Functional Web Development with Elixir and LiveView
In the ever-evolving landscape of web development, developers are constantly seeking new ways to create more efficient, robust, and interactive applications. Traditional web development stacks often involve a complex mix of languages, frameworks, and libraries that can sometimes lead to cumbersome codebases and slow development cycles. Enter Elixir and LiveView – a powerful combination that offers a fresh approach to building dynamic web applications.
1. Understanding Elixir and LiveView
1.1 The Elixir Language
At the core of this duo lies Elixir, a functional programming language designed for building scalable and maintainable applications. Elixir runs on the Erlang Virtual Machine (BEAM), known for its exceptional concurrency and fault-tolerance capabilities. This combination makes Elixir well-suited for building applications that demand high performance and real-time interactivity.
1.1.1 Benefits of Elixir
- Concurrency: Elixir’s lightweight processes, also known as actors, enable true concurrency. Each process runs independently, communicating through message passing, which eliminates many of the complexities associated with traditional thread-based concurrency.
- Fault Tolerance: The BEAM VM, on which Elixir runs, is renowned for its fault tolerance. Applications can be designed to gracefully recover from failures, ensuring high availability and reliability.
- Scalability: Elixir’s actor-based concurrency model makes it easier to scale applications horizontally by adding more servers to the cluster. This allows applications to handle increasing workloads without sacrificing performance.
1.2 Introducing Phoenix LiveView
While Elixir provides a solid foundation for backend development, it’s Phoenix LiveView that takes the spotlight when it comes to frontend interactivity. LiveView is a game-changing library that brings real-time updates and interactivity to web applications without the need for writing JavaScript.
1.2.1 How LiveView Works
LiveView leverages Elixir’s concurrency model to create lightweight processes for each user’s session. These processes maintain the application’s state on the server and handle user interactions. When a user performs an action, such as clicking a button, LiveView captures the event, processes it on the server, and sends the updated HTML back to the client. The client then seamlessly updates the UI to reflect the changes.
1.2.2 The Advantages of LiveView
- Reduced Complexity: With LiveView, developers can build rich, interactive web applications using familiar tools like Elixir and HTML. This eliminates the need to manage complex frontend frameworks and libraries, reducing the overall complexity of the tech stack.
- Enhanced Productivity: LiveView’s declarative syntax allows developers to express dynamic behavior directly in the template. This reduces the back-and-forth between frontend and backend developers, leading to faster development cycles.
- SEO-Friendly: One common challenge with single-page applications (SPAs) is their impact on SEO. Since LiveView generates HTML on the server and sends it to the client, search engines can easily crawl and index the content, improving SEO.
2. Building a Dynamic Web Application with Elixir and LiveView
To better understand how Elixir and LiveView work together, let’s walk through the process of building a simple yet dynamic to-do list application.
2.1 Setting Up the Project
First, make sure you have Elixir and Phoenix installed on your system. You can create a new Phoenix project using the following command:
bash mix phx.new todo_list --no-webpack --no-html
Here, we’re skipping the default frontend setup (webpack and HTML) since LiveView will handle the frontend.
2.2 Creating the To-Do List LiveView
Let’s start by generating a LiveView for our to-do list:
bash mix phx.gen.live Todo Task tasks description:string done:boolean
This command generates a LiveView named TaskLive along with the necessary database migration and schema.
2.3 Designing the User Interface
Open the task_live.ex file in the lib/todo_list_web/live directory. Here’s an example of how the LiveView module could look:
elixir defmodule TodoListWeb.TaskLive do use Phoenix.LiveView def mount(_params, _session, socket) do {:ok, assign(socket, tasks: TodoList.Tasks.list_tasks())} end def handle_event("toggle_done", %{"id" => id}, socket) do task = TodoList.Tasks.get_task!(id) updated_task = TodoList.Tasks.toggle_done(task) {:noreply, assign(socket, tasks: TodoList.Tasks.list_tasks())} end end
In this module, we’re defining the initial mount function to fetch and assign tasks to the socket. We’re also defining an event handler to toggle the status of a task when the “toggle_done” event is triggered.
2.4 Building the Template
Next, open the task_live.html.leex file in the same directory. Here’s an example of how the LiveView template could look:
html <div> <ul> <%= for task <- @tasks do %> <li> <label class="<%= if task.done, do: "completed" %>"> <input type="checkbox" phx-click="toggle_done" phx-value-id="<%= task.id %>" <%= if task.done, do: "checked=\"checked\"" %> /> <%= task.description %> </label> </li> <% end %> </ul> </div>
This template iterates through the list of tasks and renders each task as a list item. The checkbox’s state is bound to the task’s “done” status, and clicking it triggers the “toggle_done” event.
2.5 Starting the LiveView
To start the LiveView, add the following snippet to the root.html.leex file in the lib/todo_list_web/templates/layout directory:
html <%= live_render(@conn, TodoListWeb.TaskLive) %>
This snippet embeds the LiveView into the layout, making it available on every page.
3. Running the Application
Start your Phoenix server with:
bash mix phx.server
Visit http://localhost:4000 in your browser, and you’ll find your dynamic to-do list ready to use!
Conclusion
Elixir and LiveView offer a refreshing approach to web development by combining the power of functional programming with real-time interactivity. With Elixir’s concurrency and fault tolerance, and LiveView’s seamless frontend updates, developers can create performant and engaging web applications without the complexity of managing multiple technologies. This duo proves that functional programming isn’t just for the backend – it’s a game-changer for the frontend too. So why not give Elixir and LiveView a try and experience the future of functional web development firsthand? Your users and your development team will thank you!
Table of Contents
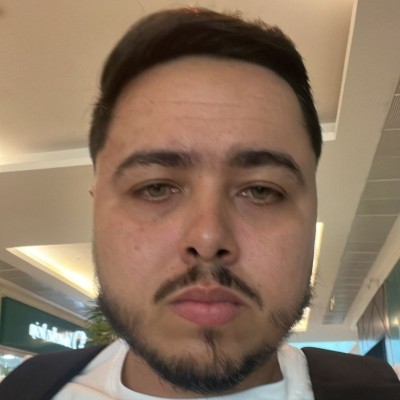
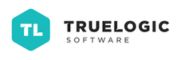