Building Robust APIs with Express: Best Practices and Tips
Building robust and efficient APIs is essential for creating successful web applications. Express, a popular Node.js framework, provides a flexible and powerful environment for API development. In this blog post, we will explore the best practices and tips for building robust APIs using Express. By following these guidelines, you can ensure your APIs are scalable, maintainable, and performant. Let’s dive in!
Setting Up Express: Installation and Basic Configuration
To get started with Express, you’ll need to install it as a dependency in your Node.js project. Open your terminal and run the following command:
Shell npm install express
Once Express is installed, you can create a basic server by importing the module, setting up routes, and starting the server. Here’s an example:
javascript const express = require('express'); const app = express(); // Define routes app.get('/', (req, res) => { res.send('Hello, World!'); }); // Start the server app.listen(3000, () => { console.log('Server running on port 3000'); });
Structuring Your API Code
Organizing your code is crucial for maintainability. Consider using a modular approach by separating routes, controllers, and middleware into separate files. Here’s an example structure:
plaintext - src - routes - userRoutes.js - controllers - userController.js - middleware - authMiddleware.js - app.js
Handling Routes and Middleware
Express provides a clean and straightforward way to handle routes and middleware. Use the express.Router() to create modular route handlers. Here’s an example:
javascript // userRoutes.js const express = require('express'); const router = express.Router(); const userController = require('../controllers/userController'); // Define routes router.get('/', userController.getAllUsers); router.post('/', userController.createUser); module.exports = router; javascript // app.js const express = require('express'); const app = express(); const userRoutes = require('./routes/userRoutes'); // Middleware app.use(express.json()); // Routes app.use('/users', userRoutes); // Start the server app.listen(3000, () => { console.log('Server running on port 3000'); });
Input Validation and Data Sanitization
Validating and sanitizing user input is crucial for security and data integrity. Express provides various middleware libraries, such as express-validator and sanitize, to handle these tasks. Here’s an example:
javascript const { body, validationResult } = require('express-validator'); // Validate and sanitize input router.post('/', [ body('name').trim().isLength({ min: 3 }), body('email').isEmail().normalizeEmail(), ], (req, res) => { const errors = validationResult(req); if (!errors.isEmpty()) { return res.status(400).json({ errors: errors.array() }); } // Process the request });
Error Handling and Logging
Proper error handling and logging improve the stability and debugging experience of your API. Use Express middleware to handle errors and log them for future reference. Here’s an example:
javascript // Error handling middleware app.use((err, req, res, next) => { console.error(err.stack); res.status(500).send('Internal Server Error'); }); // Logging middleware app.use((req, res, next) => { console.log(`${req.method} ${req.url}`); next(); });
Authentication and Authorization
Securing your API is crucial to protect sensitive data and prevent unauthorized access. Use middleware libraries like passport and jsonwebtoken for authentication and authorization. Here’s an example:
javascript // Authenticate middleware const authenticate = (req, res, next) => { // Check if the user is authenticated if (req.isAuthenticated()) { return next(); } // Unauthorized access res.status(401).send('Unauthorized'); }; // Protected route router.get('/profile', authenticate, (req, res) => { // Access user profile });
Performance Optimization
Optimizing your API’s performance ensures a smooth and efficient user experience. Consider implementing caching, compression, and request throttling techniques. Here’s an example using the compression middleware:
javascript const compression = require('compression'); // Enable compression app.use(compression());
Testing and Documentation
Testing your API helps ensure its correctness and reliability. Tools like Mocha and Chai can be used for unit and integration testing. Additionally, documenting your API using tools like Swagger or apidoc facilitates its understanding and usage for other developers.
Deployment and Continuous Integration
When deploying your Express API, consider using containerization with tools like Docker and container orchestration platforms like Kubernetes. Utilize continuous integration and deployment (CI/CD) pipelines to automate the build, test, and deployment processes for increased efficiency.
Conclusion
Building robust APIs with Express requires following best practices and adopting essential tips to ensure scalability, maintainability, and performance. By implementing proper code structuring, handling routes and middleware, input validation, error handling, authentication, performance optimization, testing, and documentation, you can create APIs that meet industry standards. Happy coding and enjoy building robust Express APIs!
Table of Contents
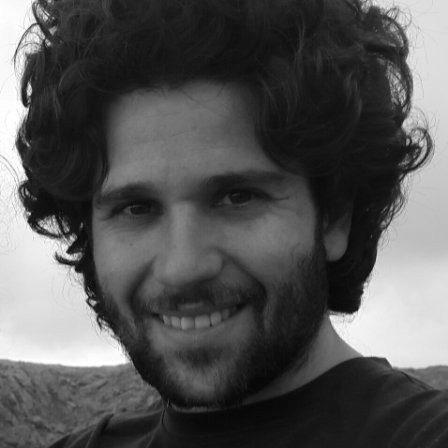
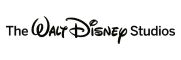