Express and Angular: Creating Dynamic Web Applications
Express and Angular are two powerful frameworks that, when combined, can be used to create dynamic and scalable web applications. Express, a minimalist web framework for Node.js, provides a robust back-end solution, while Angular, a popular front-end framework by Google, allows developers to build responsive and interactive user interfaces. This article explores how to integrate Express and Angular effectively and provides practical examples to demonstrate the creation of dynamic web applications.
Understanding the Express Framework:
Express is a flexible Node.js web application framework that provides a set of robust features for web and mobile applications. It simplifies the process of building a server and handling HTTP requests, making it an ideal choice for back-end development.
Introduction to Angular:
Angular is a comprehensive front-end framework for building single-page applications (SPAs). It offers features like two-way data binding, dependency injection, and modularity, which streamline the development of complex and interactive user interfaces.
Integrating Express and Angular:
Combining Express with Angular allows developers to create full-stack applications where the back-end serves the data and the front-end provides a responsive user interface. Below are key steps and examples to demonstrate the integration of Express and Angular.
1. Setting Up the Express Server
The first step in building a dynamic web application is to set up the back-end server using Express. Express handles the routing and API endpoints that Angular will consume.
Example: Creating a Basic Express Server
```javascript const express = require('express'); const app = express(); const port = 3000; // Serve static files from the Angular dist directory app.use(express.static('dist/angular-app')); // API endpoint app.get('/api/data', (req, res) => { res.json({ message: 'Hello from Express!' }); }); // Catch-all route to serve Angular's index.html app.get('*', (req, res) => { res.sendFile(__dirname + '/dist/angular-app/index.html'); }); app.listen(port, () => { console.log(`Server running at http://localhost:${port}`); }); ```
2. Creating the Angular Front-End
Angular will be responsible for the front-end of the application, handling the user interface and making HTTP requests to the Express back-end.
Example: Setting Up an Angular Service to Consume the Express API
```typescript import { Injectable } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Observable } from 'rxjs'; @Injectable({ providedIn: 'root' }) export class DataService { private apiUrl = '/api/data'; constructor(private http: HttpClient) {} getData(): Observable<any> { return this.http.get<any>(this.apiUrl); } } ```
Example: Displaying Data in an Angular Component
```typescript import { Component, OnInit } from '@angular/core'; import { DataService } from './data.service'; @Component({ selector: 'app-root', template: ` <div> <h1>{{ data.message }}</h1> </div> ` }) export class AppComponent implements OnInit { data: any; constructor(private dataService: DataService) {} ngOnInit() { this.dataService.getData().subscribe((response) => { this.data = response; }); } } ```
3. Handling Routing and Navigation
Angular’s powerful routing module allows you to create a single-page application where users can navigate through different views without reloading the page.
Example: Setting Up Angular Routing
```typescript import { NgModule } from '@angular/core'; import { RouterModule, Routes } from '@angular/router'; import { HomeComponent } from './home/home.component'; import { AboutComponent } from './about/about.component'; const routes: Routes = [ { path: '', component: HomeComponent }, { path: 'about', component: AboutComponent }, ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule] }) export class AppRoutingModule { } ```
4. Deploying the Application
Once development is complete, you can deploy your Express and Angular application to a production environment. Tools like Heroku, AWS, or Azure can be used to host the application.
Example: Building and Deploying the Angular App
```bash ng build --prod ```
After building the Angular app, it can be served through the Express server as shown in the initial setup.
Conclusion
Combining Express and Angular allows developers to build powerful, full-stack web applications. Express handles the server-side logic, while Angular provides a responsive and dynamic front-end. By leveraging these two frameworks together, you can create scalable, efficient, and user-friendly web applications.
Further Reading:
Table of Contents
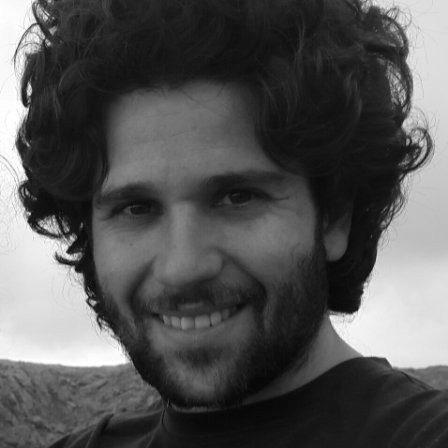
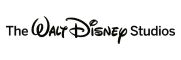