Express.js Best Practices: Writing Clean and Maintainable Code
Express.js is a popular framework for building web applications in Node.js. To ensure your Express.js applications are clean, maintainable, and scalable, following best practices is crucial. This blog explores key strategies for writing effective code in Express.js and provides practical examples to guide you.
Understanding Express.js
Express.js is a minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications. It simplifies the process of handling HTTP requests, routing, and middleware management, making it a go-to choice for many developers.
Best Practices for Writing Clean and Maintainable Code in Express.js
1. Organize Your Project Structure
A well-organized project structure improves code readability and maintainability. Follow a consistent pattern for organizing routes, controllers, and middleware.
Example: Project Structure
``` /myapp ??? /controllers ? ??? userController.js ? ??? postController.js ??? /routes ? ??? userRoutes.js ? ??? postRoutes.js ??? /middleware ? ??? authMiddleware.js ??? /models ? ??? userModel.js ??? app.js ??? package.json ```
2. Use Middleware Wisely
Middleware functions in Express.js are essential for handling requests, responses, and errors. Implement middleware to manage authentication, logging, and error handling efficiently.
Example: Authentication Middleware
```javascript // middleware/authMiddleware.js const jwt = require('jsonwebtoken'); const authenticate = (req, res, next) => { const token = req.headers['authorization']; if (!token) return res.status(403).json({ message: 'No token provided' }); jwt.verify(token, 'your-secret-key', (err, decoded) => { if (err) return res.status(500).json({ message: 'Failed to authenticate token' }); req.userId = decoded.id; next(); }); }; module.exports = authenticate; ```
3. Implement Modular Routing
Use modular routing to keep route definitions organized and manageable. This approach separates route logic into individual files, making it easier to maintain and scale.
Example: Modular Routing
```javascript // routes/userRoutes.js const express = require('express'); const router = express.Router(); const userController = require('../controllers/userController'); router.get('/users', userController.getAllUsers); router.post('/users', userController.createUser); module.exports = router; ``` ```javascript // app.js const express = require('express'); const app = express(); const userRoutes = require('./routes/userRoutes'); app.use('/api', userRoutes); ```
4. Handle Errors Gracefully
Centralize error handling to ensure consistent error responses and avoid repetitive code. Use a global error handler to catch and respond to errors.
Example: Global Error Handler
```javascript // app.js const express = require('express'); const app = express(); // Define routes and middleware here // Global Error Handler app.use((err, req, res, next) => { console.error(err.stack); res.status(500).json({ message: 'Something went wrong' }); }); app.listen(3000, () => console.log('Server running on port 3000')); ```
5. Follow Coding Conventions
Adhere to coding conventions and style guides to ensure consistency and readability across your codebase. Use tools like ESLint for JavaScript linting and Prettier for code formatting.
Example: ESLint Configuration
```json // .eslintrc.json { "env": { "node": true, "es6": true }, "extends": "eslint:recommended", "parserOptions": { "ecmaVersion": 2020 }, "rules": { "no-unused-vars": "warn", "no-console": "off" } } ```
6. Optimize Performance
Ensure your Express.js application performs efficiently by implementing caching, minimizing response times, and using asynchronous operations where appropriate.
Example: Caching with Redis
```javascript // middleware/cacheMiddleware.js const redis = require('redis'); const client = redis.createClient(); const cache = (req, res, next) => { const key = req.originalUrl || req.url; client.get(key, (err, data) => { if (err) throw err; if (data) { return res.send(JSON.parse(data)); } else { res.sendResponse = res.send; res.send = (body) => { client.setex(key, 3600, JSON.stringify(body)); res.sendResponse(body); }; next(); } }); }; module.exports = cache; ```
7. Write Unit Tests
Writing unit tests for your routes, controllers, and middleware ensures your application behaves as expected and helps prevent regressions.
Example: Unit Test with Mocha and Chai
```javascript // test/userController.test.js const chai = require('chai'); const chaiHttp = require('chai-http'); const app = require('../app'); chai.use(chaiHttp); const { expect } = chai; describe('GET /api/users', () => { it('should return all users', (done) => { chai.request(app) .get('/api/users') .end((err, res) => { expect(res).to.have.status(200); expect(res.body).to.be.an('array'); done(); }); }); }); ```
Conclusion
Applying best practices in Express.js development helps you write clean, maintainable, and scalable code. By organizing your project structure, using middleware effectively, implementing modular routing, and adhering to coding conventions, you can create robust web applications that are easier to maintain and extend. Emphasize performance optimization and testing to ensure your application performs well and remains reliable.
Further Reading:
Table of Contents
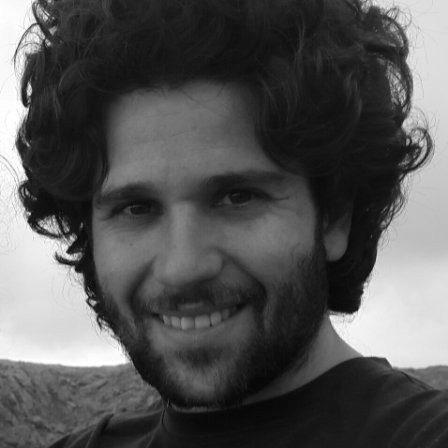
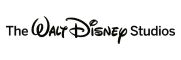