Express and AWS: Deploying Your Application on the Cloud
Express.js, a minimal and flexible Node.js web application framework, is widely used for building web applications and APIs. AWS (Amazon Web Services) is one of the leading cloud service providers, offering a robust and scalable environment for deploying applications. This guide will walk you through the process of deploying an Express.js application on AWS, including setting up an EC2 instance, configuring security groups, and deploying your application.
Understanding Express.js and AWS
Express.js is known for its simplicity and performance, making it an ideal choice for building web applications. AWS, on the other hand, offers a variety of services that provide the infrastructure needed to host, scale, and manage your applications effectively. Deploying an Express.js application on AWS combines the best of both worlds—allowing you to build and deploy applications with high availability and scalability.
Deploying an Express.js Application on AWS
Deploying an Express.js application on AWS involves several steps, from setting up the server environment to configuring your application to run smoothly on the cloud.
1. Setting Up an EC2 Instance
The first step in deploying your application is to set up an EC2 instance, which acts as a virtual server in the cloud.
Step-by-Step Guide:
– Launch an EC2 Instance:
Log in to your AWS Management Console, navigate to the EC2 service, and click on “Launch Instance.” Choose an Amazon Machine Image (AMI), such as Amazon Linux 2 or Ubuntu, select an instance type (e.g., t2.micro for free tier), and configure instance details.
– Configure Security Groups:
Create a security group with the necessary inbound rules. For a typical Express.js application, you’ll need to allow HTTP (port 80) and SSH (port 22) access.
– Connect to Your Instance:
Use SSH to connect to your EC2 instance using a terminal or a tool like PuTTY.
```bash ssh -i "your-key-pair.pem" ec2-user@your-ec2-public-dns ```
2. Installing Node.js and Express
Once your EC2 instance is set up, the next step is to install Node.js and Express.
Step-by-Step Guide:
– Update Your Server:
Update your package lists and install Node.js.
```bash sudo yum update -y sudo yum install -y nodejs npm ```
– Install Express:
Use npm to install Express globally on your EC2 instance.
```bash sudo npm install -g express-generator ```
– Create Your Express App:
Generate a new Express application.
```bash express myapp cd myapp npm install ```
3. Configuring Your Express App for Production
To ensure your Express application runs smoothly in a production environment, some configuration is needed.
Step-by-Step Guide:
– Set Environment Variables:
Store sensitive information such as database credentials in environment variables.
```bash export NODE_ENV=production export PORT=80 ```
– Install and Configure Process Manager (PM2):
PM2 is a process manager that keeps your Node.js applications running. It’s useful for ensuring that your application restarts automatically if it crashes.
```bash sudo npm install -g pm2 pm2 start ./bin/www pm2 startup ```
4. Setting Up a Reverse Proxy with NGINX
NGINX can be used as a reverse proxy to forward incoming requests to your Express.js application.
Step-by-Step Guide:
– Install NGINX:
Install NGINX on your EC2 instance.
```bash sudo yum install -y nginx ```
– Configure NGINX:
Edit the NGINX configuration file to set up the reverse proxy.
```bash sudo vi /etc/nginx/nginx.conf ```
Add the following configuration to your server block:
```nginx server { listen 80; server_name your-domain.com; location / { proxy_pass http://localhost:80; proxy_http_version 1.1; proxy_set_header Upgrade $http_upgrade; proxy_set_header Connection 'upgrade'; proxy_set_header Host $host; proxy_cache_bypass $http_upgrade; } } ```
– Start NGINX:
Enable and start NGINX.
```bash sudo systemctl enable nginx sudo systemctl start nginx ```
5. Deploying Your Express App
Now that your environment is set up, you can deploy your Express.js application.
Step-by-Step Guide:
– Transfer Your Application:
Use SCP or Git to transfer your application files to the EC2 instance.
```bash scp -i "your-key-pair.pem" -r /path/to/your/app ec2-user@your-ec2-public-dns:/home/ec2-user/myapp ```
– Install Dependencies and Start the Application:
Navigate to your application directory on the server, install dependencies, and start your application with PM2.
```bash cd myapp npm install pm2 start ./bin/www ```
6. Monitoring and Scaling Your Application
AWS offers services like CloudWatch for monitoring and Auto Scaling for automatically scaling your application based on demand.
Step-by-Step Guide:
– Set Up CloudWatch:
Configure CloudWatch to monitor your application’s performance and set up alarms for critical metrics.
– Enable Auto Scaling:
Use AWS Auto Scaling to automatically adjust the number of EC2 instances in response to traffic changes.
Conclusion
Deploying an Express.js application on AWS provides a powerful and scalable solution for hosting your web applications. By following the steps outlined in this guide, you can set up a robust environment for your application, ensuring high availability and performance.
Further Reading:
Table of Contents
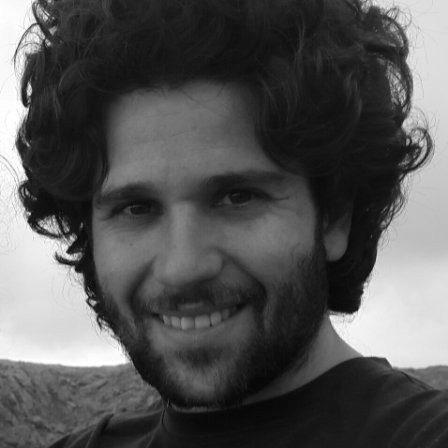
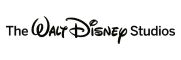