Building a Chat Application with Express and Socket.IO
Real-time communication has become a key feature in modern web applications, enabling instant interaction between users. Express and Socket.IO, both powered by Node.js, offer a powerful combination for building real-time chat applications. In this blog, we’ll explore how to create a chat application using Express for the backend and Socket.IO for WebSocket-based communication.
Understanding Express and Socket.IO
Express is a minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications. It simplifies the process of building APIs, managing routes, and handling middleware.
Socket.IO is a library that enables real-time, bidirectional, and event-based communication between clients and servers. It uses WebSockets under the hood but also provides fallbacks for older browsers that do not support WebSockets.
Setting Up the Project
Before diving into the code, let’s set up our project environment. You’ll need Node.js installed on your machine.
1. Initialize the Project:
Start by creating a new directory for your chat application and initialize it with `npm`:
```bash mkdir chat-app cd chat-app npm init -y ```
2. Install Dependencies:
Next, install the required packages:
```bash npm install express socket.io ```
Creating the Server with Express
Now, let’s create the Express server that will handle HTTP requests and serve the chat application.
1. Setup the Express Server:
Create a file named `index.js` and set up a basic Express server:
```javascript const express = require('express'); const http = require('http'); const socketIO = require('socket.io'); const app = express(); const server = http.createServer(app); const io = socketIO(server); const PORT = process.env.PORT || 3000; app.get('/', (req, res) => { res.sendFile(__dirname + '/index.html'); }); server.listen(PORT, () => { console.log(`Server running on port ${PORT}`); }); ```
2. Create a Basic HTML Client:
Create an `index.html` file that will serve as the frontend for your chat application:
```html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Chat Application</title> </head> <body> <h1>Chat Room</h1> <div id="messages"></div> <input id="messageInput" autocomplete="off" /> <button onclick="sendMessage()">Send</button> <script src="/socket.io/socket.io.js"></script> <script> const socket = io(); socket.on('message', function(msg){ const messages = document.getElementById('messages'); const messageElement = document.createElement('div'); messageElement.textContent = msg; messages.appendChild(messageElement); }); function sendMessage() { const input = document.getElementById('messageInput'); socket.emit('message', input.value); input.value = ''; } </script> </body> </html> ```
Integrating Socket.IO for Real-Time Communication
With the basic server and client setup, let’s integrate Socket.IO to enable real-time chat functionality.
1. Handling Socket Connections:
In the `index.js` file, add the following code to handle socket connections:
```javascript io.on('connection', (socket) => { console.log('A user connected'); socket.on('message', (msg) => { io.emit('message', msg); }); socket.on('disconnect', () => { console.log('A user disconnected'); }); }); ```
This code listens for incoming socket connections and messages. When a message is received, it broadcasts the message to all connected clients.
Testing the Chat Application
To test the chat application, start the server:
```bash node index.js ```
Open your browser and navigate to `http://localhost:3000`. You should see the chat interface. Open multiple tabs or browsers to simulate multiple users, and you’ll see the real-time chat in action.
Enhancing the Chat Application
With the basic chat application working, you can enhance it by adding features like:
- User Authentication:
Implement user authentication to manage user sessions and display usernames alongside messages.
- Private Messaging:
Extend the application to support private messaging between users.
- Message Persistence:
Store chat history in a database like MongoDB, enabling users to view past conversations.
- Styling the Interface:
Use CSS frameworks like Bootstrap or Tailwind CSS to improve the look and feel of the chat interface.
Conclusion
Building a chat application with Express and Socket.IO is a great way to learn real-time communication in web development. By leveraging Node.js, you can create scalable and efficient chat systems that enhance user interaction. The code examples provided here should give you a solid foundation to start building your own chat applications.
Further Reading:
Table of Contents
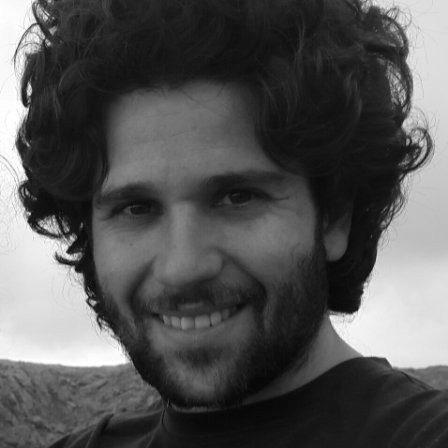
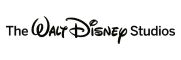