How to write unit tests in Elixir?
Writing unit tests in Elixir using the ExUnit testing framework is a crucial practice for ensuring the correctness and reliability of your code. Unit tests focus on testing individual functions or modules in isolation, verifying that they behave as expected. Here’s a step-by-step guide on how to write unit tests in Elixir:
- Create a Test Module: Start by creating a test module that mirrors the module you want to test. For example, if you have a module named `MyModule`, create a corresponding test module named `MyModuleTest`. Ensure that you add `use ExUnit.Case` at the beginning of your test module to import ExUnit’s testing capabilities.
- Define Test Cases: Within your test module, define test cases as functions with names ending in `test`. Each test case should focus on a specific aspect of the module’s behavior. For instance, if you have a function `MyModule.my_function/2`, you might write a test case like this:
```elixir test "should return the sum of two numbers" do result = MyModule.my_function(2, 3) assert result == 5 end ```
- Use Assertions: ExUnit provides a range of built-in assertions to check the correctness of your code. Common assertions include `assert`, `refute`, `assert_equal`, `assert_match`, and more. Choose the assertion that best suits the condition you want to verify in your test case.
- Arrange, Act, Assert (AAA): Follow the AAA pattern in your tests. Arrange: Set up any necessary preconditions. Act: Invoke the function or code under test. Assert: Verify the expected outcomes or states.
- Run Tests: You can run your tests using the `mix test` command in your project’s root directory. This command will execute all the tests in your project and provide detailed feedback on test results.
- Interpret Test Results: After running tests, you’ll receive feedback on which tests passed and which failed. Carefully review any failing tests to identify the issues in your code.
- Refactor and Repeat: If tests uncover issues or bugs in your code, make the necessary corrections, and rerun the tests. The goal is to iterate until all tests pass, indicating that your code behaves as expected.
- Edge Cases and Error Handling: Ensure your tests cover not only typical cases but also edge cases and error scenarios. This helps identify potential issues that might occur in real-world usage.
- Mocks and Stubs: When necessary, use third-party libraries like `Mox` for mocking and stubbing external dependencies or behaviors that are hard to replicate in unit tests.
- Test Coverage: Keep an eye on test coverage metrics to ensure that your tests adequately cover your codebase. Aim for comprehensive coverage to minimize the risk of undetected issues.
By following these steps, you can effectively write unit tests for your Elixir codebase, promoting code quality, maintainability, and confidence in your software’s behavior. Remember that unit tests are just one part of a comprehensive testing strategy that also includes integration tests and end-to-end tests for complete coverage.
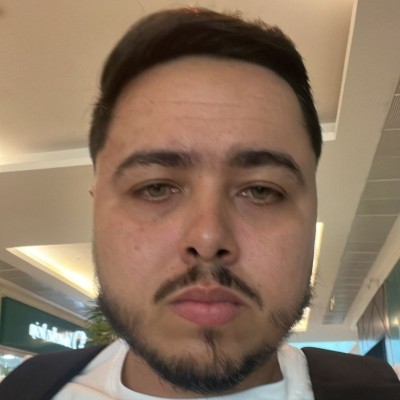
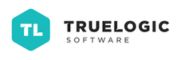