications. Elixir’s robust performance is relied upon by companies like Discord, Pinterest, and Bleacher Report, who manage large volumes of traffic and requests. This demonstrates the growing demand to hire Elixir developers for creating resilient, high-performance systems.
```elixir # Elixir code example defmodule HelloWorld do def hello(name) do "Hello, #{name}!" end end IO.puts HelloWorld.hello("World") # Outputs: Hello, World! ```
3. Comparing Elixir and Ruby
Now that we have a brief understanding of both Ruby and Elixir, let’s delve deeper and examine various criteria to understand their differences and strengths.
3.1 Performance
If we talk about raw performance, Elixir has an edge due to its Erlang backbone. It allows Elixir to run lightweight processes concurrently, which gives it the ability to handle a large number of simultaneous connections. This makes Elixir a great choice for applications that require real-time processing, such as chat applications or live video streaming platforms.
On the other hand, Ruby’s performance might not be as robust as Elixir’s in handling high concurrency, but it is more than adequate for many web applications. Additionally, Ruby on Rails, with its rich ecosystem, provides many performance optimization options.
3.2 Syntax and Learning Curve
Ruby’s syntax is praised for its elegance and readability. It’s designed with programmer happiness in mind, making it friendly for beginners. The Object-Oriented nature of Ruby also makes it quite intuitive for people coming from other OO languages like Java or Python.
Elixir’s syntax has a functional programming paradigm, heavily influenced by Ruby and Erlang. This can seem a bit more complex for developers new to functional programming. However, if you’re already comfortable with Ruby’s syntax, picking up Elixir will not be too challenging, thanks to its syntactic similarity.
3.2 Libraries and Community
Ruby, with its long history and popularity, boasts a large and vibrant community. It has a wide variety of gems (libraries) that can help you build almost anything you can think of. This mature ecosystem is a big advantage for Ruby.
Elixir, though younger, has a rapidly growing community. The Hex package manager provides a growing number of libraries. While not as extensive as Ruby’s ecosystem, it is continually expanding.
3.3 Concurrency and Fault Tolerance
One of the most significant advantages of Elixir is its first-class support for concurrency and its incredible fault tolerance. This is due to its foundation on the Erlang Virtual Machine, which was originally designed for telecommunications systems that required high availability.
While Ruby can also handle concurrent programming with tools and gems, it isn’t as inherent or robust as in Elixir. This means that for applications where high concurrency and fault tolerance are crucial, Elixir may be the better choice.
3.4 Development Speed
One of the reasons Ruby, and particularly Ruby on Rails, gained popularity is its emphasis on Convention over Configuration and Don’t Repeat Yourself (DRY) principles. These philosophies allow developers to quickly prototype and build applications by reducing the decisions they need to make and the amount of code they have to write. Ruby’s ecosystem is packed with gems that can further speed up the development process.
```ruby # Example of DRY principle in Ruby on Rails class UsersController < ApplicationController def show @user = User.find(params[:id]) end def update @user = User.find(params[:id]) @user.update(user_params) redirect_to @user end private def user_params params.require(:user).permit(:username, :email) end end ```
Elixir, with its Phoenix Framework, also offers rapid development. Phoenix comes with built-in support for WebSockets (real-time communication), a feature often requiring additional setup in other frameworks. Elixir’s functional nature encourages small, reusable modules which can be beneficial for maintenance and readability.
```elixir # Example of Elixir Phoenix handling real-time communication defmodule MyAppWeb.UserChannel do use Phoenix.Channel def join("user:lobby", _message, socket) do {:ok, socket} end def handle_in("new:msg", payload, socket) do broadcast(socket, "new:msg", payload) {:noreply, socket} end end ```
3.5 Scalability
Scalability often becomes a major concern as applications grow and begin to serve larger user bases. Ruby’s performance under high traffic scenarios has been a topic of discussion over the years. While it’s true that Ruby may not be the best fit for applications that require high concurrency, it’s worth noting that many large-scale applications, like GitHub and Shopify, have managed to scale Ruby effectively with the right architecture and optimizations.
Elixir, being built on the Erlang VM, is designed to support distributed and concurrent systems out of the box. This makes Elixir an excellent choice for applications that need to handle many simultaneous users or requests, or those that require real-time data processing. This scalability is one of the reasons why companies with high concurrency requirements, like Discord and Bleacher Report, have chosen Elixir for their backends.
3.6 Job Market
If we look at the job market, as of my knowledge cutoff in 2021, Ruby has more job opportunities, largely due to its history and widespread adoption. Ruby on Rails remains a popular choice for web development, and there’s a steady demand for Ruby developers.
Elixir, while growing in popularity, still has a smaller market. That said, the high performance and scalability of Elixir make it a valuable skill, particularly for jobs requiring real-time functionality or high concurrency handling. As Elixir adoption grows, it’s likely we’ll see an increase in demand for Elixir developers.
Remember, the right language for you will depend on various factors, including the nature of your project, the skills you want to develop, and the type of jobs you’re aiming for. Both Ruby and Elixir have a lot to offer, and understanding their strengths and weaknesses will help you make an informed choice.
So, Which One Should You Choose?
The choice between Elixir and Ruby boils down to your project’s specific requirements, your team’s skill set, and your personal preference.
Choose Ruby if:
– You are a beginner in programming or coming from an OO language.
– You want to leverage a mature ecosystem with a rich set of libraries.
– You are building a standard web application where Ruby on Rails can speed up development.
Choose Elixir if:
– You need to build a high-performance, real-time system.
– You are dealing with a high-traffic, distributed system.
– Your system requires a high degree of fault tolerance.
Conclusion
In conclusion, both Ruby and Elixir are excellent languages, each with unique strengths. Ruby offers elegance, simplicity, and a mature ecosystem, perfect for projects that prioritize rapid development and ease of use. On the other hand, Elixir brings high performance, real-time processing, and fault tolerance to the table. This makes it a sought-after choice for high-traffic, distributed systems, and underscores why many businesses are looking to hire Elixir developers. Understanding these traits and aligning them with your specific needs will help guide you in making the right choice, whether that entails hiring Ruby or Elixir developers.
Table of Contents
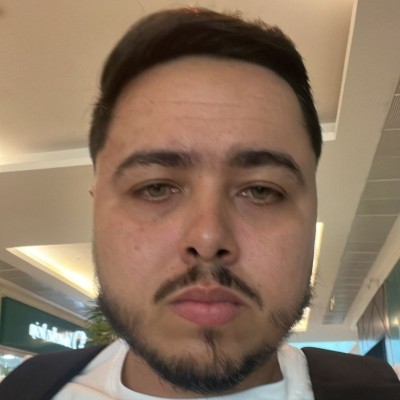
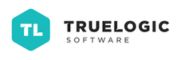